vue3子组件怎么像父组件传递一个方法
时间: 2023-09-18 16:06:34 浏览: 227
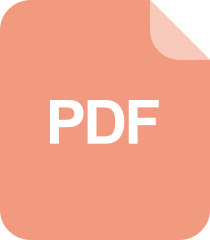
vue-prop父组件向子组件进行传值的方法
### 回答1:
在 Vue3 中,你可以使用 `props` 将方法传递给子组件。下面是一个示例:
首先,在父组件中定义一个方法,并将其作为 `props` 传递给子组件:
```vue
<template>
<div>
<child-component :handleClick="handleClick"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
methods: {
handleClick() {
console.log('Button clicked');
},
},
};
</script>
```
然后,在子组件中,你可以接收 `handleClick` 方法作为 `props`,并在需要的地方调用它:
```vue
<template>
<button @click="handleClick">Click me</button>
</template>
<script>
export default {
props: {
handleClick: Function,
},
};
</script>
```
现在,当用户点击子组件中的按钮时,将会调用父组件中的 `handleClick` 方法。
### 回答2:
在Vue3中,可以通过使用Props属性将父组件中的方法传递给子组件。
首先,确认父组件中的方法需要在子组件中传递。比如,父组件中有一个计算方法`calculate`:
```javascript
methods: {
calculate() {
// 进行计算
}
}
```
在子组件中,通过声明props属性来接收父组件传递的方法:
```javascript
props: {
calculateFunction: {
type: Function,
required: true
}
}
```
然后,在父组件中使用子组件时,通过将计算方法作为props属性的参数传递给子组件:
```html
<template>
<div>
<child-component :calculateFunction="calculate"></child-component>
</div>
</template>
```
最后,在子组件中即可使用传递而来的方法:
```javascript
methods: {
callParentMethod() {
this.calculateFunction(); // 调用父组件中的方法
}
}
```
这样,父组件中的方法`calculate`就能够通过props属性传递给子组件,并且在子组件中被调用了。
### 回答3:
在Vue 3中,子组件向父组件传递方法的常用方式是通过自定义事件。具体步骤如下:
1. 在父组件中定义一个方法,用于处理子组件传递过来的事件。例如,可以在父组件中定义一个名为`handleEvent`的方法。
2. 在父组件中使用子组件,并为子组件绑定一个自定义事件。通过`v-on`或`@`指令,将子组件触发的事件与父组件的方法关联起来,例如:
```html
<template>
<ChildComponent @customEvent="handleEvent"></ChildComponent>
</template>
```
3. 在子组件中,通过`$emit`方法触发自定义事件,并传递需要传递给父组件的参数。例如:
```js
methods: {
handleClick() {
this.$emit('customEvent', 'Hello from child component!');
}
}
```
在子组件的某个方法(例如点击事件)中,通过`$emit`方法触发名为`customEvent`的自定义事件,并传递了一个参数作为消息。
4. 父组件中的`handleEvent`方法会接收到子组件传递过来的参数,可以在该方法中对参数进行处理。例如:
```js
methods: {
handleEvent(data) {
console.log(data); // 输出:Hello from child component!
}
}
```
通过以上步骤,子组件就可以像父组件传递一个方法了。子组件中使用`$emit`触发自定义事件,父组件中使用`@`指令关联自定义事件与方法,实现了子组件向父组件传递方法的功能。
阅读全文
相关推荐
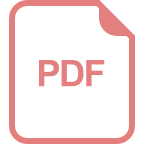
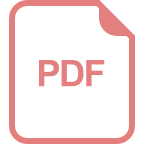
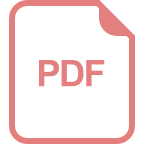
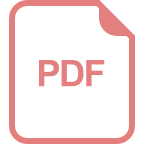
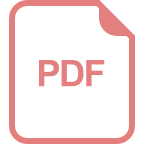
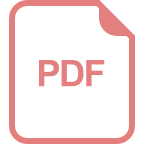
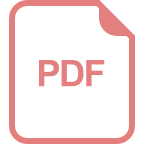
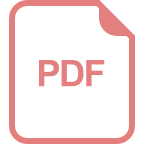
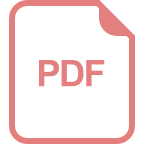
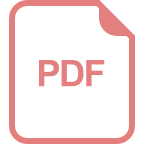
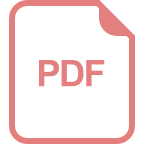
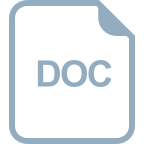
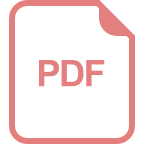
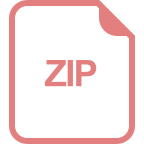