antd pro预览打印pdf文件
时间: 2023-08-16 14:09:44 浏览: 285
Ant Design Pro 中可以使用 `react-pdf` 这个第三方库来实现 PDF 文件的预览和打印。具体实现步骤如下:
1. 安装 `react-pdf` 库
```
npm install react-pdf
```
2. 在组件中引入 `Document`、`Page` 和 `pdfjs` 组件
```javascript
import { Document, Page, pdfjs } from 'react-pdf';
pdfjs.GlobalWorkerOptions.workerSrc = `//cdnjs.cloudflare.com/ajax/libs/pdf.js/${pdfjs.version}/pdf.worker.js`;
```
3. 在页面中使用 `Document` 和 `Page` 组件来渲染 PDF 文件
```javascript
<Document file="/example.pdf">
<Page pageNumber={1} />
</Document>
```
其中 `file` 属性指定要渲染的 PDF 文件路径,`Page` 组件的 `pageNumber` 属性指定要渲染的页码。
4. 添加打印功能
```javascript
import { pdfjs, Document, Page, pdf } from 'react-pdf';
import { Button } from 'antd';
pdfjs.GlobalWorkerOptions.workerSrc = `//cdnjs.cloudflare.com/ajax/libs/pdf.js/${pdfjs.version}/pdf.worker.js`;
class PDFViewer extends React.Component {
state = {
numPages: null,
pageNumber: 1,
};
onDocumentLoadSuccess = ({ numPages }) => {
this.setState({ numPages });
};
goToPrevPage = () => {
this.setState(prevState => ({ pageNumber: prevState.pageNumber - 1 }));
};
goToNextPage = () => {
this.setState(prevState => ({ pageNumber: prevState.pageNumber + 1 }));
};
printDocument = () => {
pdfjs.GlobalWorkerOptions.workerSrc = `//cdnjs.cloudflare.com/ajax/libs/pdf.js/${pdfjs.version}/pdf.worker.js`;
const loadingTask = pdfjs.getDocument(this.props.file);
loadingTask.promise.then(pdf => {
const printOptions = {
...pdfjs.DefaultPrintParams,
printResolution: 150,
embedJavascript: true,
};
const pdfWindow = window.open('', '_blank', 'width=800,height=600,scrollbars=no');
pdfWindow.document.write('<html><head><title>Print</title></head><body>');
pdfWindow.document.write('<embed type="application/pdf" ');
pdfWindow.document.write(`src="${this.props.file}" `);
pdfWindow.document.write(`id="pdfDocument" `);
pdfWindow.document.write(`print="print" `);
pdfWindow.document.write(`options='${JSON.stringify(printOptions)}' `);
pdfWindow.document.write('></embed>');
pdfWindow.document.write('</body></html>');
pdfWindow.document.close();
setTimeout(() => {
pdfWindow.print();
}, 500);
});
};
render() {
const { pageNumber, numPages } = this.state;
return (
<div>
<p>
Page {pageNumber} of {numPages}
</p>
<Document file={this.props.file} onLoadSuccess={this.onDocumentLoadSuccess}>
<Page pageNumber={pageNumber} />
</Document>
<Button disabled={pageNumber <= 1} onClick={this.goToPrevPage}>
Previous
</Button>
<Button disabled={pageNumber >= numPages} onClick={this.goToNextPage}>
Next
</Button>
<Button onClick={this.printDocument}>Print</Button>
</div>
);
}
}
export default PDFViewer;
```
在上面的代码中,我们添加了一个 `printDocument` 方法,该方法会打开一个新的窗口,并在窗口中显示 PDF 文件,然后调用 `window.print()` 方法将 PDF 文件打印出来。注意,该方法中使用了 PDF.js 库的 `getDocument` 方法来获取 PDF 文件的信息。
这样就可以在 Ant Design Pro 中预览和打印 PDF 文件了。
阅读全文
相关推荐
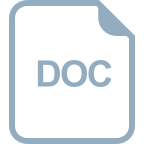
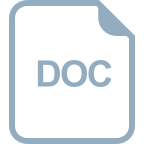
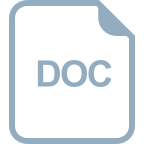
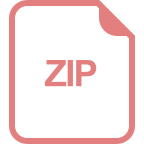
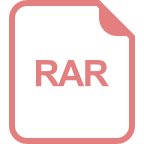
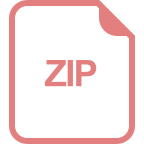
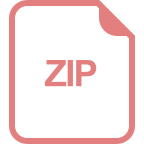
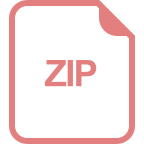
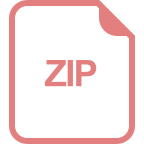
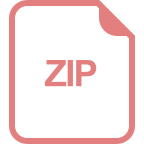
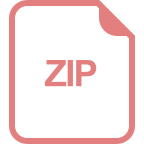







