Qcustomplot实现图表联动,并列出代码
时间: 2023-08-24 07:04:51 浏览: 128
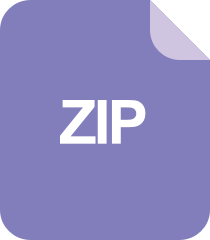
包含qcustomplot的示例代码
QCustomPlot是一个非常方便的开源C++库,可以用于绘制各种类型的图表,包括直方图、折线图、散点图等等。下面是QCustomPlot实现图表联动的示例代码:
```cpp
#include <QApplication>
#include <QMainWindow>
#include "qcustomplot.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
// 创建主窗口
QMainWindow mainWindow;
// 创建第一个图表
QCustomPlot plot1(&mainWindow);
plot1.setGeometry(0, 0, 400, 300);
plot1.addGraph();
QVector<double> x1(101), y1(101);
for (int i=0; i<101; i++)
{
x1[i] = i/50.0 - 1;
y1[i] = x1[i]*x1[i];
}
plot1.graph(0)->setData(x1, y1);
plot1.replot();
// 创建第二个图表
QCustomPlot plot2(&mainWindow);
plot2.setGeometry(0, 310, 400, 300);
plot2.addGraph();
QVector<double> x2(101), y2(101);
for (int i=0; i<101; i++)
{
x2[i] = i/50.0 - 1;
y2[i] = sin(x2[i]*3.14*2);
}
plot2.graph(0)->setData(x2, y2);
plot2.replot();
// 创建联动器
QCPRange range1 = plot1.graph(0)->data()->boundingRect();
QCPRange range2 = plot2.graph(0)->data()->boundingRect();
QCPRange totalRange(range1.lower, range2.upper);
QCPSelectionDecorator decorator;
QCPSelectionDecorator::setFromSelectable(plot1.graph(0), decorator);
QCPSelectionDecorator::setFromSelectable(plot2.graph(0), decorator);
QCPSelectionDecorator::setSelectable(plot1.graph(0), true);
QCPSelectionDecorator::setSelectable(plot2.graph(0), true);
QCPRangeDrag dragX(&plot2.xAxis);
QCPRangeDrag dragY(&plot2.yAxis);
dragX.setOrientation(Qt::Horizontal);
dragY.setOrientation(Qt::Vertical);
dragX.setRange(totalRange);
dragY.setRange(totalRange);
dragX.setSelectionDecorator(&decorator);
dragY.setSelectionDecorator(&decorator);
QCPRangeZoom zoomX(&plot2.xAxis);
QCPRangeZoom zoomY(&plot2.yAxis);
zoomX.setOrientation(Qt::Horizontal);
zoomY.setOrientation(Qt::Vertical);
zoomX.setRange(totalRange);
zoomY.setRange(totalRange);
zoomX.setSelectionDecorator(&decorator);
zoomY.setSelectionDecorator(&decorator);
plot1.setInteractions(QCP::iRangeDrag | QCP::iRangeZoom);
plot2.setInteractions(QCP::iRangeDrag | QCP::iRangeZoom);
plot2.xAxis->setRange(range1);
plot2.yAxis->setRange(range2);
// 显示窗口
mainWindow.setCentralWidget(&plot1);
mainWindow.setGeometry(100, 100, 400, 610);
mainWindow.show();
return a.exec();
}
```
这个示例程序中,我们创建了两个图表并将它们放在主窗口中。然后,我们创建了一个联动器,用于将两个图表关联起来。联动器包括一个QCPRangeDrag对象和一个QCPRangeZoom对象,用于实现拖动和缩放功能。我们还设置了选择装饰器,以便在选择时高亮显示数据。最后,我们将两个图表的交互模式设置为iRangeDrag和iRangeZoom,以便用户可以拖动和缩放它们。
运行这个程序,您将看到两个图表。您可以使用鼠标拖动或缩放任何一个图表,另一个图表也会相应地更新。这就是QCustomPlot实现图表联动的方法。
阅读全文
相关推荐
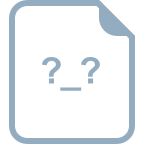
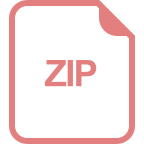




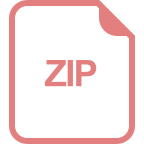
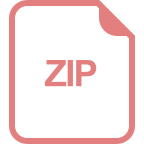
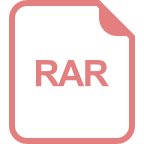
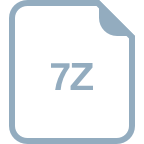
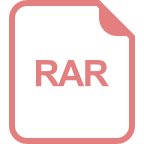
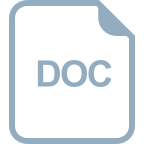
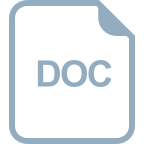
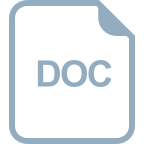
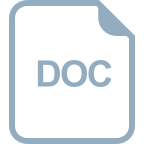
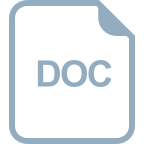
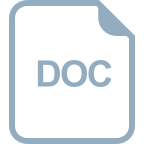
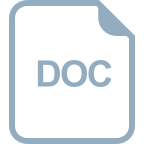