搭建visual studio 2010与openssl的环境
时间: 2023-10-05 16:05:17 浏览: 76
您可以按照以下步骤搭建Visual Studio 2010与OpenSSL的环境:
1. 首先,下载OpenSSL的源码,并进行解压缩。
2. 打开Visual Studio 2010,并新建一个项目。
3. 在项目属性中,选择“配置属性”->“C/C++”->“常规”,并将“附加包含目录”设置为OpenSSL的include目录路径。
4. 然后,选择“配置属性”->“链接器”->“常规”,并将“附加库目录”设置为OpenSSL的lib目录路径。
5. 接下来,在“配置属性”->“链接器”->“输入”中,将“附加依赖项”设置为所需的OpenSSL库文件名。
6. 最后,保存并生成项目,以确保环境搭建完成。
注意:以上步骤仅供参考,您需要根据自己的实际情况进行调整。同时,请确保遵守OpenSSL的使用协议及版权声明。
相关问题
(一)使用C语言编写DES算法。 (二)搭建visual studio 2010与openssl的环境。 (三)用openssl密码库实现对称加密解密算法(DES、AES),并分析算法源码。
(一)使用C语言编写DES算法。
DES算法是一种对称加密算法,它的加密和解密使用相同的密钥。下面是一个使用C语言编写DES算法的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "des.h"
#define KEY "12345678" // 密钥
#define BLOCK_SIZE 8 // 块大小为8字节
int main(int argc, char **argv) {
char *plaintext = "hello world"; // 明文
char ciphertext[BLOCK_SIZE]; // 密文
char decryptedtext[BLOCK_SIZE]; // 解密后的明文
// 初始化密钥
des_key_schedule key;
des_set_key((des_cblock *)KEY, key);
// 加密
des_ecb_encrypt((des_cblock *)plaintext, (des_cblock *)ciphertext, key, DES_ENCRYPT);
// 输出密文
printf("ciphertext: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%02x", (unsigned char)ciphertext[i]);
}
printf("\n");
// 解密
des_ecb_encrypt((des_cblock *)ciphertext, (des_cblock *)decryptedtext, key, DES_DECRYPT);
// 输出解密后的明文
printf("decryptedtext: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%c", decryptedtext[i]);
}
printf("\n");
return 0;
}
```
(二)搭建visual studio 2010与openssl的环境。
要在Visual Studio 2010中使用OpenSSL,需要先安装OpenSSL并设置好环境变量。以下是在Windows上安装OpenSSL并设置环境变量的步骤:
1. 下载OpenSSL二进制文件:https://slproweb.com/products/Win32OpenSSL.html
2. 安装OpenSSL,选择默认安装路径。
3. 添加OpenSSL的bin目录到系统环境变量中,例如:C:\OpenSSL-Win32\bin。
4. 在Visual Studio 2010中打开项目,右键单击项目,选择“属性”。
5. 在“VC++目录”中的“包含目录”中添加OpenSSL的include目录,例如:C:\OpenSSL-Win32\include。
6. 在“VC++目录”中的“库目录”中添加OpenSSL的lib目录,例如:C:\OpenSSL-Win32\lib。
7. 在“链接器”中的“输入”中添加OpenSSL的库文件,例如:libeay32.lib。
(三)用openssl密码库实现对称加密解密算法(DES、AES),并分析算法源码。
OpenSSL密码库提供了DES和AES算法的加密解密函数。以下是使用OpenSSL密码库实现DES和AES算法的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/des.h>
#include <openssl/aes.h>
#define KEY "12345678" // 密钥
#define BLOCK_SIZE 8 // 块大小为8字节
int main(int argc, char **argv) {
char *plaintext = "hello world"; // 明文
char ciphertext[BLOCK_SIZE]; // 密文
char decryptedtext[BLOCK_SIZE]; // 解密后的明文
// 初始化密钥
DES_key_schedule des_key;
DES_set_key((DES_cblock *)KEY, &des_key);
// 加密
DES_ecb_encrypt((DES_cblock *)plaintext, (DES_cblock *)ciphertext, &des_key, DES_ENCRYPT);
// 输出密文
printf("ciphertext: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%02x", (unsigned char)ciphertext[i]);
}
printf("\n");
// 解密
DES_ecb_encrypt((DES_cblock *)ciphertext, (DES_cblock *)decryptedtext, &des_key, DES_DECRYPT);
// 输出解密后的明文
printf("decryptedtext: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%c", decryptedtext[i]);
}
printf("\n");
// 初始化密钥
AES_KEY aes_key;
AES_set_encrypt_key((unsigned char *)KEY, 128, &aes_key);
// 加密
AES_encrypt((unsigned char *)plaintext, (unsigned char *)ciphertext, &aes_key);
// 输出密文
printf("ciphertext: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%02x", (unsigned char)ciphertext[i]);
}
printf("\n");
// 解密
AES_set_decrypt_key((unsigned char *)KEY, 128, &aes_key);
AES_decrypt((unsigned char *)ciphertext, (unsigned char *)decryptedtext, &aes_key);
// 输出解密后的明文
printf("decryptedtext: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%c", decryptedtext[i]);
}
printf("\n");
return 0;
}
```
在以上代码中,使用了DES_set_key、DES_ecb_encrypt、AES_set_encrypt_key、AES_encrypt、AES_set_decrypt_key和AES_decrypt等函数实现了DES和AES算法的加密解密。这些函数的源码可以在OpenSSL的源代码中找到。
visual studio怎么用openssl
要在Visual Studio中使用OpenSSL,您需要遵循以下步骤:
1. 下载和安装OpenSSL库
从官方网站下载和安装OpenSSL库,确保选择与您的Visual Studio版本和系统架构(32位或64位)兼容的版本。
2. 将OpenSSL包含目录添加到项目中
在Visual Studio中打开您的项目,右键单击项目名称并选择“属性”选项。在属性窗口中,选择“VC++目录”选项卡,然后将OpenSSL库的包含目录添加到“包含目录”中。
3. 将OpenSSL库目录添加到项目中
在属性窗口中,选择“VC++目录”选项卡,然后将OpenSSL库的库目录添加到“库目录”中。
4. 链接OpenSSL库
在属性窗口中,选择“链接器”选项卡,然后选择“输入”选项卡。在“附加依赖项”中添加OpenSSL库文件的名称,例如“libeay32.lib”和“ssleay32.lib”。
现在,您可以在您的Visual Studio项目中使用OpenSSL库了。
相关推荐
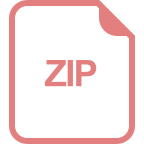
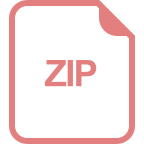
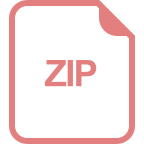












