c语言获取json对象中的所有键值
时间: 2023-12-10 10:42:06 浏览: 278
在 C 语言中,可以使用 cJSON 库来解析 JSON 数据。以下是一个简单的示例代码,演示如何获取 JSON 对象中的所有键值:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "cJSON.h"
int main() {
char *json_str = "{\"name\":\"John\",\"age\":30,\"city\":\"New York\"}";
cJSON *json = cJSON_Parse(json_str);
if (json != NULL) {
cJSON *child = json->child;
while (child != NULL) {
printf("%s: ", child->string);
switch (child->type) {
case cJSON_String:
printf("%s\n", child->valuestring);
break;
case cJSON_Number:
printf("%d\n", child->valueint);
break;
case cJSON_NULL:
printf("null\n");
break;
default:
printf("Unknown type\n");
break;
}
child = child->next;
}
cJSON_Delete(json);
} else {
printf("Error parsing JSON string\n");
}
return 0;
}
```
在上面的代码中,我们首先将 JSON 字符串解析为 cJSON 对象,然后遍历其所有子节点,并根据节点类型输出相应的键和值。你可以将上面的代码复制到一个 C 语言源文件中,然后使用 cJSON 库进行编译和执行。
阅读全文
相关推荐
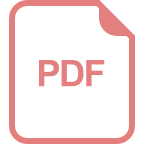

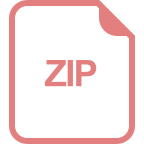
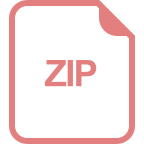
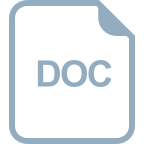

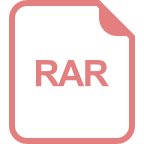
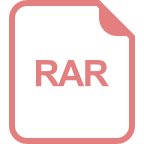
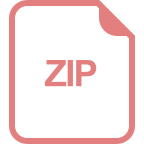
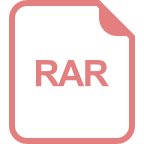
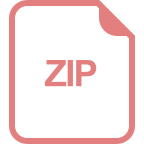
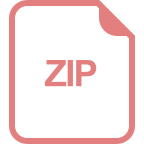
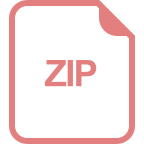
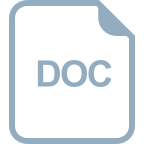
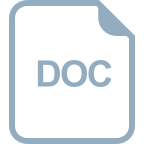
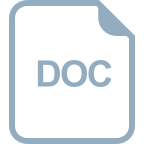
