将reducebykeyAndWindow词频统计结果分别写入文本文件和mysql数据库中
时间: 2024-05-16 15:18:10 浏览: 16
首先,我们需要将 `reduceByKeyAndWindow` 的结果转换为可以写入文本文件和 MySQL 数据库的格式,比如一个包含单词和频率的元组列表。假设我们已经完成了这个转换,下面是将结果写入文本文件和 MySQL 数据库的示例代码:
```python
# 导入必要的库
import mysql.connector
import os
# 将结果写入文本文件
def write_to_file(result, filename):
with open(filename, 'w') as f:
for word, freq in result:
f.write(f'{word}:{freq}\n')
# 将结果写入 MySQL 数据库
def write_to_mysql(result, db_config):
# 连接数据库
conn = mysql.connector.connect(**db_config)
cursor = conn.cursor()
# 创建表
cursor.execute('CREATE TABLE IF NOT EXISTS wordfreq (word VARCHAR(255), freq INT)')
# 清空表
cursor.execute('TRUNCATE TABLE wordfreq')
# 插入数据
for word, freq in result:
cursor.execute('INSERT INTO wordfreq (word, freq) VALUES (%s, %s)', (word, freq))
# 提交事务并关闭连接
conn.commit()
cursor.close()
conn.close()
# 示例代码
if __name__ == '__main__':
# 假设结果已经转换为列表形式,并存储在变量 result 中
result = [('hello', 3), ('world', 2), ('python', 1)]
# 将结果写入文本文件
write_to_file(result, 'wordfreq.txt')
# 将结果写入 MySQL 数据库
db_config = {
'host': 'localhost',
'user': 'root',
'password': 'password',
'database': 'test'
}
write_to_mysql(result, db_config)
```
请注意,这只是一个示例代码,实际应用需要根据具体情况进行修改。此外,为了将结果写入 MySQL 数据库,我们需要安装 `mysql-connector` 库。
相关推荐
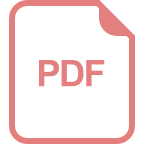
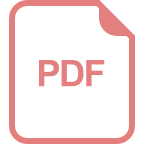
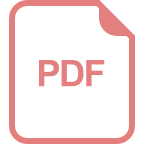











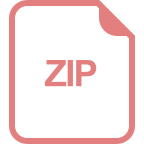
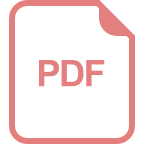
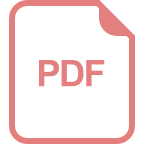
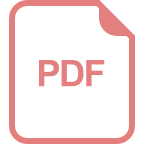