void TIMx_DMA_IRQHandler(void) { HAL_DMA_IRQHandler(TimHandle.hdma[TIM_DMA_ID_CC1]); }
时间: 2023-10-11 19:08:35 浏览: 184
这段代码定义了一个中断处理函数TIMx_DMA_IRQHandler,用于处理定时器通道1的DMA传输完成中断。在函数中,调用HAL库函数HAL_DMA_IRQHandler,将中断处理交给HAL库处理。具体地,通过传入定时器的DMA句柄中的hdma[TIM_DMA_ID_CC1]参数,将DMA传输中断的处理交给HAL库进行处理,从而实现对定时器通道1 DMA传输中断的响应。
相关问题
stm32标准库用dma将定时器的计数值搬运到存储器的代码
STM32标准库中利用DMA (Direct Memory Access) 将定时器的计数值搬运到内存通常涉及到以下几个步骤:
1. 首先,你需要配置定时器(Timer)。例如,如果你使用的是TIMx定时器,可以设置其工作模式、预分频值和捕获/比较寄存器。
```c
TIM_HandleTypeDef htim;
TIM_ClockConfigTypeDef sClockSourceConfig;
// 初始化TIMx
htim.Instance = TIMx;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIMx, ENABLE);
TIM_TimeBaseInit(&htim, &sTimeBaseStructure);
// 设置定时器的工作模式(如PWM模式)
TIM_MasterConfigTypeDef sMasterConfig;
TIM_MasterSlaveConfig(htim.Instance, TIM_MasterActive, TIM_SlavePassive);
// 如果需要,配置时钟源
sClockSourceConfig.ClockSource = TIM_CK_PrescalerSource_ITR; // 使用内部时钟
TIM_ClockSourceConfig(&htim, &sClockSourceConfig);
```
2. 然后配置DMA用于传输数据。假设你要从TIMx的Capture Compare Register(CCRn)传输数据到内存地址`MemoryAddress`。你需要初始化一个DMA通道,并设置正确的传输参数。
```c
DMA_HandleTypeDef hdma_tim_to_mem;
// 初始化DMA
hdma_tim_to_mem.Init.Channel = DMA_Channel_1; // DMA channel you want to use
hdma_tim_to_mem.Init.Direction = DMA_MemoryToPeripheral;
hdma_tim_to_mem.Init.PeriphInc = DMA_PeripheralInc_Disable;
hdma_tim_to_mem.Init.MemInc = DMA_MemoryInc_Enable;
hdma_tim_to_mem.Init.PeriphDataAlignment = DMA_PeripheralDataAlignment_Word;
hdma_tim_to_mem.Init.MemoryDataAlignment = DMA_MemoryData Alignment_Word;
hdma_tim_to_mem.Init.Mode = DMA_Mode_Normal;
hdma_tim_to_mem.Init.Priority = DMA_PRIORITY_HIGH;
hdma_tim_to_mem.Init.FIFOMode = DMA_FIFOMode_Disabled;
hdma_tim_to_mem.Init.Request = DMARequest_None;
// 将DMA链接到TIMx的某个事件
hdma_tim_to_mem.Init TransferDirection = DMA_TransferFromMemory;
hdma_tim_to_mem.Init_IRQn = TIMx_DMA_IRQn;
hdma_tim_to_mem.Init.ErrorCallback = NULL;
HAL_DMA_Init(&hdma_tim_to_mem);
```
3. 绑定DMA请求到定时器的特定事件(例如, Capture Compare Event),并启动DMA。
```c
HAL_TIM_DMACConnect(TIMx, TIM_DMABurstLength_1Transfer, DMA_CHANNEL_1, TIMxCCRnCompare_ID); // replace n with your CCR number
// 开启DMA
HAL_DMA_Start_IT(&hdma_tim_to_mem);
```
4. 最后,记得注册中断处理函数,当DMA传输完成时会触发这个函数。
```c
void TIMx_DMA_IRQHandler(DMA_HandleTypeDef *hdma)
{
if(hdma == &hdma_tim_to_mem)
{
HAL_DMA_IRQHandler(hdma);
// 根据需要更新内存位置并清除标志
MemoryAddress += sizeof(uint16_t); // assuming uint16_t data size
// Clear the DMA flag in the timer peripheral
TIM_ClearFlag(TIMx, TIM_FLAG_Update);
}
}
```
注意:实际代码中可能需要根据你的硬件配置、数据类型和目标内存地址做相应的调整。在使用之前确保对相关的头文件进行了包含,如`stm32f4xx_hal_dmac.h`等。此外,以上示例假设了每个定时器周期产生一个16位的数据值。
阅读全文
相关推荐
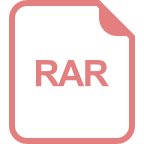
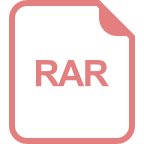
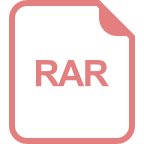
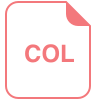
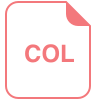
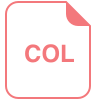
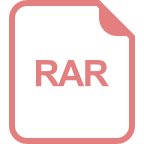
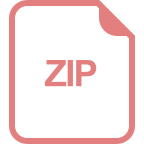
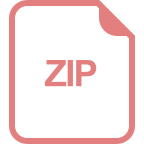
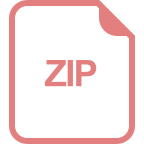
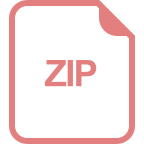
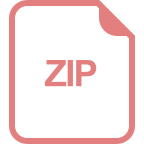
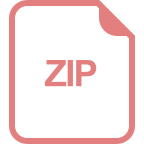
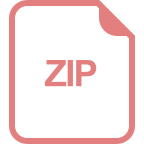