python实现万年历
时间: 2023-06-29 11:15:23 浏览: 596
以下是 Python 实现万年历的代码:
```python
import calendar
# 输入年份和月份
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
# 获取该月份的日历字符串
cal = calendar.month(year, month)
# 输出日历
print("以下是%d年%d月的日历:" % (year, month))
print(cal)
```
输入年份和月份后,程序会调用 Python 内置的 `calendar.month()` 函数获取该月份的日历字符串,然后输出日历。这个程序可以输出任意年份和月份的日历,可以实现万年历的功能。
相关问题
Python实现万年历
以下是Python实现万年历的代码:
```python
# 定义函数,判断某一年是否为闰年
def is_leap(year):
if year % 4 == 0 and year % 100 != 0 or year % 400 == 0:
return True
else:
return False
# 定义函数,计算某一年的总天数
def total_days(year):
total = 0
for y in range(1900, year):
if is_leap(y):
total += 366
else:
total += 365
return total
# 定义函数,计算某一月的天数
def month_days(year, month):
if month in [1, 3, 5, 7, 8, 10, 12]:
return 31
elif month == 2:
if is_leap(year):
return 29
else:
return 28
else:
return 30
# 按照格式输出日历
def print_calendar(year, month):
# 定义变量,记录当前日期
day = 1
# 计算当前月份的第一天是星期几
first_day = (total_days(year) + sum([month_days(year, m) for m in range(1, month)])) % 7
# 输出月份和星期几的表头
print("{0}年{1}月".format(year, month))
print("日 一 二 三 四 五 六")
# 输出日历
for i in range(6):
for j in range(7):
if i == 0 and j < first_day or day > month_days(year, month):
print(" ", end="")
else:
print("{0:2d}".format(day), end="")
day += 1
if j < 6:
print(" ", end="")
print()
# 测试代码
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
print_calendar(year, month)
```
在运行代码时,需要输入指定的年份和月份,程序会输出该月份的日历。例如:
```
请输入年份:2021
请输入月份:10
2021年10月
日 一 二 三 四 五 六
1 2
3 4 5 6 7 8 9
10 11 12 13 14 15 16
17 18 19 20 21 22 23
24 25 26 27 28 29 30
31
```
这就是Python实现万年历的代码。
python实现万年历包含农历
好的,我可以帮您实现一个Python万年历,包含农历。您需要先安装一个Python库 called lunarcalendar。您可以使用 pip 命令来安装它:
```python
pip install lunarcalendar
```
然后,您可以使用以下代码来实现一个简单的万年历(包含公历和农历):
```python
import calendar
from lunarcalendar import Converter, Solar, Lunar
def get_calendar(year, month):
"""
获取指定月份的公历和农历日历
"""
# 获取公历的月历
cal = calendar.monthcalendar(year, month)
# 获取农历的月历
lunar_cal = []
for week in cal:
lunar_week = []
for day in week:
if day == 0:
lunar_week.append((0, ''))
continue
# 将公历日期转换为农历日期
solar_date = Solar(year, month, day)
lunar_date = Converter.solar2lunar(solar_date)
# 获取农历日期的中文表示
lunar_day = Lunar(lunar_date.year, lunar_date.month, lunar_date.day)
lunar_day_str = lunar_day.day_full
lunar_week.append((day, lunar_day_str))
lunar_cal.append(lunar_week)
return cal, lunar_cal
# 测试代码
year = 2022
month = 12
cal, lunar_cal = get_calendar(year, month)
print('{}年{}月'.format(year, month))
print('公历:')
print(calendar.month_name[month])
print(calendar.weekheader(2))
for week in cal:
print(week)
print('农历:')
for week in lunar_cal:
for day in week:
print('{:<2} '.format(day[1]), end='')
print()
```
这个程序将打印出给定年份和月份的公历和农历日历。您可以根据需要进行修改。
阅读全文
相关推荐
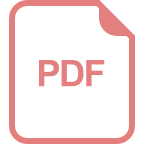











