python实现一个简单的通讯录管理,包括添加、删除、查询、保存等功能。 应用到的知识有:类、字典、文件操作
时间: 2023-10-20 08:14:04 浏览: 155
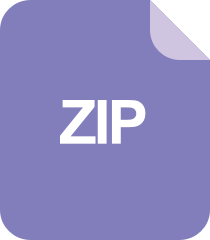
基于C++语言和单链表数据结构实现的通讯录管理系统,包含文件读取、文件保存、增删改查、排序、清空等功能.zip

好的,这里提供一个简单的通讯录管理程序实现。
1. 创建一个Contact类,包含姓名和电话两个属性,以及添加、删除、查询联系人等方法。
```python
class Contact:
def __init__(self, name, phone):
self.name = name
self.phone = phone
def __str__(self):
return f'{self.name}\t{self.phone}'
class ContactList:
def __init__(self):
self.contacts = {}
def add_contact(self, name, phone):
contact = Contact(name, phone)
self.contacts[name] = contact
def remove_contact(self, name):
if name in self.contacts:
del self.contacts[name]
else:
print(f'{name} not found in contacts')
def search_contact(self, name):
if name in self.contacts:
return str(self.contacts[name])
else:
print(f'{name} not found in contacts')
```
2. 创建一个函数用于保存通讯录,将通讯录数据保存至文件中。
```python
def save_contacts(filename, contacts):
with open(filename, 'w') as f:
for name, contact in contacts.items():
f.write(f'{name},{contact.phone}\n')
```
3. 创建一个函数用于读取通讯录文件,将数据加载到字典中。
```python
def load_contacts(filename):
contacts = {}
try:
with open(filename, 'r') as f:
for line in f:
name, phone = line.strip().split(',')
contacts[name] = Contact(name, phone)
except FileNotFoundError:
pass
return contacts
```
4. 创建一个主函数用于交互式操作通讯录,包括添加、删除、查询、保存等功能。
```python
def main():
contacts = load_contacts('contacts.txt')
contact_list = ContactList()
for name, contact in contacts.items():
contact_list.add_contact(name, contact.phone)
while True:
print('1. Add contact')
print('2. Remove contact')
print('3. Search contact')
print('4. Save contacts')
print('5. Exit')
choice = input('Enter your choice: ')
if choice == '1':
name = input('Enter name: ')
phone = input('Enter phone: ')
contact_list.add_contact(name, phone)
elif choice == '2':
name = input('Enter name: ')
contact_list.remove_contact(name)
elif choice == '3':
name = input('Enter name: ')
print(contact_list.search_contact(name))
elif choice == '4':
save_contacts('contacts.txt', contact_list.contacts)
elif choice == '5':
break
else:
print('Invalid choice')
```
5. 在程序中调用主函数以运行程序。
```python
if __name__ == '__main__':
main()
```
以上就是一个简单的通讯录管理程序的实现,包括添加、删除、查询、保存等功能,涉及到类、字典、文件操作等知识点。
阅读全文
相关推荐
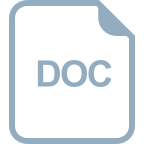
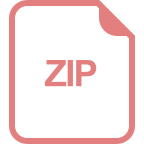
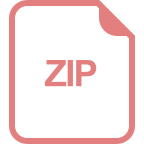
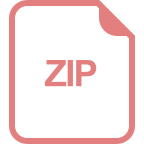
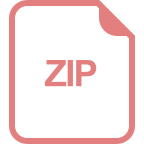
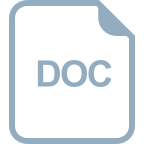
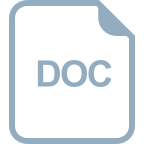
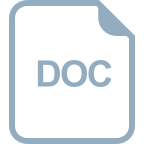
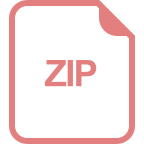
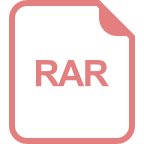
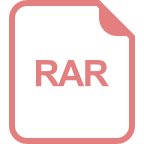
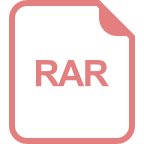
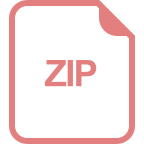
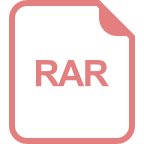
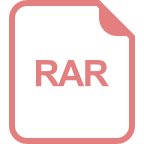
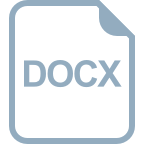
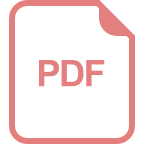