public static MediaPlayer create(Context context, Uri uri, SurfaceHolder holder, AudioAttributes audioAttributes, int audioSessionId) { try { MediaPlayer mp = new MediaPlayer(audioSessionId); final AudioAttributes aa = audioAttributes != null ? audioAttributes : new AudioAttributes.Builder().build(); mp.setAudioAttributes(aa); //设置音频属性 mp.native_setAudioSessionId(audioSessionId); //设置声音的会话Id,视频和音频分开渲染 mp.setDataSource(context, uri); //如果 holder 不为 null,则将其设置为 MediaPlayer 的显示界面 if (holder != null) { mp.setDisplay(holder); } mp.prepare(); return mp; } catch (IOException ex) { Log.d(TAG, "create failed:", ex); // fall through } catch (IllegalArgumentException ex) { Log.d(TAG, "create failed:", ex); // fall through } catch (SecurityException ex) { Log.d(TAG, "create failed:", ex); // fall through } return null; }
时间: 2024-02-10 13:34:11 浏览: 162
这是一段代码,看起来是创建一个 MediaPlayer 对象的静态方法。它接受一些参数,包括上下文、音频和视频的 URI、SurfaceHolder 对象、AudioAttributes 对象和音频会话 ID。在方法内部,它使用这些参数来设置 MediaPlayer 的音频属性、声音会话 ID 和显示界面,并最终返回一个准备好的 MediaPlayer 对象。如果在创建过程中出现了异常,该方法将返回 null。
相关问题
public static MediaPlayer create(Context context, int resid, AudioAttributes audioAttributes, int audioSessionId) { try { AssetFileDescriptor afd = context.getResources().openRawResourceFd(resid); if (afd == null) return null; MediaPlayer mp = new MediaPlayer(audioSessionId); final AudioAttributes aa = audioAttributes != null ? audioAttributes : new AudioAttributes.Builder().build(); mp.setAudioAttributes(aa); mp.native_setAudioSessionId(audioSessionId); mp.setDataSource(afd.getFileDescriptor(), afd.getStartOffset(), afd.getLength()); afd.close(); mp.prepare(); return mp; } catch (IOException ex) { Log.d(TAG, "create failed:", ex); // fall through } catch (IllegalArgumentException ex) { Log.d(TAG, "create failed:", ex); // fall through } catch (SecurityException ex) { Log.d(TAG, "create failed:", ex); // fall through } return null; }
这是另一个创建 MediaPlayer 对象的静态方法,它接受一个资源 ID、AudioAttributes 对象和音频会话 ID 作为参数。在方法内部,它使用上下文和资源 ID 来获取 AssetFileDescriptor 对象,并使用该对象设置 MediaPlayer 的数据源。然后,它设置音频属性、声音会话 ID,并准备 MediaPlayer 对象。如果在创建过程中出现了异常,该方法将返回 null。
Android mediaplayer的create函数参数详解
Android MediaPlayer类中的create()函数用于创建一个新的MediaPlayer对象,其参数如下:
1. Context:传递应用程序的上下文,通常为Activity对象。
2. Uri:要播放的媒体文件的URI。可以是本地文件路径的URI,也可以是网络上的文件的URI。
3. SurfaceHolder:如果要在SurfaceView中播放视频,则需要传递SurfaceHolder对象。
4. AudioAttributes:用于指定音频播放属性,例如音量和音频焦点。
5. OnPreparedListener:当MediaPlayer对象准备好播放媒体时,将调用此接口的 onPrepared() 回调函数。
6. OnCompletionListener:当MediaPlayer对象播放媒体完成时,将调用此接口的 onCompletion() 回调函数。
7. OnErrorListener:当MediaPlayer对象发生错误时,将调用此接口的 onError() 回调函数。
注意:以上参数中只有 Uri 是必需的,其他参数可以为 null。
阅读全文
相关推荐





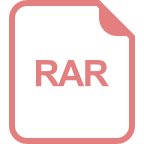



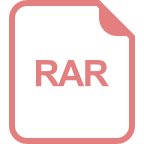




