opencv去除图像的正弦噪声,c++代码
时间: 2023-11-04 21:14:29 浏览: 24
以下是使用opencv去除图像的正弦噪声的C代码示例:
```c
#include <stdio.h>
#include <opencv2/opencv.hpp>
using namespace cv;
int main(int argc, char** argv )
{
if ( argc != 2 )
{
printf("usage: remove_noise <Image_Path>\n");
return -1;
}
Mat image, gray, dst;
image = imread( argv[1], 1 );
if ( !image.data )
{
printf("No image data \n");
return -1;
}
cvtColor(image, gray, COLOR_BGR2GRAY);
// Apply FFT to the image
Mat fft_image;
dft(gray, fft_image, DFT_COMPLEX_OUTPUT);
// Create a filter to remove the noise
Mat filter(gray.size(), CV_32FC2, Scalar(0));
const int radius = 30;
const float strength = 10.0f;
Point center(gray.cols / 2, gray.rows / 2);
for (int i = 0; i < gray.rows; i++)
{
for (int j = 0; j < gray.cols; j++)
{
float distance = norm(Point(j, i) - center);
if (distance <= radius)
{
filter.at<Vec2f>(i, j)[0] = strength;
filter.at<Vec2f>(i, j)[1] = strength;
}
}
}
// Apply the filter to the image in frequency domain
Mat filtered_image;
mulSpectrums(fft_image, filter, filtered_image, 0);
// Apply inverse FFT to get the filtered image
idft(filtered_image, dst, DFT_SCALE | DFT_REAL_OUTPUT);
// Convert the image back to uint8 format
dst.convertTo(dst, CV_8U);
namedWindow("Original Image", WINDOW_NORMAL);
namedWindow("Filtered Image", WINDOW_NORMAL);
imshow("Original Image", image);
imshow("Filtered Image", dst);
waitKey(0);
return 0;
}
```
这个代码示例首先读取图像,并将其转换为灰度图像。然后,它使用FFT(快速傅里叶变换)对图像进行处理,以便滤除正弦噪声。它创建了一个滤波器来滤除噪声,并将其应用于频域中的图像。最后,它使用逆FFT将滤波器应用于图像,并将其转换回uint8格式以进行显示。
相关推荐
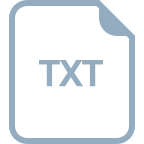




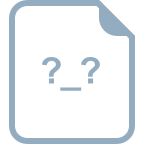
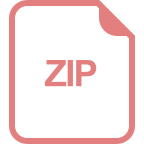
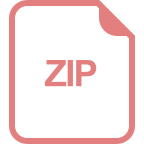
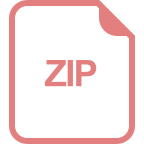
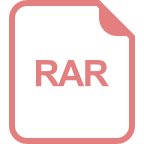
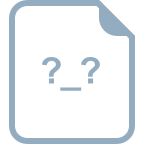
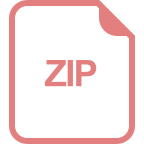