wxpython的MessageDialog用法
时间: 2023-08-07 20:05:57 浏览: 121
wxpython的MessageDialog是一个弹出式对话框,用于在GUI应用程序中显示消息、警告、错误或询问用户输入等信息。以下是MessageDialog的用法:
1. 导入wxPython库:
```python
import wx
```
2. 创建MessageDialog:
```python
dlg = wx.MessageDialog(parent, message, caption, style)
```
参数说明:
- parent:父窗口,可以为None。
- message:对话框显示的消息。
- caption:对话框的标题。
- style:对话框的样式,可以为wx.OK|wx.CANCEL|wx.YES|wx.NO|wx.ICON_INFORMATION|wx.ICON_QUESTION|wx.ICON_WARNING|wx.ICON_ERROR等。
3. 显示MessageDialog:
```python
result = dlg.ShowModal()
```
4. 处理MessageDialog的返回值:
```python
if result == wx.ID_OK:
# 用户点击了OK按钮
elif result == wx.ID_CANCEL:
# 用户点击了Cancel按钮
elif result == wx.ID_YES:
# 用户点击了Yes按钮
elif result == wx.ID_NO:
# 用户点击了No按钮
```
完整示例代码如下:
```python
import wx
class MyFrame(wx.Frame):
def __init__(self, parent):
super().__init__(parent, title="MessageDialog Demo")
self.InitUI()
def InitUI(self):
panel = wx.Panel(self)
vbox = wx.BoxSizer(wx.VERTICAL)
hbox = wx.BoxSizer(wx.HORIZONTAL)
btn_info = wx.Button(panel, label="Info")
btn_warn = wx.Button(panel, label="Warning")
btn_error = wx.Button(panel, label="Error")
btn_question = wx.Button(panel, label="Question")
hbox.Add(btn_info, 1, wx.EXPAND|wx.ALL, 5)
hbox.Add(btn_warn, 1, wx.EXPAND|wx.ALL, 5)
hbox.Add(btn_error, 1, wx.EXPAND|wx.ALL, 5)
hbox.Add(btn_question, 1, wx.EXPAND|wx.ALL, 5)
vbox.Add(hbox, 1, wx.EXPAND|wx.ALL, 5)
panel.SetSizer(vbox)
btn_info.Bind(wx.EVT_BUTTON, self.OnInfo)
btn_warn.Bind(wx.EVT_BUTTON, self.OnWarn)
btn_error.Bind(wx.EVT_BUTTON, self.OnError)
btn_question.Bind(wx.EVT_BUTTON, self.OnQuestion)
self.Show()
def OnInfo(self, e):
dlg = wx.MessageDialog(self, "This is an information message.", "Information", wx.OK|wx.ICON_INFORMATION)
dlg.ShowModal()
dlg.Destroy()
def OnWarn(self, e):
dlg = wx.MessageDialog(self, "This is a warning message.", "Warning", wx.OK|wx.ICON_WARNING)
dlg.ShowModal()
dlg.Destroy()
def OnError(self, e):
dlg = wx.MessageDialog(self, "This is an error message.", "Error", wx.OK|wx.ICON_ERROR)
dlg.ShowModal()
dlg.Destroy()
def OnQuestion(self, e):
dlg = wx.MessageDialog(self, "Do you want to save your changes?", "Question", wx.YES_NO|wx.ICON_QUESTION)
result = dlg.ShowModal()
if result == wx.ID_YES:
print("User clicked Yes button")
elif result == wx.ID_NO:
print("User clicked No button")
dlg.Destroy()
if __name__ == '__main__':
app = wx.App()
frame = MyFrame(None)
app.MainLoop()
```
运行程序,点击不同的按钮,会弹出不同类型的MessageDialog。
阅读全文
相关推荐
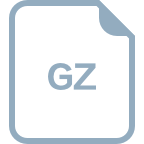
















