请帮我用java写出一个五子棋游戏,要求有菜单,GUI界面
时间: 2024-03-25 08:35:33 浏览: 18
很高兴能为您提供帮助。以下是一个简单的五子棋游戏的Java代码,具有菜单和GUI界面:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class FiveInARow extends JFrame implements ActionListener {
private JButton[][] board = new JButton[15][15];
private JLabel label;
private JMenuItem newGameItem, quitItem;
private boolean player1 = true;
private boolean gameOver = false;
public FiveInARow() {
setTitle("Five In A Row");
setSize(600, 600);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
JMenu fileMenu = new JMenu("File");
menuBar.add(fileMenu);
newGameItem = new JMenuItem("New Game");
newGameItem.addActionListener(this);
fileMenu.add(newGameItem);
quitItem = new JMenuItem("Quit");
quitItem.addActionListener(this);
fileMenu.add(quitItem);
JPanel boardPanel = new JPanel();
boardPanel.setLayout(new GridLayout(15, 15));
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
board[i][j] = new JButton();
board[i][j].setBackground(Color.LIGHT_GRAY);
board[i][j].addActionListener(this);
boardPanel.add(board[i][j]);
}
}
label = new JLabel("Player 1's turn");
label.setHorizontalAlignment(JLabel.CENTER);
Container contentPane = getContentPane();
contentPane.setLayout(new BorderLayout());
contentPane.add(boardPanel, BorderLayout.CENTER);
contentPane.add(label, BorderLayout.SOUTH);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == newGameItem) {
newGame();
return;
}
if (e.getSource() == quitItem) {
System.exit(0);
}
if (gameOver) {
return;
}
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
if (e.getSource() == board[i][j]) {
if (board[i][j].getBackground() != Color.LIGHT_GRAY) {
return;
}
if (player1) {
board[i][j].setBackground(Color.BLACK);
label.setText("Player 2's turn");
} else {
board[i][j].setBackground(Color.WHITE);
label.setText("Player 1's turn");
}
player1 = !player1;
if (checkWin(i, j)) {
gameOver = true;
if (player1) {
label.setText("Player 2 wins!");
} else {
label.setText("Player 1 wins!");
}
}
return;
}
}
}
}
private void newGame() {
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
board[i][j].setBackground(Color.LIGHT_GRAY);
}
}
player1 = true;
gameOver = false;
label.setText("Player 1's turn");
}
private boolean checkWin(int row, int col) {
Color color = player1 ? Color.BLACK : Color.WHITE;
int count = 1;
for (int i = row - 1; i >= 0; i--) {
if (board[i][col].getBackground() != color) {
break;
}
count++;
}
for (int i = row + 1; i < 15; i++) {
if (board[i][col].getBackground() != color) {
break;
}
count++;
}
if (count >= 5) {
return true;
}
count = 1;
for (int j = col - 1; j >= 0; j--) {
if (board[row][j].getBackground() != color) {
break;
}
count++;
}
for (int j = col + 1; j < 15; j++) {
if (board[row][j].getBackground() != color) {
break;
}
count++;
}
if (count >= 5) {
return true;
}
count = 1;
for (int i = row - 1, j = col - 1; i >= 0 && j >= 0; i--, j--) {
if (board[i][j].getBackground() != color) {
break;
}
count++;
}
for (int i = row + 1, j = col + 1; i < 15 && j < 15; i++, j++) {
if (board[i][j].getBackground() != color) {
break;
}
count++;
}
if (count >= 5) {
return true;
}
count = 1;
for (int i = row - 1, j = col + 1; i >= 0 && j < 15; i--, j++) {
if (board[i][j].getBackground() != color) {
break;
}
count++;
}
for (int i = row + 1, j = col - 1; i < 15 && j >= 0; i++, j--) {
if (board[i][j].getBackground() != color) {
break;
}
count++;
}
if (count >= 5) {
return true;
}
return false;
}
public static void main(String[] args) {
FiveInARow game = new FiveInARow();
game.setVisible(true);
}
}
```
这个代码使用了JButton来表示棋盘上的每个位置,使用了JLabel来显示当前玩家的回合。checkWin方法用于检查是否有玩家获胜。在actionPerformed方法中,当玩家点击一个按钮时,它会检查该按钮是否可用,如果是,则将其标记为当前玩家的颜色,并检查是否有玩家获胜。如果有玩家获胜,游戏结束并显示获胜者。如果玩家点击New Game菜单项,则会重置游戏。如果玩家点击Quit菜单项,则会退出游戏。
希望这个代码能够帮助到您!
相关推荐
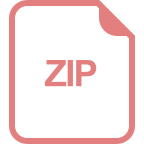
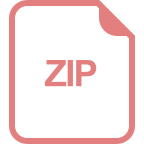














