qt线程安全的单例模式
时间: 2023-07-25 08:32:10 浏览: 77
在 Qt 中,可以使用 QMutex 实现线程安全的单例模式。具体实现步骤如下:
1. 在单例类中定义一个静态成员变量,用来保存唯一实例的指针。
```cpp
class MySingleton {
public:
static MySingleton* getInstance();
private:
MySingleton();
static MySingleton* m_instance;
};
```
2. 在 getInstance 函数中,使用 QMutex 来确保线程安全。如果 m_instance 为 NULL,则创建一个新实例;否则直接返回 m_instance。
```cpp
MySingleton* MySingleton::getInstance() {
static QMutex mutex;
QMutexLocker locker(&mutex);
if (!m_instance) {
m_instance = new MySingleton;
}
return m_instance;
}
```
3. 在单例类的析构函数中删除唯一实例。
```cpp
MySingleton::~MySingleton() {
delete m_instance;
m_instance = NULL;
}
```
这样,就可以在多线程环境下安全地使用单例模式了。在使用单例的时候,只需要调用 getInstance 函数即可。
需要注意的是,如果单例类的构造函数是私有的,需要在类的定义中声明 getInstance 函数为友元函数,以便 getInstance 函数可以访问私有构造函数。
相关推荐
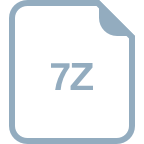














