C语言实现单循环链表的产生,查找,插入,删除,输出
时间: 2024-05-14 20:11:55 浏览: 87
单循环链表是一种链表形式,它的最后一个节点指向链表的头节点,形成一个环。下面是C语言实现单循环链表的代码:
```c
#include <stdio.h>
#include <stdlib.h>
/* 定义单循环链表节点结构体 */
typedef struct node {
int data;
struct node *next;
} Node;
/* 初始化单循环链表 */
Node *initList() {
Node *head = (Node *)malloc(sizeof(Node));
head->next = head;
return head;
}
/* 输出单循环链表 */
void printList(Node *head) {
Node *p = head->next;
while (p != head) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
/* 查找单循环链表 */
Node *findNode(Node *head, int data) {
Node *p = head->next;
while (p != head) {
if (p->data == data) {
return p;
}
p = p->next;
}
return NULL;
}
/* 插入单循环链表 */
void insertNode(Node *head, int data) {
Node *p = (Node *)malloc(sizeof(Node));
p->data = data;
p->next = head->next;
head->next = p;
}
/* 删除单循环链表 */
void deleteNode(Node *head, int data) {
Node *p = head->next;
Node *prev = head;
while (p != head) {
if (p->data == data) {
prev->next = p->next;
free(p);
return;
}
prev = p;
p = p->next;
}
}
int main() {
Node *head = initList();
insertNode(head, 1);
insertNode(head, 2);
insertNode(head, 3);
printList(head);
deleteNode(head, 2);
printList(head);
Node *node = findNode(head, 3);
if (node != NULL) {
printf("find node: %d\n", node->data);
}
return 0;
}
```
上面的代码实现了单循环链表的初始化、输出、查找、插入和删除操作。其中,初始化操作将头节点的next指针指向自己,表示链表为空;输出操作遍历链表的所有节点,直到回到头节点;查找操作从头节点开始遍历链表,查找节点数据等于给定值的节点;插入操作在链表头部插入一个新节点;删除操作从头节点开始遍历链表,查找数据等于给定值的节点,并删除它。
阅读全文
相关推荐
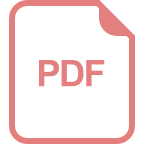
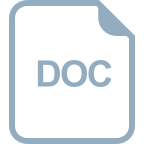
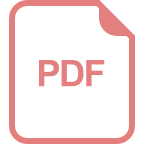
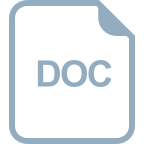
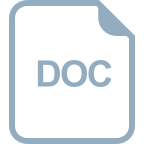

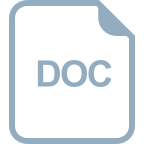
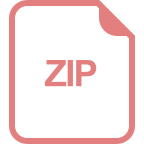
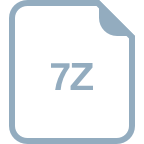
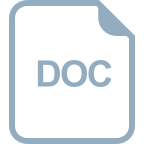
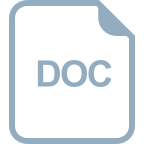
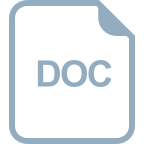
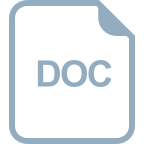
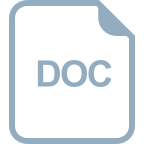
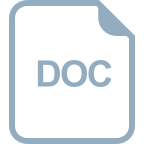
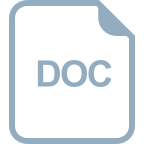
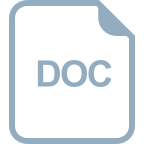