大型稀疏矩阵的对称下三角非零元素的一维存储及共轭梯度法求解C#代码
时间: 2024-05-06 11:14:54 浏览: 89
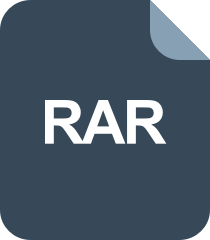
稀疏矩阵存储和转置及乘法 c++源代码 原创

以下是C#代码示例:
```
using System;
public class SparseMatrix
{
private int size;
private int[] rowPtr;
private int[] colInd;
private double[] values;
public SparseMatrix(int size, int nnz)
{
this.size = size;
rowPtr = new int[size + 1];
colInd = new int[nnz];
values = new double[nnz];
}
public void Set(int row, int col, double value)
{
int index = rowPtr[row];
while (index < rowPtr[row + 1] && colInd[index] < col)
{
index++;
}
if (index < rowPtr[row + 1] && colInd[index] == col)
{
values[index] = value;
}
else
{
for (int i = size; i > row; i--)
{
rowPtr[i] = rowPtr[i - 1];
}
rowPtr[row + 1]++;
for (int i = rowPtr[row + 1]; i <= size; i++)
{
rowPtr[i]++;
}
colInd[index] = col;
values[index] = value;
}
}
public double Get(int row, int col)
{
int index = rowPtr[row];
while (index < rowPtr[row + 1] && colInd[index] < col)
{
index++;
}
if (index < rowPtr[row + 1] && colInd[index] == col)
{
return values[index];
}
else
{
return 0.0;
}
}
public void ConjugateGradient(double[] b, double[] x, double tolerance, int maxIterations)
{
double[] r = new double[size];
double[] p = new double[size];
double[] Ap = new double[size];
for (int i = 0; i < size; i++)
{
x[i] = 0.0;
r[i] = b[i];
p[i] = r[i];
}
double rsold = DotProduct(r, r);
for (int i = 0; i < maxIterations; i++)
{
Multiply(p, Ap);
double alpha = rsold / DotProduct(p, Ap);
for (int j = 0; j < size; j++)
{
x[j] += alpha * p[j];
r[j] -= alpha * Ap[j];
}
double rsnew = DotProduct(r, r);
if (Math.Sqrt(rsnew) < tolerance)
{
break;
}
for (int j = 0; j < size; j++)
{
p[j] = r[j] + (rsnew / rsold) * p[j];
}
rsold = rsnew;
}
}
private double DotProduct(double[] a, double[] b)
{
double result = 0.0;
for (int i = 0; i < size; i++)
{
result += a[i] * b[i];
}
return result;
}
private void Multiply(double[] x, double[] result)
{
for (int i = 0; i < size; i++)
{
result[i] = 0.0;
for (int j = rowPtr[i]; j < rowPtr[i + 1]; j++)
{
result[i] += values[j] * x[colInd[j]];
}
}
}
}
```
其中,SparseMatrix类表示一个稀疏矩阵,包含以下方法:
- 构造函数SparseMatrix(int size, int nnz):创建一个大小为size、非零元素数量为nnz的稀疏矩阵对象。
- 方法Set(int row, int col, double value):设置第row行第col列的元素值为value。如果该元素已存在,则覆盖原有值;否则,将该元素插入到正确位置。
- 方法Get(int row, int col):获取第row行第col列的元素值。
- 方法ConjugateGradient(double[] b, double[] x, double tolerance, int maxIterations):使用共轭梯度法求解线性方程组Ax=b,其中A为该稀疏矩阵,b为右侧向量,x为解向量,tolerance为精度要求,maxIterations为最大迭代次数。
另外,还定义了私有方法DotProduct(double[] a, double[] b)和Multiply(double[] x, double[] result),分别用于计算向量内积和矩阵向量积。
阅读全文
相关推荐
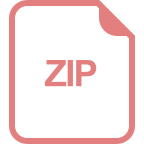
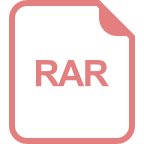
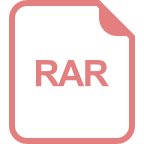
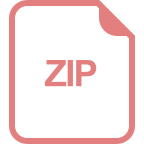
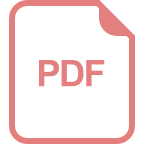
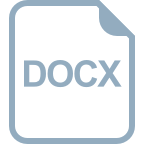
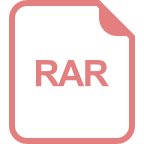
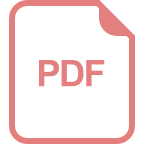
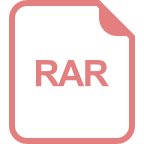
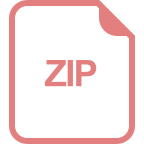
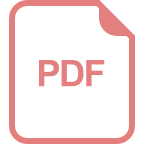
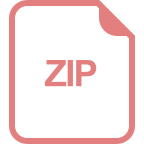
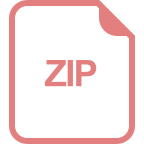
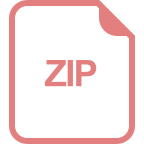