我想要使用pytorch,vgg16,有使用預訓練,gpu訓練,實現two stream early fusion
时间: 2024-02-24 11:55:56 浏览: 28
好的,您想使用 PyTorch 框架,使用 VGG16 模型,并使用预训练模型进行训练。您还想要使用 GPU 进行训练,并实现 two stream early fusion。这些都是可行的,下面是大致的步骤:
1.安装 PyTorch 框架并加载 VGG16 模型:
```python
import torch
import torchvision.models as models
# 加载 VGG16 模型
vgg16 = models.vgg16(pretrained=True)
```
2.将模型放到 GPU 上进行训练:
```python
# 将模型放到 GPU 上
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
vgg16.to(device)
# 声明优化器和损失函数
optimizer = torch.optim.SGD(vgg16.parameters(), lr=0.001, momentum=0.9)
criterion = torch.nn.CrossEntropyLoss()
# 训练模型
for epoch in range(num_epochs):
for i, (inputs, labels) in enumerate(train_loader):
inputs, labels = inputs.to(device), labels.to(device)
optimizer.zero_grad()
outputs = vgg16(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
```
3.实现 two stream early fusion:
two stream early fusion 是通过将两个模态(如 RGB 图像和光流)的特征在早期融合起来来提高模型性能的技术。具体实现可以基于以下步骤:
- 首先,将两个模态的输入分别送入 VGG16 模型中,得到两个模态的特征;
- 接着,将两个模态的特征在第一个卷积块或第二个卷积块中进行融合,可以使用 torch.cat 函数将两个特征串联起来;
- 最后,将融合后的特征送入后续的网络层进行训练。
具体实现可以参考以下代码:
```python
class TwoStreamEarlyFusion(nn.Module):
def __init__(self, num_classes=10):
super(TwoStreamEarlyFusion, self).__init__()
self.rgb_vgg16 = models.vgg16(pretrained=True)
self.flow_vgg16 = models.vgg16(pretrained=True)
# 将两个模态的 VGG16 的第一层卷积融合起来
self.conv1 = nn.Conv2d(6, 64, kernel_size=3, stride=1, padding=1)
self.relu = nn.ReLU(inplace=True)
self.maxpool = nn.MaxPool2d(kernel_size=2, stride=2)
# 其他网络层
self.fc = nn.Linear(4096, num_classes)
def forward(self, rgb_inputs, flow_inputs):
rgb_features = self.rgb_vgg16.features(rgb_inputs)
flow_features = self.flow_vgg16.features(flow_inputs)
# 将两个特征串联起来
x = torch.cat((rgb_features, flow_features), dim=1)
# 在第一个卷积块中进行融合
x = self.conv1(x)
x = self.relu(x)
x = self.maxpool(x)
# 其他网络层
x = x.view(x.size(0), -1)
x = self.fc(x)
return x
```
希望以上内容对您有所帮助!
相关推荐
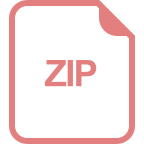
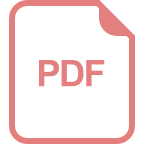
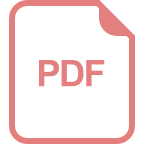
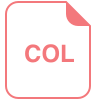













