边缘检测:使用边缘检测算法(如Canny算法)找到物体的边缘,然后利用轮廓检测算法(如findContours函数)获取物体的轮廓信息,在轮廓信息中找到最小外接矩形(boundingRect函数),并计算矩形的中心坐标。代码
时间: 2024-06-08 17:07:46 浏览: 15
使用OpenCV库可以很方便地实现边缘检测和轮廓检测,以下是一个简单的示例代码:
```python
import cv2
# 读取图像
img = cv2.imread('example.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 边缘检测
edges = cv2.Canny(gray, 100, 200)
# 轮廓检测
contours, hierarchy = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 绘制轮廓
cv2.drawContours(img, contours, -1, (0, 0, 255), 2)
# 获取最小外接矩形
rect = cv2.boundingRect(contours[0])
# 计算矩形中心坐标
center_x = rect[0] + rect[2] / 2
center_y = rect[1] + rect[3] / 2
# 在图像上绘制矩形和中心点
cv2.rectangle(img, (rect[0], rect[1]), (rect[0]+rect[2], rect[1]+rect[3]), (0, 255, 0), 2)
cv2.circle(img, (int(center_x), int(center_y)), 5, (255, 0, 0), -1)
# 显示图像
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`cv2.Canny`函数用于边缘检测,其前两个参数分别为低阈值和高阈值,可根据具体情况进行调整;`cv2.findContours`函数用于轮廓检测,其第二个参数指定轮廓的检索模式,第三个参数指定轮廓的近似方法;`cv2.boundingRect`函数用于获取最小外接矩形,返回值为矩形的左上角坐标、宽度和高度。在绘制矩形和中心点时,可以根据需要调整颜色、线宽等参数。
相关推荐
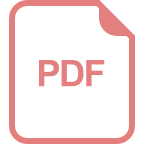
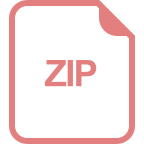
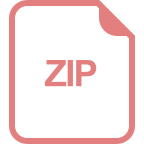
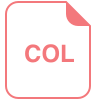
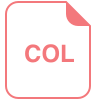
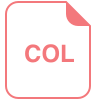
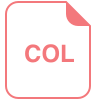
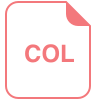









