java分块查找代码
时间: 2023-09-21 11:06:23 浏览: 169
### 回答1:
Java分块查找是一种查找算法,用于在数组或其他有序的数据结构中查找特定的元素。下面是一个示例代码:
```
public class BlockSearch {
public static void main(String[] args) {
int[] arr = {2, 4, 6, 8, 10, 12, 14, 16, 18, 20};
int blockSize = 3;
int searchElement = 14;
int blockIndex = searchElement / blockSize;
int start = blockIndex * blockSize;
int end = start + blockSize - 1;
while (start <= end && arr[start] <= searchElement && arr[end] >= searchElement) {
if (arr[start] == searchElement) {
System.out.println("Element found at index " + start);
return;
}
if (arr[end] == searchElement) {
System.out.println("Element found at index " + end);
return;
}
start++;
end--;
}
System.out.println("Element not found in the array");
}
}
```
在上面的代码中,我们首先将数组分成若干个块,每个块的大小由`blockSize`变量指定。然后我们计算出要搜索的元素所在的块的索引,并将搜索范围限制在该块内。最后,我们使用双指针查找法在该块内查找元素。如果找到了要查找的元素,就输出它的索引,否则输出“元素不存在”的消息。
### 回答2:
对于Java分块查找代码,可以采用以下方式实现:
首先,需要准备一个有序的数组,以便于进行分块查找。假设数组名为`arr[]`,其中包含了一定量的元素。
接下来,需要定义一个分块查找的方法,以便于在数组中查找指定的元素。该方法的输入参数包括数组`arr[]`,要查找的值`value`,以及每个块的大小`blockSize`。
具体代码实现如下:
```java
public class BlockSearch {
public static int blockSearch(int arr[], int value, int blockSize) {
int n = arr.length; // 数组的长度
int numOfBlocks = (int) Math.ceil((double) n / blockSize); // 计算块的数量
// 遍历所有的块
for (int i = 0; i < numOfBlocks; i++) {
int start = i * blockSize; // 当前块的起始位置
int end = Math.min(start + blockSize - 1, n - 1); // 当前块的结束位置
// 在当前块中进行线性查找,查找值为value
for (int j = start; j <= end; j++) {
if (arr[j] == value) {
return j; // 返回找到的元素在数组中的位置
}
}
}
return -1; // 没有找到元素
}
public static void main(String[] args) {
int arr[] = {1, 3, 5, 7, 9, 2, 4, 6, 8, 10};
int value = 5;
int blockSize = 3;
int index = blockSearch(arr, value, blockSize);
if (index != -1) {
System.out.println("元素 " + value + " 的位置为 " + index);
} else {
System.out.println("没有找到元素 " + value);
}
}
}
```
以上代码实现了一个简单的分块查找方法。它首先将数组分成块,然后再每个块中进行线性查找,以找到指定的元素。最后通过返回值来表示查找结果,-1表示没有找到元素,其他值则表示元素在数组中的位置。
### 回答3:
分块查找也叫索引查找,是一种多级索引的查找方法。它将数据分为若干块,每一块内部有序,块间有序。通过在每一块的末尾建立一个索引表,记录每一块的起始位置和关键字,从而实现在较少的数据量上进行查找。
以下是一个使用Java实现分块查找的代码:
```java
import java.util.ArrayList;
import java.util.List;
public class BlockSearch {
public static int search(int[] data, int value, int blockSize) {
int blockIndex = -1;
// 建立索引表
List<Integer> index = new ArrayList<>();
for (int i = 0; i < data.length; i += blockSize) {
index.add(data[i]);
}
// 在索引表中查找所在的块
for (int i = 0; i < index.size(); i++) {
if (value <= index.get(i)) {
blockIndex = i;
break;
}
}
// 在所在的块中进行顺序查找
int start = blockIndex * blockSize;
int end = Math.min(start + blockSize, data.length);
for (int i = start; i < end; i++) {
if (data[i] == value) {
return i;
}
}
return -1; // 如果没找到,返回-1
}
public static void main(String[] args) {
int[] data = {5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65};
int blockSize = 4;
int value = 40;
int result = search(data, value, blockSize);
if (result != -1) {
System.out.println("找到了,索引位置是:" + result);
} else {
System.out.println("没找到");
}
}
}
```
上述代码中,`search`方法接受数据数组、待查找值和块大小作为参数,返回找到的索引位置。首先建立索引表,然后在索引表中查找所在的块。最后,在所在的块中进行顺序查找,找到则返回索引位置,否则返回-1。
阅读全文
相关推荐

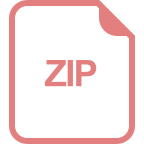
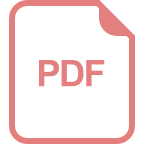
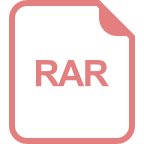
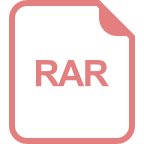
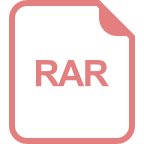
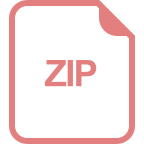
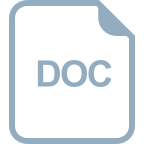
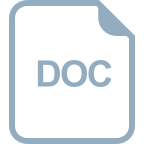
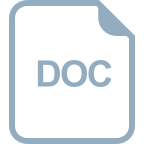
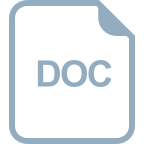
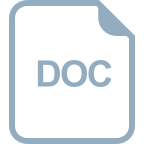
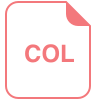
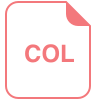
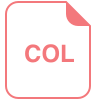
