springboot如何获取spring容器的Bean
时间: 2024-03-08 07:48:13 浏览: 123
SpringBoot可以通过使用@Autowired注解或者@Resource注解来获取Spring容器中的Bean。这些注解可以用在类的属性、构造函数或者方法上。
示例代码:
```java
@RestController
public class MyController {
@Autowired
private MyService myService;
@GetMapping("/hello")
public String hello() {
return myService.sayHello();
}
}
```
在上面的示例中,@Autowired注解被用来注入MyService这个Bean。然后在hello()方法中使用这个Bean的方法。
注:需要在启动类上加上@EnableAutoConfiguration注解,SpringBoot才能自动扫描并初始化Bean。
相关问题
springboot 获取容器中的bean
在使用SpringBoot的过程中,获取容器中的bean可以使用@Autowired注解或者通过ApplicationContext获取。其中,@Autowired注解可以自动注入容器中的bean实例,而ApplicationContext则提供了多种方式来获取bean。
使用@Autowired注解获取bean,只需要在需要使用bean的属性或者构造函数上标注该注解,并且确保该bean在程序启动时已经注入到容器中。例如:
```java
@Service
public class MyService {
//...
}
@RestController
public class MyController {
@Autowired
private MyService myService;
//...
}
```
在上面的例子中,MyController类中的myService属性使用@Autowired注解进行了注入,SpringBoot会自动从容器中查找类型为MyService的bean,并将其注入到属性中。
除了@Autowired注解,还可以使用ApplicationContext来获取bean实例。具体来说,可以通过@ComponentScan注解将指定包下的类扫描并注册到Spring容器中,然后使用ApplicationContext来获取这些bean。例如:
```java
@SpringBootApplication
@ComponentScan(basePackages = "com.example")
public class MyApplication {
//...
}
@Service
public class MyService {
//...
}
public class MyUtils {
public static MyService getService() {
ApplicationContext context = new AnnotationConfigApplicationContext(MyApplication.class);
return context.getBean(MyService.class);
}
}
```
在上面的例子中,MyApplication使用@ComponentScan注解来指定需要注册到容器中的类所在的包,然后在MyUtils中使用ApplicationContext来获取容器中的MyService实例。需要注意的是,在每次获取bean实例时都会创建一个新的ApplicationContext,因此可能会有性能上的影响。
SpringBoot获取Spring上下文
在Spring Boot中,可以使用ApplicationContext来获取Spring上下文。下面是一个示例代码[^1]:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ApplicationContext;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
ApplicationContext context = SpringApplication.run(DemoApplication.class, args);
// 获取Spring上下文中的bean
MyBean myBean = context.getBean(MyBean.class);
myBean.doSomething();
}
}
class MyBean {
public void doSomething() {
System.out.println("Doing something...");
}
}
```
在上面的示例中,我们通过调用`SpringApplication.run()`方法来启动Spring Boot应用,并获取ApplicationContext对象。然后,我们可以使用`getBean()`方法从上下文中获取我们需要的bean,这里是一个名为MyBean的bean。最后,我们调用MyBean的方法来执行相应的操作。
需要注意的是,上述示例中的MyBean类需要被Spring容器管理,可以通过在类上添加@Component注解或者其他相关注解来实现。
阅读全文
相关推荐
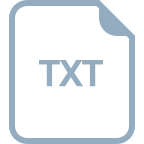
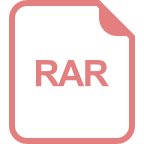
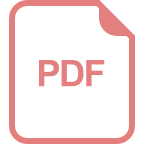
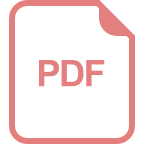
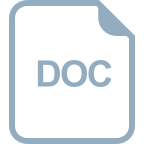
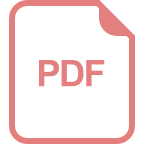
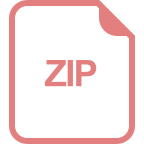
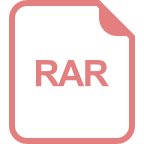






