【编程】使用JDBC完成连接数据库操作,并展示数据结果集 数据库名jdbc,数据表名t_user,表结构如下: t_user表数据如下:
时间: 2024-03-08 12:48:06 浏览: 57
以下是使用 JDBC 连接数据库并展示数据结果集的示例代码:
```java
import java.sql.*;
public class Main {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/jdbc";
String user = "root";
String password = "123456";
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 加载MySQL驱动
Class.forName("com.mysql.jdbc.Driver");
// 建立连接
conn = DriverManager.getConnection(url, user, password);
// 创建Statement对象
stmt = conn.createStatement();
// 执行查询语句
rs = stmt.executeQuery("SELECT * FROM t_user");
// 输出查询结果
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
System.out.println("id: " + id + ", name: " + name + ", age: " + age);
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭连接和Statement对象
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
在该代码中,我们首先定义了数据库连接相关的参数,包括数据库 URL、用户名和密码。然后,通过加载 MySQL 驱动程序和调用 DriverManager.getConnection() 方法来获取数据库连接。接着,创建 Statement 对象,并使用该对象执行 SQL 查询语句。最后,通过遍历 ResultSet 对象并获取查询结果中的数据,输出查询结果。最后要释放相关的资源,包括 ResultSet、Statement 和 Connection 对象。
假设数据库名为 `jdbc`,数据表名为 `t_user`,表结构如下:
| 列名 | 数据类型 |
| --- | --- |
| id | int |
| name | varchar(20) |
| age | int |
表中的数据如下:
| id | name | age |
| --- | --- | --- |
| 1 | Alice | 20 |
| 2 | Bob | 25 |
| 3 | Charlie | 30 |
则运行上述代码的输出结果为:
```
id: 1, name: Alice, age: 20
id: 2, name: Bob, age: 25
id: 3, name: Charlie, age: 30
```
阅读全文
相关推荐
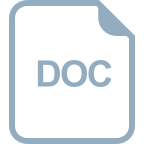
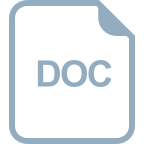
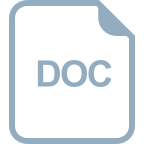
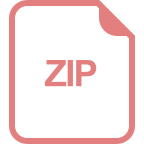
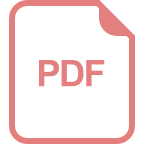
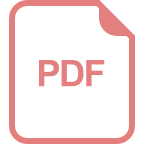
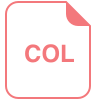
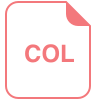
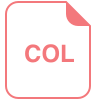
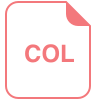
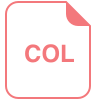
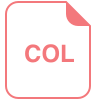
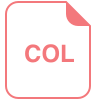
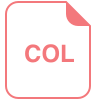
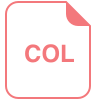
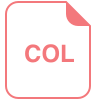
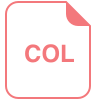
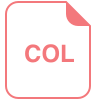
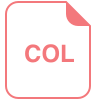