C语言 字符串替换 【问题描述】编写一个程序,该程序将查找,搜索和替换文本字符串。 程序从输入中读取文本直到行尾。 然后,您在文本中搜索短语,并输入替换短语替换所有搜索的短语。 替换短语可以比搜索短语短或长。 在显示屏上显示更新的初始文本行。思路:从头遍历输入的字符串,每次移动一个单位,并判断从当前位置开始是否存在字符串与搜索的字符串相同,相同则进行替换
时间: 2024-03-03 15:51:05 浏览: 18
好的,以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char text[1000], search[100], replace[100], result[1000];
printf("请输入文本:");
fgets(text, 1000, stdin); // 从标准输入中读取字符串
printf("请输入要搜索的字符串:");
scanf("%s", search);
printf("请输入要替换的字符串:");
scanf("%s", replace);
int textLen = strlen(text);
int searchLen = strlen(search);
int replaceLen = strlen(replace);
int resultLen = 0;
for(int i = 0; i < textLen; i++) {
int j = 0;
while(text[i + j] == search[j] && j < searchLen) {
j++;
}
if(j == searchLen) { // 找到搜索字符串
for(int k = 0; k < replaceLen; k++) {
result[resultLen++] = replace[k]; // 将替换字符串加入结果中
}
i += searchLen - 1; // 将 i 移动到搜索字符串之后
} else {
result[resultLen++] = text[i]; // 将当前字符加入结果中
}
}
result[resultLen] = '\0'; // 确保结果字符串以空字符结尾
printf("替换后的文本为:%s\n", result);
return 0;
}
```
在这里,我们首先使用 `fgets()` 函数从标准输入中读取字符串。然后,我们分别读取要搜索的字符串和要替换的字符串,并计算出它们的长度。接下来,我们遍历输入的字符串,每次移动一个字符,并比较从当前位置开始的字符串是否与搜索字符串相同。如果是,我们将替换字符串加入结果中,并将 i 移动到搜索字符串之后。否则,我们将当前字符加入结果中。最终,我们打印出替换后的字符串。注意,在这里我们使用了一个 `result` 数组来保存结果字符串。
相关推荐
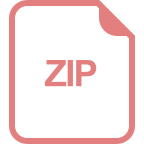
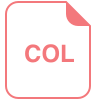
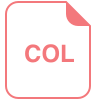
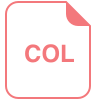
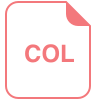
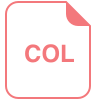









