请编写程序,对一段英文文本,统计其中所有不同单词的个数,以及词频最大的前10%的单词。 所谓“单词”,是指由不超过80个单词字符组成的连续字符串,但长度超过15的单词将只截取保留前15个单词字符。而合法的“单词字符”为大小写字母、数字和下划线,其它字符均认为是单词分隔符。
时间: 2023-05-31 22:18:06 浏览: 359
### 回答1:
请使用以下程序实现所需功能:
1. 将文本中的所有字符读入程序中
2. 使用正则表达式(regex)从文本中提取出所有单词
3. 对每个单词进行如下处理:
a. 如果长度大于15,截取前15个字符
b. 计数器+1,表示单词出现次数
4. 对单词数量进行排序,统计词频最高的10%的单词。
5. 输出不同单词的个数和词频最高的前10%的单词。
### 回答2:
为了实现对文本的单词统计,我们可以分以下几个步骤来实现:
1. 读取文本文件并将其转换为字符串
可以使用Python中的open()函数打开文本文件,利用read()函数读取其中的内容作为字符串,如下所示:
```python
file = open('file.txt', 'r')
text = file.read()
file.close()
```
2. 将字符串中的特殊字符替换为单词分隔符
由于题目中规定了单词分隔符包括除大小写字母、数字和下划线之外的所有字符,我们可以通过正则表达式将这些字符替换为空格,如下所示:
```python
import re
text = re.sub('[^A-Za-z0-9_]', ' ', text)
```
3. 统计单词出现次数
为了统计单词出现的次数,我们可以将字符串先按照空格分割为单词列表,再利用Python中的字典进行统计。代码如下:
```python
words = text.split()
word_dict = {}
for word in words:
if len(word) > 15:
word = word[:15]
if word in word_dict:
word_dict[word] += 1
else:
word_dict[word] = 1
```
4. 统计单词个数并选出词频最大的前10%
为了统计单词个数,我们可以直接对字典中的键值对数量进行统计。为了选出词频最大的前10%,可以利用Python中的sorted()函数和切片操作实现,代码如下:
```python
word_num = len(word_dict)
sorted_words = sorted(word_dict.items(), key=lambda x: x[1], reverse=True)
top10_percent = sorted_words[:int(word_num*0.1)]
```
上述代码中,sorted_words是一个按照单词出现次数排序的元组列表,top10_percent则是其中排名前10%的元素。
最终,我们可以将上述步骤整合到一个程序中,如下所示:
```python
import re
# 读取文本文件并将其转换为字符串
file = open('file.txt', 'r')
text = file.read()
file.close()
# 将字符串中的特殊字符替换为单词分隔符
text = re.sub('[^A-Za-z0-9_]', ' ', text)
# 统计单词出现次数
words = text.split()
word_dict = {}
for word in words:
if len(word) > 15:
word = word[:15]
if word in word_dict:
word_dict[word] += 1
else:
word_dict[word] = 1
# 统计单词个数并选出词频最大的前10%
word_num = len(word_dict)
sorted_words = sorted(word_dict.items(), key=lambda x: x[1], reverse=True)
top10_percent = sorted_words[:int(word_num*0.1)]
# 输出结果
word_num_str = '单词总数:{}'.format(word_num)
top10_percent_str = '词频最大的前10%单词如下:\n'
for item in top10_percent:
top10_percent_str += '{}: {}\n'.format(item[0], item[1])
print(word_num_str)
print(top10_percent_str)
```
这样,我们就能够方便地对一个英文文本进行单词统计和词频分析了。
### 回答3:
题目要求编写程序,对一段英文文本进行统计,分别统计其单词总数和词频最大的前10%的单词,下面给出具体思路:
1. 将文本按照单词分割,将每一个单词存入一个字典中,并记录每个单词出现的次数。
2. 对字典中的单词进行排序,按照出现次数从大到小排列。
3. 取出排序后的前10%的单词,即出现次数最多的前10%的单词。
4. 统计单词总数,并将结果输出。
下面给出具体代码实现:
```
import re
# 定义变量
word_dict = {}
word_list = []
text = ''
total_words = 0
# 读取文本文件
with open('text.txt', 'r') as f:
text = f.read()
# 正则表达式匹配单词
pattern = re.compile(r'\b\w{1,80}\b')
match = pattern.findall(text)
# 统计单词出现次数
for word in match:
# 截取单词前15个字符
if len(word) > 15:
word = word[:15]
# 判断单词是否已在字典中
if word not in word_dict:
word_dict[word] = 1
else:
word_dict[word] += 1
# 统计单词总数
for value in word_dict.values():
total_words += value
# 对单词按照出现次数从大到小排序
word_list = sorted(word_dict.items(), key=lambda x: x[1], reverse=True)
# 取出出现次数最多的前10%的单词
top_words = word_list[:int(len(word_list)*0.1)]
# 输出结果
print('单词总数:', total_words)
print('词频最大的前10%的单词:')
for word in top_words:
print(word[0], word[1])
```
以上是一个简单的Python程序,能够完成所述任务,但是还有许多优化的空间。比如,在统计单词出现次数时,可以使用Python的Counter类。在截取单词前15个字符时,可以使用Python的字符串切片操作。在读取文件时,可以考虑使用with open语句。在输出结果时,可以使用Python的格式化字符串功能。等等。
阅读全文
相关推荐







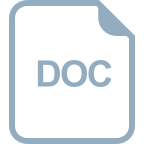
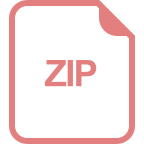





