unity中父物体水平转动朝向目标点,子物体竖直转动朝向目标点,依次循环形成S轨迹的代码
时间: 2023-09-09 19:09:51 浏览: 44
可以使用以下代码实现:
```
using UnityEngine;
public class SPathMovement : MonoBehaviour
{
public Transform[] waypoints; // 存储所有路径点的数组
public float moveSpeed = 5f; // 移动速度
public float rotationSpeed = 5f; // 转向速度
private int currentWaypointIndex = 0; // 当前路径点索引
private Transform currentWaypoint; // 当前路径点
void Start()
{
currentWaypoint = waypoints[currentWaypointIndex]; // 初始化当前路径点
}
void Update()
{
// 计算朝向当前路径点的旋转角度
Vector3 direction = currentWaypoint.position - transform.position;
Quaternion rotation = Quaternion.LookRotation(direction);
// 让父物体水平转向当前路径点
transform.rotation = Quaternion.Slerp(transform.rotation, rotation, rotationSpeed * Time.deltaTime);
// 让子物体竖直转向当前路径点
Vector3 localDirection = transform.InverseTransformDirection(direction);
float angle = Mathf.Atan2(localDirection.y, localDirection.x) * Mathf.Rad2Deg;
transform.GetChild(0).rotation = Quaternion.Euler(new Vector3(0f, 0f, angle - 90f));
// 移动到当前路径点
transform.position = Vector3.MoveTowards(transform.position, currentWaypoint.position, moveSpeed * Time.deltaTime);
// 到达当前路径点后切换到下一个路径点
if (Vector3.Distance(transform.position, currentWaypoint.position) < 0.1f)
{
currentWaypointIndex++;
if (currentWaypointIndex >= waypoints.Length)
{
currentWaypointIndex = 0;
}
currentWaypoint = waypoints[currentWaypointIndex];
}
}
}
```
在这个脚本中,我们通过存储所有路径点的数组来实现S轨迹移动。在每帧更新中,我们计算出朝向当前路径点的旋转角度,并让父物体水平转向当前路径点,子物体竖直转向当前路径点。然后,我们使用`MoveTowards`方法来移动到当前路径点。如果到达当前路径点,我们就切换到下一个路径点。最后,我们使用`Slerp`方法来平滑转向目标方向,并使用`Euler`方法将角度转换为欧拉角。
要使用这个脚本,可以将其添加到带有父物体和子物体的游戏对象上,并将路径点拖到`waypoints`数组中。然后,调整移动速度和转向速度以获得所需的效果。
相关推荐
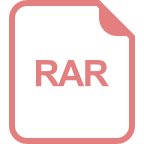
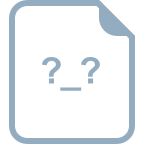














