python numpy查找元素位置
时间: 2023-04-27 13:03:42 浏览: 1314
可以使用numpy库中的where函数来查找元素位置。
例如,假设有一个numpy数组a:
```
import numpy as np
a = np.array([1, 2, 3, 4, 5])
```
要查找元素3的位置,可以使用where函数:
```
index = np.where(a == 3)
print(index)
```
输出结果为:
```
(array([2]),)
```
其中,array([2])表示元素3在数组a中的位置为第3个元素(数组下标从0开始计数)。
如果要查找多个元素的位置,可以将多个元素放在一个列表中,然后使用numpy的in1d函数来判断元素是否在数组中,再使用where函数来查找位置。
例如,假设要查找元素2和4的位置:
```
b = np.array([2, 4])
index = np.where(np.in1d(a, b))
print(index)
```
输出结果为:
```
(array([1, 3]),)
```
其中,array([1, 3])表示元素2和4在数组a中的位置分别为第2个和第4个元素。
相关问题
numpy查找元素索引
Sure, I can help you with that! To find the index of an element in a numpy array, you can use the `numpy.where` function. Here's an example:
```python
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
idx = np.where(arr == 3)
print(idx)
```
This will output `(array([2]),)`, which means that the index of the element `3` in the array is `2`.
python中numpy查找最近值,并输出最近值以及最近值得index
您可以使用numpy中的argmin函数来查找最近值的索引,然后使用该索引获取最近值。下面是一个示例代码:
```python
import numpy as np
# 创建一个示例数组
arr = np.array([1, 2, 3, 4, 5, 6])
# 查找最近值的索引
value = 3.8
index = np.abs(arr - value).argmin()
# 获取最近值
nearest_value = arr[index]
# 输出结果
print("最近值为:", nearest_value)
print("最近值的索引为:", index)
```
在这个示例中,我们创建了一个数组`arr`,然后通过`np.abs(arr - value).argmin()`查找最近值的索引。这里,`np.abs(arr - value)`计算了数组`arr`中每个元素与目标值`value`的差的绝对值,然后`argmin()`函数返回最小值的索引。最后,我们使用`arr[index]`获取最近值,并输出结果。
阅读全文
相关推荐
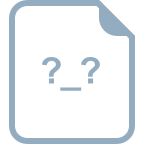
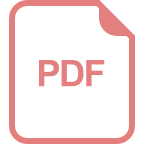
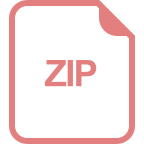
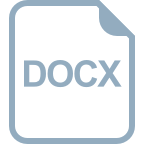
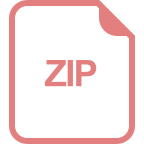
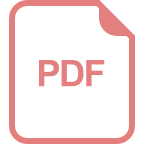
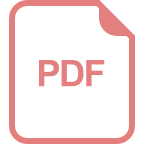
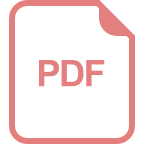
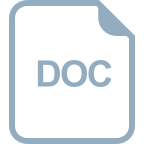
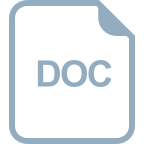
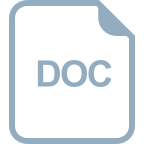
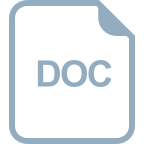
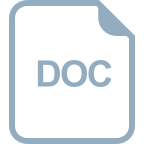
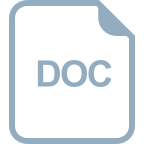
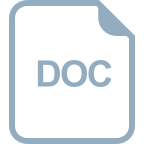

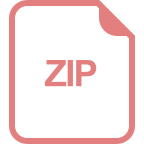