根据文件内容,编写软件代码
时间: 2024-11-27 09:11:17 浏览: 11
编写一个完整的在线教育课程管理系统的代码涉及多个模块和技术栈,这里我将为你提供一些关键模块的示例代码。我们将重点关注后端的核心功能模块,如课程管理、学生管理和教学资源管理。假设我们使用 Java 和 Spring Boot 框架,数据库使用 MySQL。
### 1. 项目初始化
首先,我们需要创建一个 Spring Boot 项目,并添加必要的依赖项。你可以使用 Spring Initializr 来生成初始项目结构。
#### `pom.xml` 文件
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
```
### 2. 实体类
#### `Course.java`
```java
package com.example.education.model;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Course {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String courseCode;
private String name;
private String description;
private String instructor;
private String type; // "live" or "recorded"
// Getters and Setters
}
```
#### `Student.java`
```java
package com.example.education.model;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String studentNumber;
private String firstName;
private String lastName;
private int age;
private String gender;
private String email;
// Getters and Setters
}
```
#### `Resource.java`
```java
package com.example.education.model;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Resource {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String resourceName;
private String resourceType; // "video", "document", etc.
private String uploadTime;
private String url;
// Getters and Setters
}
```
### 3. 数据访问对象 (DAO)
#### `CourseRepository.java`
```java
package com.example.education.repository;
import com.example.education.model.Course;
import org.springframework.data.jpa.repository.JpaRepository;
public interface CourseRepository extends JpaRepository<Course, Long> {
}
```
#### `StudentRepository.java`
```java
package com.example.education.repository;
import com.example.education.model.Student;
import org.springframework.data.jpa.repository.JpaRepository;
public interface StudentRepository extends JpaRepository<Student, Long> {
}
```
#### `ResourceRepository.java`
```java
package com.example.education.repository;
import com.example.education.model.Resource;
import org.springframework.data.jpa.repository.JpaRepository;
public interface ResourceRepository extends JpaRepository<Resource, Long> {
}
```
### 4. 服务层
#### `CourseService.java`
```java
package com.example.education.service;
import com.example.education.model.Course;
import com.example.education.repository.CourseRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class CourseService {
@Autowired
private CourseRepository courseRepository;
public List<Course> getAllCourses() {
return courseRepository.findAll();
}
public Course getCourseById(Long id) {
return courseRepository.findById(id).orElse(null);
}
public Course createCourse(Course course) {
return courseRepository.save(course);
}
public Course updateCourse(Long id, Course courseDetails) {
Course course = courseRepository.findById(id).orElse(null);
if (course != null) {
course.setCourseCode(courseDetails.getCourseCode());
course.setName(courseDetails.getName());
course.setDescription(courseDetails.getDescription());
course.setInstructor(courseDetails.getInstructor());
course.setType(courseDetails.getType());
return courseRepository.save(course);
}
return null;
}
public void deleteCourse(Long id) {
courseRepository.deleteById(id);
}
}
```
#### `StudentService.java`
```java
package com.example.education.service;
import com.example.education.model.Student;
import com.example.education.repository.StudentRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class StudentService {
@Autowired
private StudentRepository studentRepository;
public List<Student> getAllStudents() {
return studentRepository.findAll();
}
public Student getStudentById(Long id) {
return studentRepository.findById(id).orElse(null);
}
public Student createStudent(Student student) {
return studentRepository.save(student);
}
public Student updateStudent(Long id, Student studentDetails) {
Student student = studentRepository.findById(id).orElse(null);
if (student != null) {
student.setStudentNumber(studentDetails.getStudentNumber());
student.setFirstName(studentDetails.getFirstName());
student.setLastName(studentDetails.getLastName());
student.setAge(studentDetails.getAge());
student.setGender(studentDetails.getGender());
student.setEmail(studentDetails.getEmail());
return studentRepository.save(student);
}
return null;
}
public void deleteStudent(Long id) {
studentRepository.deleteById(id);
}
}
```
#### `ResourceService.java`
```java
package com.example.education.service;
import com.example.education.model.Resource;
import com.example.education.repository.ResourceRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class ResourceService {
@Autowired
private ResourceRepository resourceRepository;
public List<Resource> getAllResources() {
return resourceRepository.findAll();
}
public Resource getResourceById(Long id) {
return resourceRepository.findById(id).orElse(null);
}
public Resource createResource(Resource resource) {
return resourceRepository.save(resource);
}
public Resource updateResource(Long id, Resource resourceDetails) {
Resource resource = resourceRepository.findById(id).orElse(null);
if (resource != null) {
resource.setResourceName(resourceDetails.getResourceName());
resource.setResourceType(resourceDetails.getResourceType());
resource.setUploadTime(resourceDetails.getUploadTime());
resource.setUrl(resourceDetails.getUrl());
return resourceRepository.save(resource);
}
return null;
}
public void deleteResource(Long id) {
resourceRepository.deleteById(id);
}
}
```
### 5. 控制器
#### `CourseController.java`
```java
package com.example.education.controller;
import com.example.education.model.Course;
import com.example.education.service.CourseService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api/courses")
public class CourseController {
@Autowired
private CourseService courseService;
@GetMapping
public List<Course> getAllCourses() {
return courseService.getAllCourses();
}
@GetMapping("/{id}")
public Course getCourseById(@PathVariable Long id) {
return courseService.getCourseById(id);
}
@PostMapping
public Course createCourse(@RequestBody Course course) {
return courseService.createCourse(course);
}
@PutMapping("/{id}")
public Course updateCourse(@PathVariable Long id, @RequestBody Course courseDetails) {
return courseService.updateCourse(id, courseDetails);
}
@DeleteMapping("/{id}")
public void deleteCourse(@PathVariable Long id) {
courseService.deleteCourse(id);
}
}
```
#### `StudentController.java`
```java
package com.example.education.controller;
import com.example.education.model.Student;
import com.example.education.service.StudentService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api/students")
public class StudentController {
@Autowired
private StudentService studentService;
@GetMapping
public List<Student> getAllStudents() {
return studentService.getAllStudents();
}
@GetMapping("/{id}")
public Student getStudentById(@PathVariable Long id) {
return studentService.getStudentById(id);
}
@PostMapping
public Student createStudent(@RequestBody Student student) {
return studentService.createStudent(student);
}
@PutMapping("/{id}")
public Student updateStudent(@PathVariable Long id, @RequestBody Student studentDetails) {
return studentService.updateStudent(id, studentDetails);
}
@DeleteMapping("/{id}")
public void deleteStudent(@PathVariable Long id) {
studentService.deleteStudent(id);
}
}
```
#### `ResourceController.java`
```java
package com.example.education.controller;
import com.example.education.model.Resource;
import com.example.education.service.ResourceService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api/resources")
public class ResourceController {
@Autowired
private ResourceService resourceService;
@GetMapping
public List<Resource> getAllResources() {
return resourceService.getAllResources();
}
@GetMapping("/{id}")
public Resource getResourceById(@PathVariable Long id) {
return resourceService.getResourceById(id);
}
@PostMapping
public Resource createResource(@RequestBody Resource resource) {
return resourceService.createResource(resource);
}
@PutMapping("/{id}")
public Resource updateResource(@PathVariable Long id, @RequestBody Resource resourceDetails) {
return resourceService.updateResource(id, resourceDetails);
}
@DeleteMapping("/{id}")
public void deleteResource(@PathVariable Long id) {
resourceService.deleteResource(id);
}
}
```
### 6. 配置文件
#### `application.properties`
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/education_db
spring.datasource.username=root
spring.datasource.password=your_password
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5Dialect
```
### 7. 主启动类
#### `EducationApplication.java`
```java
package com.example.education;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class EducationApplication {
public static void main(String[] args) {
SpringApplication.run(EducationApplication.class, args);
}
}
```
以上代码展示了如何使用 Spring Boot 构建一个简单的在线教育课程管理系统的核心功能模块。你可以在此基础上继续扩展和完善其他功能,如教学互动、数据分析等。希望这对你有所帮助!
阅读全文
相关推荐


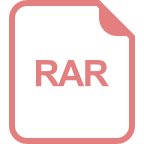




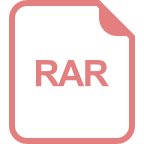

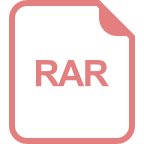
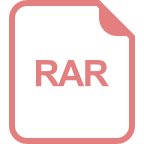
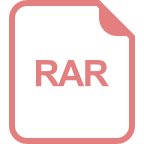





