jquery mobile酒店预定php代码
时间: 2023-07-03 18:32:09 浏览: 270
以下是一个简单的使用jQuery Mobile和PHP实现酒店预订功能的代码示例:
HTML代码:
```
<!DOCTYPE html>
<html>
<head>
<title>酒店预订</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.css">
<script src="https://code.jquery.com/jquery-1.11.3.min.js"></script>
<script src="https://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.js"></script>
</head>
<body>
<div data-role="page">
<div data-role="header">
<h1>酒店预订</h1>
</div>
<div data-role="content">
<form method="post" action="book.php">
<label for="name">姓名:</label>
<input type="text" name="name" id="name" required>
<label for="email">邮箱:</label>
<input type="email" name="email" id="email" required>
<label for="checkin">入住时间:</label>
<input type="date" name="checkin" id="checkin" required>
<label for="checkout">离店时间:</label>
<input type="date" name="checkout" id="checkout" required>
<label for="roomtype">房型:</label>
<select name="roomtype" id="roomtype" required>
<option value="">请选择房型</option>
<option value="单人间">单人间</option>
<option value="双人间">双人间</option>
<option value="豪华套房">豪华套房</option>
</select>
<label for="numofguests">客人数量:</label>
<input type="number" name="numofguests" id="numofguests" required>
<input type="submit" value="提交">
</form>
</div>
</div>
</body>
</html>
```
PHP代码(book.php):
```
<?php
// 获取表单数据
$name = $_POST['name'];
$email = $_POST['email'];
$checkin = $_POST['checkin'];
$checkout = $_POST['checkout'];
$roomtype = $_POST['roomtype'];
$numofguests = $_POST['numofguests'];
// 连接数据库
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "database";
$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn->connect_error) {
die("连接失败: " . $conn->connect_error);
}
// 插入预订信息到数据库
$sql = "INSERT INTO bookings (name, email, checkin, checkout, roomtype, numofguests)
VALUES ('$name', '$email', '$checkin', '$checkout', '$roomtype', '$numofguests')";
if ($conn->query($sql) === TRUE) {
echo "预订成功!";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
$conn->close();
?>
```
说明:
1. HTML代码使用jQuery Mobile框架实现页面布局和表单控件的样式。
2. PHP代码首先获取表单数据,然后连接数据库并将预订信息插入到数据库中。
3. 数据库表格(bookings)的结构为:id(自增主键)、name、email、checkin、checkout、roomtype、numofguests。
阅读全文
相关推荐
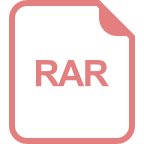

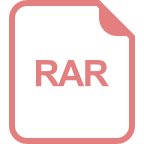
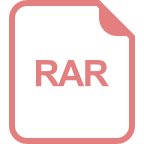


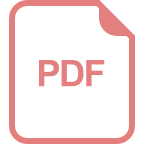
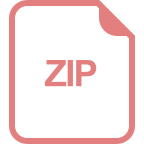
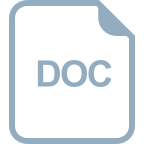
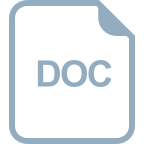
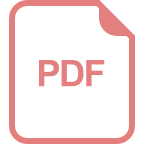
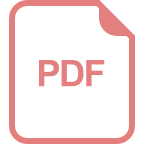
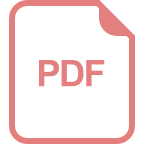
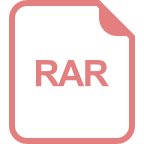
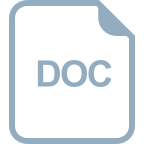
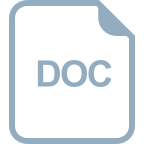
