vue循环出来的 鼠标移入移出改变其中一个背景颜色
时间: 2024-05-03 18:20:22 浏览: 116
您好,关于您的问题,可以使用Vue的指令v-bind:class和v-on:mouseover/v-on:mouseout绑定class和鼠标事件。具体代码如下:
```html
<div v-for="(item, index) in items"
v-bind:key="index"
v-bind:class="{ 'highlight': item.highlight }"
v-on:mouseover="item.highlight = true"
v-on:mouseout="item.highlight = false">
{{ item.text }}
</div>
```
其中,items为渲染数组,highlight为每个元素是否高亮的标志位。当鼠标移入元素时,对应元素的highlight会被设置为true,从而触发v-bind:class中的class绑定;当鼠标移出元素时,对应元素的highlight会被设置为false,从而解除highlight对应的class绑定。
希望这能够帮助您解决问题。如有疑问,请随时向我提问。
相关问题
vue循环出来的div div里面有删除按钮 鼠标移入移出改变其中一个按钮的背景颜色
您可以使用Vue的事件绑定和条件渲染来实现鼠标移入移出改变按钮背景颜色的效果。具体做法是:
1. 给删除按钮绑定mouseenter和mouseleave事件,分别触发对应的处理方法。
2. 在数据模型中添加一个属性用来表示当前鼠标是否在按钮上。
3. 在模板中使用v-bind:class指令来动态绑定按钮的class,根据鼠标是否在按钮上来决定是否添加特定的class来改变背景颜色。
示例代码如下:
```
<template>
<div v-for="(item, index) in list" :key="index">
<div>
{{item.text}}
<button class="delete-button"
@mouseenter="setButtonHover(true, index)"
@mouseleave="setButtonHover(false, index)"
@click="deleteItem(index)">
删除
</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
list: [
{text: 'item1'},
{text: 'item2'},
{text: 'item3'},
],
buttonHover: [],
}
},
methods: {
setButtonHover(hover, index) {
this.$set(this.buttonHover, index, hover);
},
deleteItem(index) {
this.list.splice(index, 1);
this.$delete(this.buttonHover, index);
},
},
}
</script>
<style>
.delete-button:hover {
background-color: gray;
}
.delete-button.hover {
background-color: green;
}
</style>
```
在模板中使用v-bind:class来绑定按钮的class,根据buttonHover数组中的值来判断是否添加.hover类。用this.$set和this.$delete来更新数组中某个元素的值。使用的是Vue的响应式设置。注意删除元素时,也要删除按钮hover的状态。
vue循环出来的div div里面右侧有删除按钮 鼠标移入移出改变其中一个删除按钮的背景颜色
要实现这个功能可以使用Vue的指令v-bind:class和v-on:mouseenter以及v-on:mouseleave。
首先,在循环中给每个删除按钮添加唯一的id或者索引值,在template中通过v-bind:class绑定一个类名,该类名默认不显示背景颜色。
然后,在该删除按钮上绑定鼠标移入和移出事件v-on:mouseenter和v-on:mouseleave,在事件方法中修改该删除按钮的类名,使其显示背景颜色。
代码示例:
```
<template>
<div v-for="(item, index) in list" :key="item.id">
{{item.title}}
<div class="delete-btn" :class="{color: activeIndex === index}" @mouseenter="handleMouseenter(index)" @mouseleave="handleMouseleave(index)">
删除
</div>
</div>
</template>
<script>
export default {
data() {
return {
list: [{id: 1, title: 'item1'}, {id: 2, title: 'item2'}, {id: 3, title: 'item3'}],
activeIndex: null
}
},
methods: {
handleMouseenter(index) {
this.activeIndex = index
},
handleMouseleave() {
this.activeIndex = null
}
}
}
</script>
<style>
.delete-btn {
display: inline-block;
background-color: #fff;
border: 1px solid #ccc;
padding: 5px 10px;
cursor: pointer;
}
.delete-btn.color {
background-color: #f00;
color: #fff;
}
</style>
```
阅读全文
相关推荐
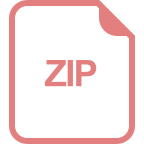
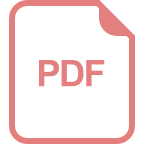

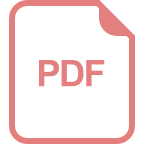
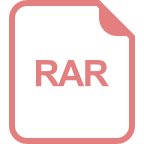
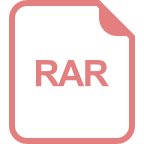
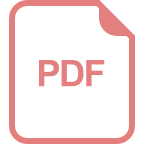
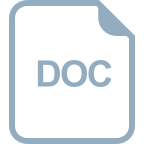
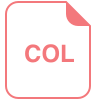
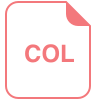




