3) Create a main.c file. Test BST code by building the following BST. a) Insert 3, 1, 4, 6, 9, 2, 5, 7,6,10 into an Empty tree.
时间: 2024-05-15 08:18:09 浏览: 81
Sure, I understand your question. To test the BST code, we can create a main.c file and write a code to insert the given elements into an empty tree. Here's an example code for the same:
```
#include <stdio.h>
#include <stdlib.h>
// Definition of Node for BST
struct node {
int data;
struct node *left, *right;
};
// Function to create a new node
struct node *newNode(int item) {
struct node *temp = (struct node *)malloc(sizeof(struct node));
temp->data = item;
temp->left = temp->right = NULL;
return temp;
}
// Function to insert a new node
struct node* insert(struct node* node, int data) {
// If the tree is empty, return a new node
if (node == NULL) return newNode(data);
// Otherwise, recur down the tree
if (data < node->data)
node->left = insert(node->left, data);
else if (data > node->data)
node->right = insert(node->right, data);
// return the (unchanged) node pointer
return node;
}
// Function to do inorder traversal of BST
void inorder(struct node *root) {
if (root != NULL) {
inorder(root->left);
printf("%d ", root->data);
inorder(root->right);
}
}
// Main Function
int main() {
struct node *root = NULL;
int arr[] = {3, 1, 4, 6, 9, 2, 5, 7, 6, 10};
int n = sizeof(arr)/sizeof(arr[0]);
for(int i=0; i<n; i++) {
root = insert(root, arr[i]);
}
inorder(root);
return 0;
}
```
In the above code, we create a new node using 'newNode' function, and then, traverse through the tree using the 'insert' function to insert each element. We then do an inorder traversal of the BST using 'inorder' function to print the sorted order of the elements.
阅读全文
相关推荐
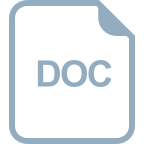
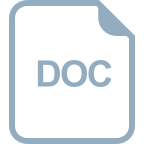
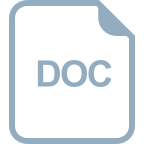
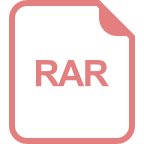
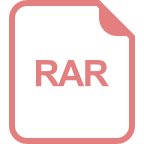
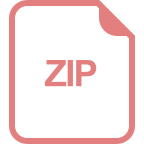
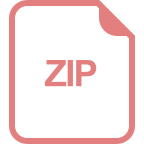
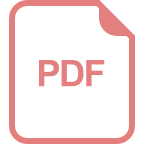
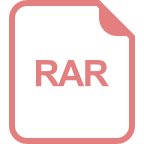
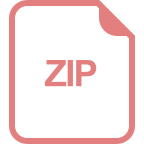
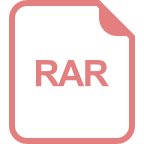
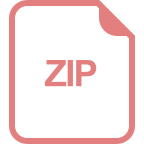
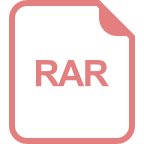
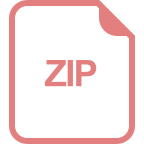
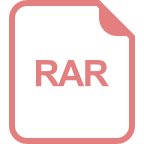
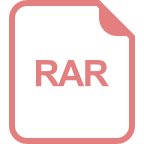
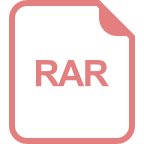