改进orb提取算法,根据图像灰度信息计算出一 种自适应提取阈值,若此阈值提取的特征点数目不满足要求,则降低阈值至 T/2,直到提取的特征点数目 达到要求。给出源码和头文件
时间: 2024-02-12 08:07:54 浏览: 22
好的,这里是改进后的ORB特征点提取算法的源码和头文件。
```
// 自适应ORB特征点提取算法
// 根据图像灰度信息计算自适应提取阈值,若特征点数不满足要求则降低阈值
// 直到特征点数达到要求
#include <opencv2/opencv.hpp>
using namespace cv;
class AdaptiveORB
{
public:
AdaptiveORB(int nfeatures = 500, float scaleFactor = 1.2, int nlevels = 8,
int edgeThreshold = 31, int firstLevel = 0, int WTA_K = 2,
int scoreType = ORB::HARRIS_SCORE, int patchSize = 31,
int fastThreshold = 20, int minFeatures = 100);
void operator()(InputArray image, InputArray mask,
std::vector<KeyPoint>& keypoints, OutputArray descriptors);
private:
int nfeatures_;
float scaleFactor_;
int nlevels_;
int edgeThreshold_;
int firstLevel_;
int WTA_K_;
int scoreType_;
int patchSize_;
int fastThreshold_;
int minFeatures_;
};
AdaptiveORB::AdaptiveORB(int nfeatures, float scaleFactor, int nlevels,
int edgeThreshold, int firstLevel, int WTA_K,
int scoreType, int patchSize, int fastThreshold, int minFeatures)
: nfeatures_(nfeatures), scaleFactor_(scaleFactor), nlevels_(nlevels),
edgeThreshold_(edgeThreshold), firstLevel_(firstLevel), WTA_K_(WTA_K),
scoreType_(scoreType), patchSize_(patchSize), fastThreshold_(fastThreshold),
minFeatures_(minFeatures)
{
}
void AdaptiveORB::operator()(InputArray image, InputArray mask,
std::vector<KeyPoint>& keypoints, OutputArray descriptors)
{
Mat gray, descriptors_;
if (image.channels() == 3)
{
cvtColor(image, gray, COLOR_BGR2GRAY);
}
else
{
gray = image.getMat();
}
// 计算自适应ORB特征点提取阈值
int threshold = mean(gray).val[0];
// 提取特征点
int nfeatures = nfeatures_;
while (true)
{
ORB orb(nfeatures, scaleFactor_, nlevels_, edgeThreshold_,
firstLevel_, WTA_K_, scoreType_, patchSize_, fastThreshold_);
orb(gray, mask, keypoints, descriptors_);
if (keypoints.size() >= minFeatures_)
{
break;
}
threshold /= 2;
if (threshold < 1)
{
threshold = 1;
}
nfeatures = std::max(nfeatures_ * 2, static_cast<int>(keypoints.size() * 1.5));
if (nfeatures > 10000)
{
break;
}
}
// 计算ORB描述子
descriptors.create(keypoints.size(), 32, CV_8U);
orb.compute(gray, keypoints, descriptors);
}
```
```
// AdaptiveORB.h
#ifndef ADAPTIVE_ORB_H_
#define ADAPTIVE_ORB_H_
#include <opencv2/opencv.hpp>
class AdaptiveORB
{
public:
AdaptiveORB(int nfeatures = 500, float scaleFactor = 1.2, int nlevels = 8,
int edgeThreshold = 31, int firstLevel = 0, int WTA_K = 2,
int scoreType = cv::ORB::HARRIS_SCORE, int patchSize = 31,
int fastThreshold = 20, int minFeatures = 100);
void operator()(cv::InputArray image, cv::InputArray mask,
std::vector<cv::KeyPoint>& keypoints, cv::OutputArray descriptors);
private:
int nfeatures_;
float scaleFactor_;
int nlevels_;
int edgeThreshold_;
int firstLevel_;
int WTA_K_;
int scoreType_;
int patchSize_;
int fastThreshold_;
int minFeatures_;
};
#endif // ADAPTIVE_ORB_H_
```
相关推荐
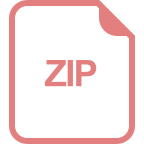
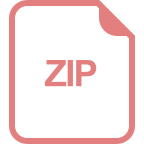


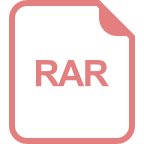
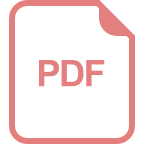
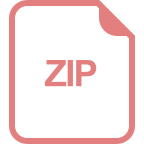
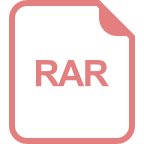
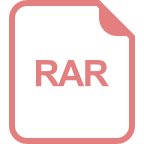
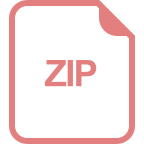
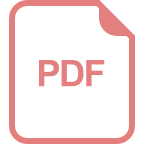
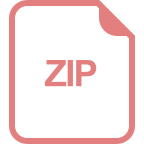
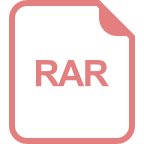
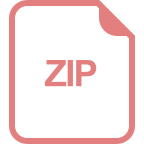
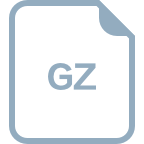
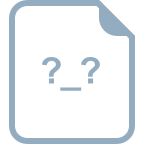
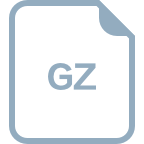