用JAVA写个快速排序
时间: 2023-09-27 11:05:24 浏览: 82
### 回答1:
我不是很清楚,但是我可以提供你一些关于用Java写快速排序的参考资料: 1. https://www.baeldung.com/java-quicksort
2. https://www.geeksforgeeks.org/quick-sort/
3. https://www.tutorialspoint.com/data_structures_algorithms/quick_sort_algorithm.htm
### 回答2:
快速排序是一种常用的排序算法,采用分治策略,通过递归地将数组划分为较小和较大的两个子数组,然后分别对两个子数组进行排序,最终将整个数组有序。
下面是用JAVA编写的快速排序算法代码:
```java
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
swap(arr, i, j);
}
}
swap(arr, i + 1, high);
return i + 1;
}
public static void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
public static void main(String[] args) {
int[] arr = {10, 7, 8, 9, 1, 5};
int n = arr.length;
quickSort(arr, 0, n - 1);
System.out.println("排序后的数组:");
for (int i = 0; i < n; i++) {
System.out.print(arr[i] + " ");
}
}
}
```
以上代码实现了快速排序算法。在`quickSort`方法中,首先选择数组中的一个元素作为基准值`pivot`,然后通过`partition`方法将数组划分为两部分,并返回基准值的索引。接着,递归地对基准值左边和右边的子数组再次进行划分和排序,直到完成整个数组的排序。在`partition`方法中,通过双指针遍历数组,将小于基准值的元素放到左边,大于基准值的元素放到右边,最后将基准值放到正确的位置上。
在`main`方法中,初始化一个整型数组,并调用`quickSort`方法对数组进行排序,最后输出排序后的数组。
快速排序算法的时间复杂度为O(nlogn),其中n为数组的长度。
### 回答3:
快速排序是一种高效的排序算法,用于对数组进行排序。下面是使用Java编写的快速排序算法:
```java
public class QuickSort {
public void quickSort(int[] array, int low, int high) {
if (low < high) {
// 将数组分区,并获取分区点的索引
int pivotIndex = partition(array, low, high);
// 对分区点的左边部分进行快速排序
quickSort(array, low, pivotIndex - 1);
// 对分区点的右边部分进行快速排序
quickSort(array, pivotIndex + 1, high);
}
}
private int partition(int[] array, int low, int high) {
// 以数组最后一个元素作为分区点
int pivot = array[high];
// 定义左指针和右指针
int i = low - 1;
for (int j = low; j < high; j++) {
// 如果当前元素小于等于分区点,则将其移动到左侧
if (array[j] <= pivot) {
i++;
swap(array, i, j);
}
}
// 将分区点移动到正确的位置
swap(array, i + 1, high);
// 返回分区点的索引
return i + 1;
}
private void swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
public static void main(String[] args) {
int[] array = {5, 3, 8, 2, 1, 6, 4};
QuickSort quickSort = new QuickSort();
quickSort.quickSort(array, 0, array.length - 1);
// 输出排序后的结果
for (int num : array) {
System.out.print(num + " ");
}
}
}
```
以上是用Java编写的快速排序算法,它通过递归地对数组进行分区,并通过分区点将数组划分为左右两个部分。再对左侧和右侧的部分分别进行快速排序,直到数组完全有序。快速排序的时间复杂度为O(nlogn),是一种非常高效的排序算法。
相关推荐






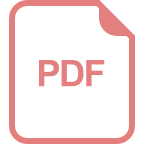