在spring boot在用thyme leaf模板写一个用css和js使用get方法提交水泵电流和电压页面的输入输出
时间: 2023-06-04 07:04:05 浏览: 156
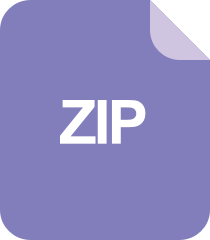
thymeleafexamples-stsm:Spring Thyme Seedstarter Manager-“ Thymeleaf + Spring 3”教程的配套应用程序,可从Thymeleaf网站下载
答:这个问题属于技术问题,我可以回答。
您可以在Thymeleaf模板中使用HTML表单来创建一个方便的方式提交详细信息。例如,您可以在模板中使用以下代码片段来创建一个表单:
<form th:action="@{/submit}" method="get">
<label for="current">Current:</label>
<input id="current" type="text" name="current" />
<label for="voltage">Voltage:</label>
<input id="voltage" type="text" name="voltage" />
<button type="submit">Submit</button>
</form>
在这个例子中,用户会看到一个表单,用户可以在表单中输入水泵的电流和电压,然后点击提交按钮。接着该页面会发送 GET 请求到服务器地址为 /submit 的 URL。
在您的 Spring Boot 应用程序中,您需要创建一个控制器来处理这个请求,这个控制器应该能够接收到输入的电流和电压,然后将它们保存到数据库中。
相信这个例子可以帮助您解决问题。
阅读全文
相关推荐
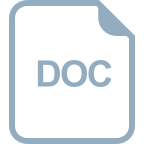
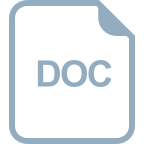

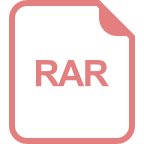


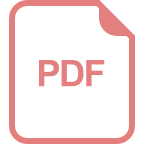
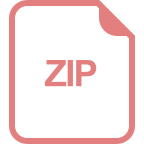
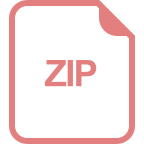
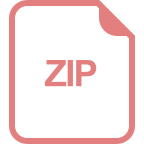
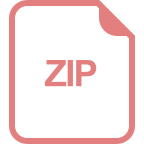
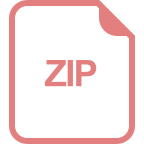
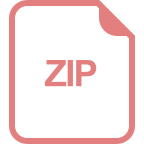
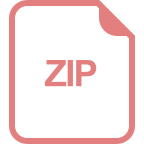
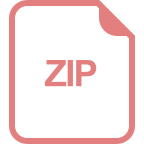
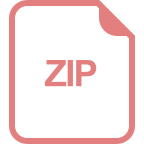
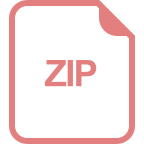