用python写一个DDPG算法案例并且注释
时间: 2024-03-15 17:45:18 浏览: 136
好的,这里给出一个使用Python实现DDPG算法的简单示例,并加上注释说明每个步骤的作用。
首先,我们需要导入需要的库,包括numpy、tensorflow等等:
```python
import tensorflow as tf
import numpy as np
import gym
from collections import deque
import random
```
然后,我们需要定义DDPG算法的参数和神经网络模型的结构。这里我们使用了一个简单的全连接神经网络:
```python
# DDPG算法的参数
state_dim = 3
action_dim = 1
gamma = 0.99
tau = 0.01
buffer_size = 1000000
batch_size = 64
# 定义Actor神经网络
actor_inputs = tf.keras.layers.Input(shape=(state_dim,))
actor_layer1 = tf.keras.layers.Dense(64, activation='relu')(actor_inputs)
actor_layer2 = tf.keras.layers.Dense(32, activation='relu')(actor_layer1)
actor_outputs = tf.keras.layers.Dense(action_dim, activation='tanh')(actor_layer2)
actor_model = tf.keras.models.Model(inputs=actor_inputs, outputs=actor_outputs)
# 定义Critic神经网络
critic_inputs = tf.keras.layers.Input(shape=(state_dim+action_dim,))
critic_layer1 = tf.keras.layers.Dense(64, activation='relu')(critic_inputs)
critic_layer2 = tf.keras.layers.Dense(32, activation='relu')(critic_layer1)
critic_outputs = tf.keras.layers.Dense(1, activation=None)(critic_layer2)
critic_model = tf.keras.models.Model(inputs=critic_inputs, outputs=critic_outputs)
```
接下来,我们需要定义经验回放缓存区的类,用来存储agent的经验,并从中随机抽样进行训练:
```python
# 定义经验回放缓存区类
class ReplayBuffer:
def __init__(self, buffer_size):
self.buffer = deque(maxlen=buffer_size)
def add(self, state, action, reward, next_state, done):
self.buffer.append((state, action, reward, next_state, done))
def sample(self, batch_size):
states, actions, rewards, next_states, dones = [], [], [], [], []
batch = random.sample(self.buffer, batch_size)
for experience in batch:
state, action, reward, next_state, done = experience
states.append(state)
actions.append(action)
rewards.append(reward)
next_states.append(next_state)
dones.append(done)
return states, actions, rewards, next_states, dones
```
然后,我们需要定义一个agent类,用来实现DDPG算法中的Actor和Critic的更新和训练:
```python
# 定义agent类
class DDPGAgent:
def __init__(self, state_dim, action_dim, buffer_size, batch_size, gamma, tau):
self.state_dim = state_dim
self.action_dim = action_dim
self.buffer = ReplayBuffer(buffer_size)
self.batch_size = batch_size
self.gamma = gamma
self.tau = tau
# 定义Actor和Critic神经网络
self.actor_model = self.build_actor_model()
self.target_actor_model = self.build_actor_model()
self.critic_model = self.build_critic_model()
self.target_critic_model = self.build_critic_model()
# 初始化目标网络的权重和偏置
self.update_target_networks(tau=1)
# 定义Actor神经网络
def build_actor_model(self):
inputs = tf.keras.layers.Input(shape=(self.state_dim,))
layer1 = tf.keras.layers.Dense(64, activation='relu')(inputs)
layer2 = tf.keras.layers.Dense(32, activation='relu')(layer1)
outputs = tf.keras.layers.Dense(self.action_dim, activation='tanh')(layer2)
model = tf.keras.models.Model(inputs=inputs, outputs=outputs)
return model
# 定义Critic神经网络
def build_critic_model(self):
inputs = tf.keras.layers.Input(shape=(self.state_dim+self.action_dim,))
layer1 = tf.keras.layers.Dense(64, activation='relu')(inputs)
layer2 = tf.keras.layers.Dense(32, activation='relu')(layer1)
outputs = tf.keras.layers.Dense(1, activation=None)(layer2)
model = tf.keras.models.Model(inputs=inputs, outputs=outputs)
return model
# 更新目标网络的权重和偏置
def update_target_networks(self, tau):
actor_weights = self.actor_model.get_weights()
target_actor_weights = self.target_actor_model.get_weights()
for i in range(len(actor_weights)):
target_actor_weights[i] = tau * actor_weights[i] + (1 - tau) * target_actor_weights[i]
self.target_actor_model.set_weights(target_actor_weights)
critic_weights = self.critic_model.get_weights()
target_critic_weights = self.target_critic_model.get_weights()
for i in range(len(critic_weights)):
target_critic_weights[i] = tau * critic_weights[i] + (1 - tau) * target_critic_weights[i]
self.target_critic_model.set_weights(target_critic_weights)
# Actor根据状态生成动作
def act(self, state):
action = self.actor_model.predict(np.array(state).reshape(1, self.state_dim))[0]
return action
# Critic根据状态和动作评估Q值
def critic_predict(self, state, action):
q_value = self.critic_model.predict(np.array([state + [action]]))[0][0]
return q_value
# 将经验添加到缓存区
def add_experience(self, state, action, reward, next_state, done):
self.buffer.add(state, action, reward, next_state, done)
# 从缓存区中随机抽样并训练Actor和Critic
def train(self):
states, actions, rewards, next_states, dones = self.buffer.sample(self.batch_size)
next_actions = self.target_actor_model.predict_on_batch(np.array(next_states))
next_qs = self.target_critic_model.predict_on_batch(np.array(next_states + next_actions))
targets = []
for i in range(self.batch_size):
if dones[i]:
targets.append(rewards[i])
else:
targets.append(rewards[i] + self.gamma * next_qs[i][0])
self.critic_model.train_on_batch(np.array(states + actions), np.array(targets))
with tf.GradientTape() as tape:
actions_pred = self.actor_model(np.array(states))
critic_pred = self.critic_model(np.concatenate((np.array(states), actions_pred), axis=1))
actor_loss = -tf.math.reduce_mean(critic_pred)
actor_grads = tape.gradient(actor_loss, self.actor_model.trainable_variables)
self.actor_model.optimizer.apply_gradients(zip(actor_grads, self.actor_model.trainable_variables))
self.update_target_networks(self.tau)
```
最后,我们需要定义一个训练过程,用来训练我们的DDPG算法:
```python
# 定义训练函数
def train_ddpg(env, agent, episodes):
for episode in range(episodes):
state = env.reset()
score = 0
done = False
while not done:
action = agent.act(state)
next_state, reward, done, _ = env.step(action)
agent.add_experience(state, action, reward, next_state, done)
if len(agent.buffer.buffer) > agent.batch_size:
agent.train()
state = next_state
score += reward
print("Episode:", episode, "Score:", score)
```
现在我们可以使用上面定义的函数来训练我们的DDPG算法了:
```python
env = gym.make('Pendulum-v0')
agent = DDPGAgent(state_dim, action_dim, buffer_size, batch_size, gamma, tau)
train_ddpg(env, agent, episodes=100)
```
这就是一个简单的DDPG算法的实现,希望对你有帮助!
阅读全文
相关推荐
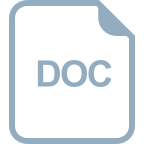
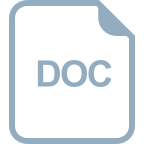
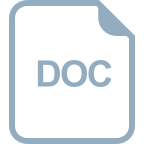

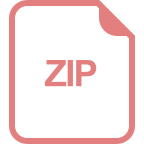

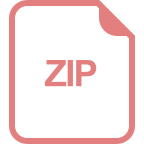
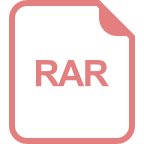
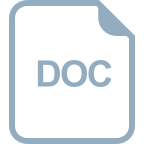


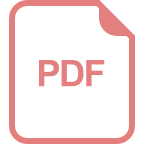
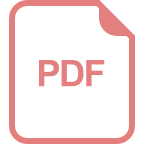
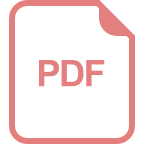
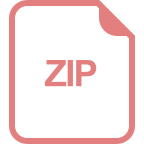
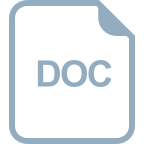
