帮我用C语言写一个用队列走迷宫的程序,迷宫随机生成7*7的数组
时间: 2023-05-17 13:07:56 浏览: 111
好的,以下是用C语言写的用队列走迷宫的程序,迷宫随机生成7*7的数组的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define ROW 7
#define COL 7
typedef struct {
int row;
int col;
} Point;
typedef struct {
Point data[ROW * COL];
int front;
int rear;
} Queue;
void initQueue(Queue *q) {
q->front = q->rear = 0;
}
int isEmpty(Queue *q) {
return q->front == q->rear;
}
void enQueue(Queue *q, Point p) {
q->data[q->rear++] = p;
}
Point deQueue(Queue *q) {
return q->data[q->front++];
}
int isVisited[ROW][COL];
int maze[ROW][COL] = {
{0, 0, 0, 0, 0, 0, 0},
{0, 1, 1, 0, 1, 1, 0},
{0, 1, 0, 0, 0, 1, 0},
{0, 1, 1, 1, 0, 1, 0},
{0, 0, 0, 1, 0, 1, 0},
{0, 1, 0, 0, 0, 1, 0},
{0, 1, 1, 1, 1, 1, 0}
};
void printMaze() {
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
printf("%d ", maze[i][j]);
}
printf("\n");
}
}
void generateMaze() {
srand(time(NULL));
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
maze[i][j] = rand() % 2;
}
}
maze[0][0] = 1;
maze[ROW - 1][COL - 1] = 1;
}
int bfs() {
Queue q;
initQueue(&q);
Point start = {0, 0};
enQueue(&q, start);
isVisited[0][0] = 1;
while (!isEmpty(&q)) {
Point cur = deQueue(&q);
if (cur.row == ROW - 1 && cur.col == COL - 1) {
return 1;
}
if (cur.row - 1 >= 0 && maze[cur.row - 1][cur.col] == 1 && !isVisited[cur.row - 1][cur.col]) {
Point next = {cur.row - 1, cur.col};
enQueue(&q, next);
isVisited[next.row][next.col] = 1;
}
if (cur.row + 1 < ROW && maze[cur.row + 1][cur.col] == 1 && !isVisited[cur.row + 1][cur.col]) {
Point next = {cur.row + 1, cur.col};
enQueue(&q, next);
isVisited[next.row][next.col] = 1;
}
if (cur.col - 1 >= 0 && maze[cur.row][cur.col - 1] == 1 && !isVisited[cur.row][cur.col - 1]) {
Point next = {cur.row, cur.col - 1};
enQueue(&q, next);
isVisited[next.row][next.col] = 1;
}
if (cur.col + 1 < COL && maze[cur.row][cur.col + 1] == 1 && !isVisited[cur.row][cur.col + 1]) {
Point next = {cur.row, cur.col + 1};
enQueue(&q, next);
isVisited[next.row][next.col] = 1;
}
}
return 0;
}
int main() {
generateMaze();
printMaze();
if (bfs()) {
printf("Can reach the end!\n");
} else {
printf("Can not reach the end!\n");
}
return 0;
}
```
这个程序使用了广度优先搜索算法来寻找从起点到终点的路径。首先,我们定义了一个 Point 结构体来表示迷宫中的一个点,然后定义了一个 Queue 结构体来表示队列。接着,我们定义了一些辅助函数,如 initQueue、isEmpty、enQueue 和 deQueue,用于操作队列。然后,我们定义了一个 isVisited 数组来记录每个点是否已经被访问过,以避免重复访问。接着,我们定义了一个 7*7 的迷宫数组 maze,并使用 generateMaze 函数来随机生成迷宫。最后,我们使用 bfs 函数来进行广度优先搜索,找到从起点到终点的路径。如果找到了路径,就输出 "Can reach the end!",否则输出 "Can not reach the end!"。
阅读全文
相关推荐
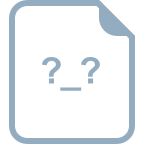
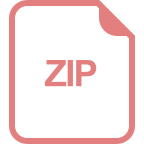
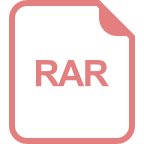
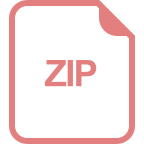
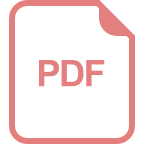
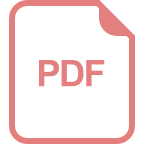
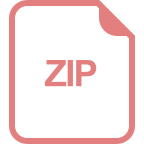
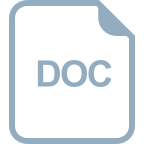
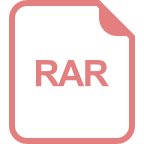
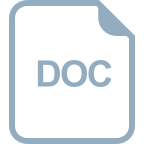
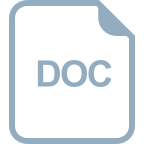




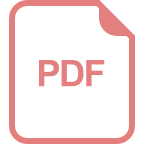