#include "main.h" #include "stm32g0xx_hal.h" // 定义LED引脚 #define LED_PIN GPIO_PIN_5 #define LED_PORT GPIOA // 定义WS2812数据帧格式 #define WS2812_LOW_TIME 30 // 单位:纳秒 #define WS2812_HIGH_TIME 70 // 单位:纳秒 // 设置RGB颜色 typedef struct { uint8_t red; uint8_t green; uint8_t blue; } RGBColor; // 发送单个位 static void WS2812_SendBit(uint8_t bitVal) { if (bitVal) { // 发送1 GPIOA->BSRR = LED_PIN; asm("nop"); asm("nop"); asm("nop"); asm("nop"); asm("nop"); asm("nop"); asm("nop"); GPIOA->BRR = LED_PIN; asm("nop"); asm("nop"); } else { // 发送0 GPIOA->BSRR = LED_PIN; asm("nop"); asm("nop"); GPIOA->BRR = LED_PIN; asm("nop"); asm("nop"); asm("nop"); asm("nop"); asm("nop"); asm("nop"); } } // 发送单个字节 static void WS2812_SendByte(uint8_t byteVal) { for (int i = 0; i < 8; i++) { WS2812_SendBit(byteVal & 0x80); byteVal <<= 1; } } // 发送RGB颜色数据 void WS2812_SendRGB(RGBColor color) { WS2812_SendByte(color.green); WS2812_SendByte(color.red); WS2812_SendByte(color.blue); } // 初始化LED引脚 void LED_Init(void) { GPIO_InitTypeDef GPIO_InitStruct = {0}; __HAL_RCC_GPIOA_CLK_ENABLE(); GPIO_InitStruct.Pin = LED_PIN; GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; GPIO_InitStruct.Pull = GPIO_NOPULL; GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; HAL_GPIO_Init(LED_PORT, &GPIO_InitStruct); } int main(void) { HAL_Init(); // 初始化LED引脚 LED_Init(); while (1) { // 发送红色 RGBColor redColor = {255, 0, 0}; WS2812_SendRGB(redColor); // 延时 HAL_Delay(500); // 发送绿色 RGBColor greenColor = {0, 255, 0}; WS2812_SendRGB(greenColor); // 延时 HAL_Delay(500); // 发送蓝色 RGBColor blueColor = {0, 0, 255}; WS2812_SendRGB(blueColor); // 延时 HAL_Delay(500); } } 增加数量代码
时间: 2024-04-10 13:28:22 浏览: 69
要增加WS2812灯的数量,您需要修改发送RGB颜色数据的函数和定义更大的缓冲区。以下是相应的更改:
```c
#include "main.h"
#include "stm32g0xx_hal.h"
#define LED_PIN GPIO_PIN_5
#define LED_PORT GPIOA
#define WS2812_LOW_TIME 30 // 单位:纳秒
#define WS2812_HIGH_TIME 70 // 单位:纳秒
#define NUM_LEDS 8 // 更改为您想要的WS2812灯的数量
typedef struct
{
uint8_t red;
uint8_t green;
uint8_t blue;
} RGBColor;
uint8_t buffer[NUM_LEDS * 3];
static void WS2812_SendBit(uint8_t bitVal)
{
// 发送逻辑1或逻辑0的代码不变
}
static void WS2812_SendByte(uint8_t byteVal)
{
// 发送单个字节的代码不变
}
void WS2812_SendRGB(void)
{
for (int i = 0; i < NUM_LEDS; i++)
{
WS2812_SendByte(buffer[i * 3 + 1]); // 发送红色通道
WS2812_SendByte(buffer[i * 3]); // 发送绿色通道
WS2812_SendByte(buffer[i * 3 + 2]); // 发送蓝色通道
}
}
void LED_Init(void)
{
// 初始化LED引脚的代码不变
}
int main(void)
{
HAL_Init();
LED_Init();
while (1)
{
// 设置每个LED的颜色
for (int i = 0; i < NUM_LEDS; i++)
{
buffer[i * 3] = 255; // 设置绿色通道
buffer[i * 3 + 1] = 0; // 设置红色通道
buffer[i * 3 + 2] = 0; // 设置蓝色通道
}
WS2812_SendRGB();
HAL_Delay(500);
// 设置每个LED的颜色
for (int i = 0; i < NUM_LEDS; i++)
{
buffer[i * 3] = 0; // 设置绿色通道
buffer[i * 3 + 1] = 255; // 设置红色通道
buffer[i * 3 + 2] = 0; // 设置蓝色通道
}
WS2812_SendRGB();
HAL_Delay(500);
// 设置每个LED的颜色
for (int i = 0; i < NUM_LEDS; i++)
{
buffer[i * 3] = 0; // 设置绿色通道
buffer[i * 3 + 1] = 0; // 设置红色通道
buffer[i * 3 + 2] = 255; // 设置蓝色通道
}
WS2812_SendRGB();
HAL_Delay(500);
}
}
```
在上面的代码中,我添加了一个大小为`NUM_LEDS * 3`的缓冲区`buffer`,用于存储每个LED的RGB颜色值。在`main`函数中,我使用循环设置每个LED的颜色,并调用`WS2812_SendRGB`函数发送数据。
请注意,以上示例程序仅供参考,您可能还需要根据您的硬件设置和WS2812灯的数量进行适当的修改。同时,确保您正确配置了WS2812灯的引脚和定时器。
希望对您有所帮助!如果您有其他问题,请随时提问。
阅读全文
相关推荐
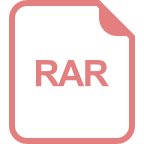
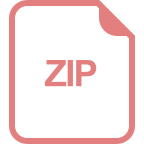
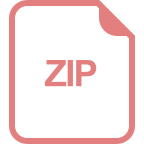















