用python爬取2345天气网的数据
时间: 2023-08-12 09:09:10 浏览: 42
好的,以下是使用Python爬取2345天气网数据的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 发送请求
url = 'https://tianqi.2345.com/'
response = requests.get(url, headers=headers)
# 解析HTML内容
soup = BeautifulSoup(response.content, 'html.parser')
# 获取天气信息
weather_info = soup.find('div', class_='weather-info')
print(weather_info.text.strip())
```
上述代码中使用了requests库和BeautifulSoup库。首先设置请求头,然后发送请求获取网页内容,接着使用BeautifulSoup库解析HTML内容,最后从HTML中获取天气信息并输出。您可以根据自己的需求修改代码以获取更多的天气信息。
相关问题
用python爬取2345天气网的全年数据
好的,以下是使用Python爬取2345天气网全年数据的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 获取全年天气数据
for month in range(1, 13):
url = f'https://tianqi.2345.com/month/{month}.htm'
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
month_table = soup.find('table', class_='tqtongji2')
rows = month_table.find_all('tr')
for row in rows[1:]:
tds = row.find_all('td')
date = tds[0].text.strip()
weather = tds[1].text.strip()
temp_high = tds[2].text.strip()
temp_low = tds[3].text.strip()
print(f'{date} | {weather} | 最高温度:{temp_high}℃ | 最低温度:{temp_low}℃')
```
上述代码中,我们使用循环遍历每个月份的URL,然后发送请求获取网页内容,使用BeautifulSoup库解析HTML内容。在每个月的HTML中找到对应的表格,遍历表格中的每一行,获取天气数据并输出。您可以根据自己的需求修改代码以获取更多的天气数据。需要注意的是,2345天气网有反爬机制,请勿频繁访问。
python爬取2345天气网24小时天气
可以使用 Python 中的 requests 和 BeautifulSoup 库来实现爬取 2345 天气网的 24 小时天气数据。以下是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'http://tianqi.2345.com/wea_history/59758.htm'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
hourly_weather = []
hourly_weather_soup = soup.find('div', {'class': 'tb-weather-his-hour'})
hourly_weather_items = hourly_weather_soup.find_all('dl')
for item in hourly_weather_items:
hour = item.find('dt').text.strip()
weather = item.find('span', {'class': 'wea'}).text.strip()
temperature = item.find('span', {'class': 'tem'}).text.strip()
hourly_weather.append({'hour': hour, 'weather': weather, 'temperature': temperature})
print(hourly_weather)
```
需要注意的是,上述代码中的 `url` 是一个示例链接,需要根据实际情况替换成你所需要获取天气数据的链接。此外,还需要注意解析 HTML 的方式,这里我选择使用了 BeautifulSoup 库。
阅读全文
相关推荐
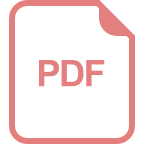
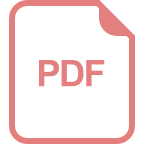





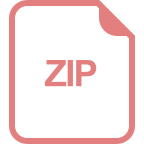
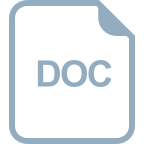



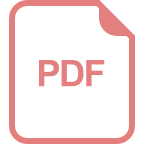