基于python和flask的农产品数据可视化,主要功能数据爬取,数据分析代码
时间: 2024-03-30 11:33:15 浏览: 145
以下是一个简单的基于Python和Flask的农产品数据可视化的示例代码,其中包括数据爬取和数据分析的基本功能。
1. 数据爬取部分示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 爬取天气数据
def get_weather():
url = 'https://www.weather.com.cn/weather/101010100.shtml'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
temp = soup.select(".tem i")
weather = soup.select(".wea")
return {'temp': temp[0].text, 'weather': weather[0].text}
# 爬取农产品价格数据
def get_price():
url = 'http://nc.mofcom.gov.cn/channel/gxdj/jghq/jg_list.shtml'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
table = soup.find_all('table')[0]
rows = table.find_all('tr')
prices = []
for row in rows[1:]:
cols = row.find_all('td')
prices.append({'name': cols[0].text, 'price': cols[1].text})
return prices
# 爬取土壤质量数据
def get_soil():
url = 'http://www.tureal.com.cn/special/20171107/3147.html'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
data = soup.select(".table-data")[0].text
return data
```
2. 数据分析部分示例代码:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 分析天气数据
def analyze_weather():
data = pd.read_csv('weather.csv')
data['temp'] = data['temp'].apply(lambda x: int(x.replace('℃', '')))
temp_mean = data['temp'].mean()
temp_std = data['temp'].std()
plt.hist(data['temp'], bins=20)
plt.title('Temperature Distribution')
plt.xlabel('Temperature')
plt.ylabel('Frequency')
plt.figtext(0.7, 0.8, 'Mean: %.2f\nStandard Deviation: %.2f' % (temp_mean, temp_std))
plt.show()
# 分析农产品价格数据
def analyze_price():
data = pd.read_csv('price.csv')
price_mean = data['price'].mean()
price_std = data['price'].std()
plt.plot(data['price'])
plt.title('Price Trend')
plt.xlabel('Time')
plt.ylabel('Price')
plt.figtext(0.7, 0.8, 'Mean: %.2f\nStandard Deviation: %.2f' % (price_mean, price_std))
plt.show()
# 分析土壤质量数据
def analyze_soil():
data = pd.read_csv('soil.csv')
# 进行数据清洗和处理
# ...
```
3. Flask应用部分示例代码:
```python
from flask import Flask, render_template, jsonify
import json
app = Flask(__name__)
# 页面路由
@app.route('/')
def index():
return render_template('index.html')
# API接口路由
@app.route('/weather')
def weather():
data = get_weather()
return jsonify(data)
@app.route('/price')
def price():
data = get_price()
return jsonify(data)
@app.route('/soil')
def soil():
data = get_soil()
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
```
以上是一个简单的示例代码,供您参考。实际应用中需要根据具体需求进行更加详细的实现。
阅读全文
相关推荐
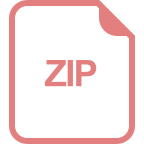
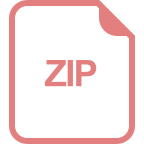

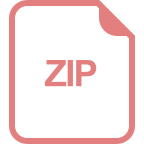
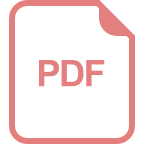
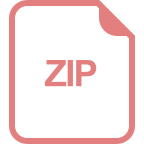
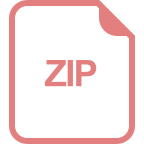
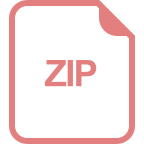
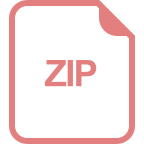
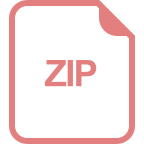
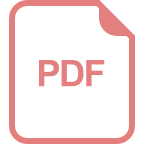
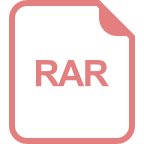
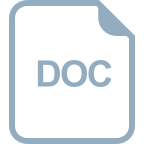
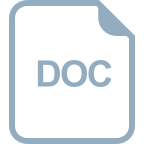
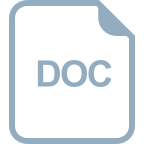
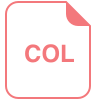
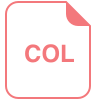
