python读取Excel中的接收人的邮件信息,然后发送邮件给他们
时间: 2024-03-27 08:36:34 浏览: 138
好的,你可以使用Python中的openpyxl库来读取Excel文件,使用smtplib库来发送邮件。以下是一个简单的示例代码:
```python
import openpyxl
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.application import MIMEApplication
# 读取Excel文件
wb = openpyxl.load_workbook('emails.xlsx')
sheet = wb.active
# 邮件信息
sender_email = 'your_email@example.com'
sender_password = 'your_password'
subject = '邮件主题'
body = '邮件正文'
# 登录邮箱服务器
smtp_server = 'smtp.example.com'
smtp_port = 587
smtp = smtplib.SMTP(smtp_server, smtp_port)
smtp.starttls()
smtp.login(sender_email, sender_password)
# 发送邮件
for row in sheet.iter_rows(min_row=2, values_only=True):
recipient_email = row[0]
recipient_name = row[1]
message = MIMEMultipart()
message['From'] = sender_email
message['To'] = recipient_email
message['Subject'] = subject
# 正文
message.attach(MIMEText(f'{recipient_name},{body}', 'plain'))
# 附件
with open('attachment.pdf', 'rb') as f:
attachment = MIMEApplication(f.read(), _subtype='pdf')
attachment.add_header('Content-Disposition', 'attachment', filename='attachment.pdf')
message.attach(attachment)
smtp.sendmail(sender_email, recipient_email, message.as_string())
# 关闭邮箱服务器连接
smtp.quit()
```
以上代码将Excel文件中第一列作为接收人的邮件地址,第二列作为接收人的姓名。邮件正文中包含接收人的姓名,附件为一个名为`attachment.pdf`的PDF文件。你需要将`your_email@example.com`和`your_password`替换为你自己的邮箱地址和密码,将`smtp.example.com`替换为你的邮箱提供商的SMTP服务器地址,将`587`替换为SMTP服务器的端口号。同时,你还需要将`emails.xlsx`和`attachment.pdf`替换为你自己的Excel文件和附件文件名。
阅读全文
相关推荐
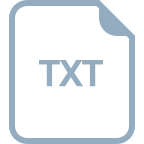
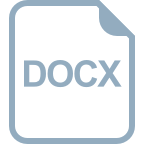
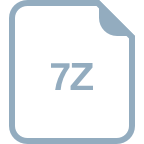
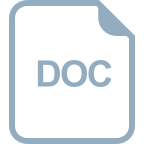
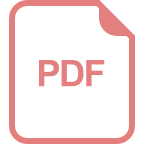
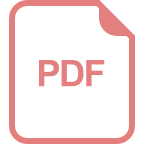
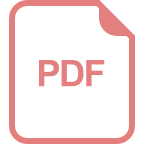
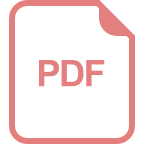
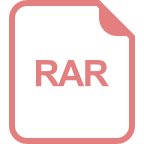
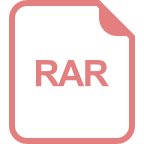
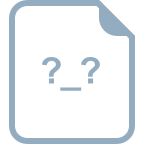
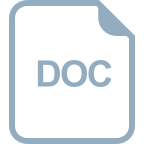
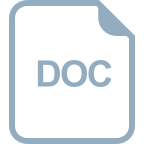
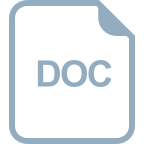


