#include <BluetoothSerial.h> #include <Button2.h> BluetoothSerial SerialBT; // Define the service UUID #define SERVICE_UUID "4fafc201-1fb5-459e-8fcc-c5c9c331914b" // Define the characteristics UUID #define CHARACTERISTIC_UUID_RX "c3e9aa9d-b4e4-4b8e-bd99-b57ad4f177b1" #define CHARACTERISTIC_UUID_TX "c3e9aa9d-b4e4-4b8e-bd99-b57ad4f177b2" // 定義按鈕接在的 GPIO 腳位 #define BUTTON_PIN 23 char val; int c = 1 ; int buttonState = 0 ; // 宣告按鈕物件,並設置 debounce 時間為 50 毫秒 Button2 button(BUTTON_PIN, INPUT_PULLUP, 50); void setup() { Serial.begin(9600); SerialBT.begin("ESP32-Client"); // 初始化蓝牙串行端口,命名为“Client” pinMode(BUTTON_PIN, INPUT_PULLUP); Serial.println("Bluetooth is ready to go!"); } void loop() { int buttonState = digitalRead(BUTTON_PIN); int i =random(100); if (SerialBT.available()) { String data = SerialBT.readString(); Serial.println("Received data: " + data); // 接收到來自Server端的資料後,輸出到Serial通訊端口 val = SerialBT.read() ; if( val == 'a' ) { SerialBT.println(i) ; delay(50 ) ; Serial.flush() ; }else if( val == 'b' ) { if(digitalRead(buttonState) == HIGH) { Serial.println( "" ) ; SerialBT.print( c ) ; }else { Serial.println( "no" ) ; // digitalWrite( led_4, HIGH ) ; } } } } 改成nano33的程式
时间: 2023-06-16 16:03:32 浏览: 58
抱歉,这段代码无法直接在Nano 33 BLE上运行,因为它使用了ESP32的蓝牙串行端口。你需要重新编写代码,使用Nano 33 BLE的蓝牙库进行蓝牙通信。以下是一个简单的例子,可以帮助你开始:
```c++
#include <ArduinoBLE.h>
#include <Button.h>
BLEService service(SERVICE_UUID);
BLECharacteristic rxCharacteristic(CHARACTERISTIC_UUID_RX, BLEWriteWithoutResponse | BLEWrite, 20);
BLECharacteristic txCharacteristic(CHARACTERISTIC_UUID_TX, BLERead | BLENotify, 20);
// 定義按鈕接在的 GPIO 腳位
const int BUTTON_PIN = 2;
Button button(BUTTON_PIN);
void setup() {
Serial.begin(9600);
while (!Serial);
if (!BLE.begin()) {
Serial.println("Starting BLE failed!");
while (1);
}
BLE.setLocalName("Nano33BLE-Client");
BLE.setAdvertisedService(service);
service.addCharacteristic(rxCharacteristic);
service.addCharacteristic(txCharacteristic);
BLE.addService(service);
txCharacteristic.setValue("Hello, Server!"); // 初始化 TX 特徵值
BLE.advertise();
Serial.println("BLE is ready to go!");
}
void loop() {
BLEDevice central = BLE.central();
if (central) {
Serial.print("Connected to central: ");
Serial.println(central.address());
while (central.connected()) {
if (rxCharacteristic.written()) {
String data = rxCharacteristic.value();
Serial.println("Received data: " + data);
char val = data.charAt(0);
if (val == 'a') {
int i = random(100);
txCharacteristic.writeValue(String(i));
} else if (val == 'b') {
if (button.isPressed()) {
txCharacteristic.writeValue("Button pressed!");
} else {
txCharacteristic.writeValue("Button not pressed!");
}
}
}
}
Serial.println("Disconnected from central");
}
}
```
这段代码使用了ArduinoBLE库,它提供了适用于Nano 33 BLE的蓝牙功能。在这个例子中,我们创建了一个BLE服务和两个BLE特征,分别用于读取和写入数据。我们还使用Button库来检测连接到Nano 33 BLE的按钮是否按下。在循环中,我们等待与中央设备的连接,并在连接建立时处理来自中央设备的数据。如果收到了'a',则生成一个随机数并将其写回中央设备。如果收到了'b',则检查按钮是否按下,并将相应的消息写回中央设备。
请注意,这只是一个简单的例子,你需要根据你的具体需求进行修改。例如,你可能需要添加安全性措施来防止未经授权的访问。
相关推荐
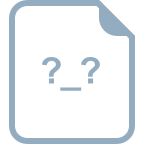
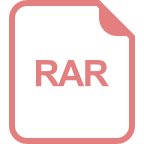
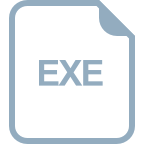
















