使用MPI编写一个程序,实现水仙花数的计算。水仙花数是指3位数字,其每个数字的立方和等于该数字本身。你的程序应该从命令行接收一个参数n,表示要计算n位数是否为水仙花数。你的程序应打印出所有n位水仙花数。
时间: 2024-03-27 15:39:35 浏览: 10
好的,我可以帮你编写这个程序。首先需要引入 MPI 头文件,并初始化 MPI 环境:
```c
#include <stdio.h>
#include <stdlib.h>
#include <mpi.h>
int main(int argc, char** argv) {
int rank, size;
MPI_Init(&argc, &argv);
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
MPI_Comm_size(MPI_COMM_WORLD, &size);
```
接下来,我们需要解析命令行参数,获取要计算的位数 n:
```c
if (argc != 2) {
if (rank == 0) {
printf("Usage: %s <n>\n", argv[0]);
}
MPI_Finalize();
return EXIT_FAILURE;
}
int n = atoi(argv[1]);
if (n <= 0) {
if (rank == 0) {
printf("Invalid argument: n should be a positive integer.\n");
}
MPI_Finalize();
return EXIT_FAILURE;
}
if (n < 3 && rank == 0) {
printf("There is no %d-digit narcissistic number.\n", n);
}
```
然后,我们需要计算出每个进程要处理的数字范围。因为我们要打印出所有的水仙花数,所以可以使用暴力枚举法来计算。每个进程处理的范围应该是从 10^(n-1) 到 10^n - 1。
```c
int start = 1, end = 10;
for (int i = 1; i < n; i++) {
start *= 10;
end *= 10;
}
int count = 0;
for (int num = start + rank; num < end; num += size) {
int sum = 0;
int tmp = num;
while (tmp > 0) {
int digit = tmp % 10;
sum += digit * digit * digit;
tmp /= 10;
}
if (sum == num) {
printf("%d is a narcissistic number.\n", num);
count++;
}
}
```
最后,我们需要将每个进程找到的水仙花数的个数相加,输出总共找到的水仙花数。并且在程序结束时需要调用 MPI_Finalize 函数,释放 MPI 环境。
```c
int total_count;
MPI_Reduce(&count, &total_count, 1, MPI_INT, MPI_SUM, 0, MPI_COMM_WORLD);
if (rank == 0) {
printf("Found %d narcissistic numbers in total.\n", total_count);
}
MPI_Finalize();
return EXIT_SUCCESS;
}
```
完整代码如下:
相关推荐
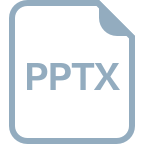
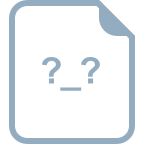














