javaFx线程池实现进度条
时间: 2023-08-31 17:05:22 浏览: 111
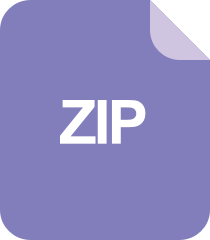
java实现进度条

要在JavaFX应用程序中使用线程池来实现进度条,可以按照以下步骤操作:
1. 创建一个JavaFX应用程序,包含一个进度条和一个按钮。
2. 当用户单击按钮时,启动一个线程池任务。
3. 在任务中,使用Platform.runLater()方法更新进度条。
下面是一个示例代码,演示了如何在JavaFX应用程序中使用线程池和进度条:
```java
import javafx.application.Application;
import javafx.application.Platform;
import javafx.concurrent.Task;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ProgressBar;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class Main extends Application {
private ProgressBar progressBar;
@Override
public void start(Stage primaryStage) throws Exception {
Button button = new Button("Start Task");
progressBar = new ProgressBar(0);
StackPane root = new StackPane();
root.getChildren().addAll(progressBar, button);
primaryStage.setScene(new Scene(root, 300, 250));
primaryStage.show();
button.setOnAction(event -> {
ExecutorService executor = Executors.newFixedThreadPool(1);
Task<Void> task = new Task<Void>() {
@Override
protected Void call() throws Exception {
for (int i = 0; i < 100; i++) {
updateProgress(i, 100);
Thread.sleep(50);
}
return null;
}
};
progressBar.progressProperty().bind(task.progressProperty());
task.setOnSucceeded(e -> {
executor.shutdown();
});
executor.submit(task);
});
}
public static void main(String[] args) {
launch(args);
}
}
```
在这个示例中,我们创建了一个进度条和一个按钮,并将它们添加到一个StackPane布局中。
当用户单击按钮时,我们启动了一个线程池任务,该任务将更新进度条的值。我们使用Platform.runLater()方法来确保更新操作在JavaFX应用程序线程上执行。
我们还使用progressBar.progressProperty().bind(task.progressProperty())方法来绑定进度条的值和任务的进度值。这将确保进度条的值与任务的进度保持同步。
当任务完成时,我们关闭线程池。
阅读全文
相关推荐
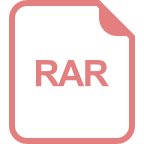

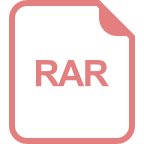
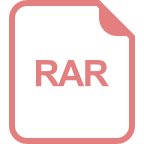
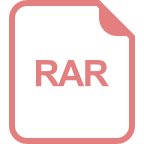
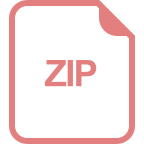
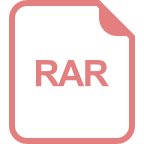
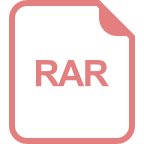
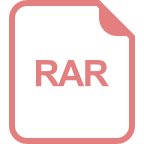
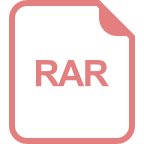
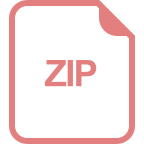
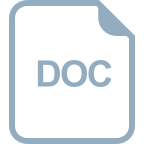
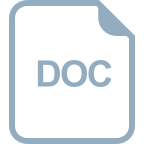
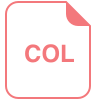
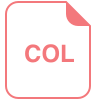
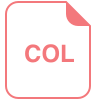
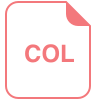