go用switch判断某一天工作日
时间: 2024-09-22 13:04:03 浏览: 35
在Go语言中,你可以使用`switch`语句结合日期处理包`time`来判断某个日期是否为工作日。工作日通常是指周一到周五。首先,你需要获取当前日期并判断其星期几:
```go
package main
import (
"fmt"
"time"
)
func isWorkDay(date time.Time) bool {
weekday := date.Weekday()
return weekday >= time.Monday && weekday <= time.Friday // Monday = 0, Sunday = 6 in Go's time package
}
func main() {
today := time.Now()
switchResult := isWorkDay(today)
fmt.Println("今天是工作日吗?", switchResult)
}
```
在这个例子中,`isWorkDay`函数接收一个`time.Time`类型的参数,通过`Weekday()`方法获取该日期是一周中的哪一天,并返回布尔值表示是不是工作日。
相关问题
java使用switch判断某年某月某日是一年中的第几天
可以使用以下代码来判断某年某月某日是一年中的第几天:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入年份:");
int year = scanner.nextInt();
System.out.print("请输入月份:");
int month = scanner.nextInt();
System.out.print("请输入日期:");
int day = scanner.nextInt();
int days = ;
switch (month) {
case 12:
days += 30;
case 11:
days += 31;
case 10:
days += 30;
case 9:
days += 31;
case 8:
days += 31;
case 7:
days += 30;
case 6:
days += 31;
case 5:
days += 30;
case 4:
days += 31;
case 3:
if ((year % 4 == && year % 100 != ) || year % 400 == ) {
days += 29;
} else {
days += 28;
}
case 2:
days += 31;
case 1:
days += day;
}
System.out.println(year + "年" + month + "月" + day + "日是" + days + "年的第" + days + "天。");
}
}
```
注意,这里使用了 switch 语句来判断每个月的天数,需要注意 case 语句的顺序。同时,需要考虑闰年的情况。
go 用switch语句实现输入某年某月某日,判断这一天是这一年的第几天
Go语言中,你可以使用`switch`语句结合简单的数学计算来实现这个功能。首先,你需要获取当前年份、月份和日期,然后根据不同月份的天数差异来判断。以下是一个基本的例子:
```go
package main
import "fmt"
func daysInMonth(year int, month int) int {
switch month {
case 1, 3, 5, 7, 8, 10, 12:
return 31
case 4, 6, 9, 11:
return 30
// 特殊情况,闰年二月有29天
case 2:
if isLeapYear(year) {
return 29
} else {
return 28
}
default:
fmt.Println("无效的月份")
return -1
}
}
// 判断是否为闰年
func isLeapYear(year int) bool {
if year % 4 != 0 {
return false
}
if year % 100 != 0 {
return true
}
if year % 400 != 0 {
return false
}
return true
}
func main() {
var input string
fmt.Print("请输入年份(YYYY)、月份(MM)和日期(DD): ")
_, monthStr, dayStr := input.Split(" ")
year, _ := strconv.Atoi(monthStr[0:4])
month, _ := strconv.Atoi(monthStr[4:])
day, _ := strconv.Atoi(dayStr)
if day <= 0 || day > daysInMonth(year, month) {
fmt.Printf("%d/%02d/%d 不是有效的一天", year, month, day)
} else {
fmt.Printf("%d/%02d/%d 是当年的第%d天", year, month, day, day)
}
}
//
阅读全文
相关推荐
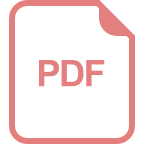
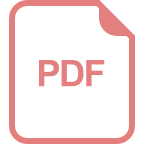
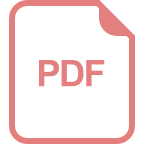













