用springboot,vue,mybatis和elementUI实现上传PDF文件到数据库,需要上传到数据库的内容有文件名,文件上传时间,文件路径,上传者的编号。请给我domain代码,controller代码,业务逻辑组件类代码,前端vue和element代码,mybatis代码,springboot配置文件和数据库建表语句,并用注释讲解
时间: 2023-05-21 21:02:44 浏览: 201
print() 是一个在编程中常用的函数,它可以将指定的文本或变量输出到控制台或终端窗口,以便于程序员进行调试和查看程序运行时的状态。在 Lua 编程语言中,print() 函数可以用来输出字符串、数字、布尔值等数据类型,也可以输出表格、函数等复杂的数据结构。
相关问题
用springboot,vue,mybatis和elementUl实现上传PDF文件到数据库,需要上传到数据库的内容有文件名,文件上传时间,文件路径,上传者编号。请告诉我springboot配置文件,domain代码,controller代码,service代码,前端vue和element代码,mybatis代码和数据库建表语句,并用注释讲解
由于涉及到多个文件和代码,建议您在学习本文时,将代码和文件分别保存在不同的位置,以便更好地跟随本文进行操作。
1. 配置文件
在Spring Boot中,我们可以通过application.properties或application.yml配置文件来配置我们的应用程序。以下是application.properties文件中需要添加的内容:
```
# 数据库连接信息
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8&useSSL=false
spring.datasource.username=root
spring.datasource.password=root
# MyBatis配置信息
mybatis.type-aliases-package=com.example.demo.entity
mybatis.mapper-locations=classpath:mapper/*.xml
# 文件上传配置信息
spring.servlet.multipart.max-file-size=50MB
spring.servlet.multipart.max-request-size=50MB
```
上述配置文件中,我们首先配置了数据库连接信息,包括数据库连接驱动、数据库url、用户名和密码。接着,我们配置了MyBatis相关的信息,包括实体类所在的包和Mapper文件所在的位置。最后,我们配置了文件上传的最大文件大小和最大请求大小。
2. Domain代码
在我们的项目中,我们需要定义一个实体类来对应数据库中的表。以下是我们的实体类代码:
```
public class PdfFile {
private Long id; // 主键
private String fileName; // 文件名
private Date uploadTime; // 上传时间
private String filePath; // 文件路径
private Long uploaderId; // 上传者编号
// getter and setter
}
```
上述代码中,我们定义了一个PdfFile类,该类有五个属性,分别对应数据库中的表字段。其中,id为主键,fileName为文件名,uploadTime为上传时间,filePath为文件路径,uploaderId为上传者编号。
3. Controller代码
在我们的项目中,我们需要定义一个Controller来接收前端请求,并调用Service层的方法来处理请求。以下是我们的Controller代码:
```
@RestController
@RequestMapping("/pdf")
public class PdfFileController {
@Autowired
private PdfFileService pdfFileService;
/**
* 上传PDF文件
*/
@PostMapping("/upload")
public Result upload(@RequestParam("file") MultipartFile file, Long uploaderId) {
try {
String fileName = file.getOriginalFilename(); // 获取文件名
String filePath = "D:/upload/"; // 上传文件保存的路径
File dest = new File(filePath + fileName);
file.transferTo(dest); // 将文件保存到本地
PdfFile pdfFile = new PdfFile();
pdfFile.setFileName(fileName);
pdfFile.setFilePath(filePath + fileName);
pdfFile.setUploaderId(uploaderId);
pdfFileService.insert(pdfFile); // 将文件信息保存到数据库中
return Result.success("上传成功!");
} catch (IOException e) {
e.printStackTrace();
return Result.fail("上传失败!");
}
}
}
```
上述代码中,我们首先使用@RestController和@RequestMapping注解来定义一个Controller,我们将该Controller映射到“/pdf”路径下。接着,我们注入了PdfFileService对象,以便在方法中调用Service层的方法。在Controller中,我们定义了一个@PostMapping注解的方法,该方法接收一个MultipartFile类型的file参数和一个Long类型的uploaderId参数,表示上传文件和上传者的编号。在方法中,我们首先获取上传文件的文件名,然后将文件保存到本地,接着创建一个PdfFile对象,并将文件名、文件路径和上传者编号设置到该对象中,最后调用PdfFileService中的insert方法将文件信息保存到数据库中。
4. Service代码
在我们的项目中,我们需要定义一个Service层来处理业务逻辑。以下是我们的Service代码:
```
@Service
public class PdfFileService {
@Autowired
private PdfFileMapper pdfFileMapper;
/**
* 保存PDF文件信息
*/
public void insert(PdfFile pdfFile) {
pdfFile.setUploadTime(new Date()); // 设置上传时间为当前时间
pdfFileMapper.insert(pdfFile); // 插入数据到数据库中
}
}
```
上述代码中,我们使用@Service注解来定义一个Service层,注入了PdfFileMapper对象。在Service层中,我们定义了一个insert方法,该方法接收一个PdfFile类型的参数,表示需要保存到数据库中的文件信息。在方法中,我们首先将上传时间设置为当前时间,然后调用PdfFileMapper中的insert方法将文件信息保存到数据库中。
5. 前端Vue和Element代码
在我们的项目中,我们需要使用Vue和Element来实现前端页面。以下是我们的Vue和Element代码:
```
<template>
<div class="pdf-upload">
<el-upload
class="upload-demo"
:auto-upload="false"
:on-change="handleChange"
:file-list="fileList"
:before-upload="beforeUpload"
:on-remove="handleRemove"
action="/pdf/upload"
>
<el-button size="small" type="primary">上传文件</el-button>
<div slot="tip" class="el-upload__tip">只能上传PDF文件,且不超过50MB</div>
</el-upload>
</div>
</template>
<script>
export default {
data() {
return {
fileList: [],
};
},
methods: {
handleChange(file) {
this.fileList.push(file.raw);
},
beforeUpload(file) {
const isPDF = file.type === 'application/pdf';
const isLt50M = file.size / 1024 / 1024 < 50;
if (!isPDF) {
this.$message.error('只能上传PDF文件!');
}
if (!isLt50M) {
this.$message.error('上传文件大小不能超过50MB!');
}
return isPDF && isLt50M;
},
handleRemove(file) {
const index = this.fileList.indexOf(file);
this.fileList.splice(index, 1);
},
},
};
</script>
```
上述代码中,我们首先定义了一个el-upload组件,并设置了auto-upload、on-change、file-list、before-upload、on-remove和action等属性。在methods中,我们定义了handleChange、beforeUpload和handleRemove三个方法,分别用于处理文件选择、文件上传前和文件删除事件。在beforeUpload方法中,我们判断上传的文件是否为PDF格式,以及文件大小是否超过50MB,如果不符合要求,我们就会弹出错误提示。最后,我们将上传的文件保存到fileList中,在文件上传事件中,我们将fileList中的文件信息发送到后台。
6. MyBatis代码和数据库建表语句
在我们的项目中,我们需要使用MyBatis来操作数据库。以下是我们的MyBatis代码和数据库建表语句:
### MyBatis代码
```
@Mapper
public interface PdfFileMapper {
/**
* 插入PDF文件信息
*/
void insert(PdfFile pdfFile);
}
```
上述代码中,我们使用@Mapper注解来定义一个PdfFileMapper接口,在接口中定义了一个insert方法,该方法用于将PdfFile对象插入到数据库中。
### 数据库建表语句
```
CREATE TABLE `pdf_file` (
`id` bigint(20) NOT NULL AUTO_INCREMENT COMMENT '主键',
`file_name` varchar(255) NOT NULL COMMENT '文件名',
`upload_time` datetime NOT NULL COMMENT '上传时间',
`file_path` varchar(255) NOT NULL COMMENT '文件路径',
`uploader_id` bigint(20) NOT NULL COMMENT '上传者编号',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COMMENT='PDF文件表';
```
上述数据库建表语句中,我们定义了一个pdf_file表,该表包括id、file_name、upload_time、file_path和uploader_id五个字段,其中id为主键。
以上就是使用Spring Boot、Vue、Element UI和MyBatis实现上传PDF文件到数据库的全部代码和配置。
用springboot,vue,mybatis和elementUI实现上传PDF文件到数据库,需要上传到数据库的内容有文件名,文件上传时间,文件路径,上传者的编号。请给我实体类代码,控制器代码,前端代码,mybatis代码和数据库建表语句
### 回答1:
很高兴为您提供所需的代码和建表语句。
首先,这是实体类代码:
```
public class PdfFile {
private Long id;
private String fileName;
private String filePath;
private Date uploadTime;
private Long uploaderId;
// getters and setters
}
```
接下来是控制器代码:
```
@RestController
@RequestMapping("/pdf")
public class PdfController {
@Autowired
private PdfService pdfService;
@PostMapping("/upload")
public ResponseEntity<?> uploadPdf(@RequestParam("file") MultipartFile file, @RequestParam("uploaderId") Long uploaderId) {
try {
PdfFile pdfFile = pdfService.savePdf(file, uploaderId);
return ResponseEntity.ok(pdfFile);
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
}
```
然后是前端代码:
```
<template>
<div>
<input type="file" @change="onFileChange">
<button @click="uploadPdf">上传</button>
</div>
</template>
<script>
export default {
data() {
return {
file: null,
uploaderId: null
};
},
methods: {
onFileChange(event) {
this.file = event.target.files[0];
},
uploadPdf() {
const formData = new FormData();
formData.append('file', this.file);
formData.append('uploaderId', this.uploaderId);
axios.post('/pdf/upload', formData)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
}
}
};
</script>
```
接下来是 MyBatis 的代码:
```
@Mapper
public interface PdfMapper {
@Insert("INSERT INTO pdf_file (file_name, file_path, upload_time, uploader_id) VALUES (#{fileName}, #{filePath}, #{uploadTime}, #{uploaderId})")
@Options(useGeneratedKeys = true, keyProperty = "id")
void insertPdf(PdfFile pdfFile);
}
```
最后是建表语句:
```
CREATE TABLE pdf_file (
id BIGINT PRIMARY KEY AUTO_INCREMENT,
file_name VARCHAR(255) NOT NULL,
file_path VARCHAR(255) NOT NULL,
upload_time DATETIME NOT NULL,
uploader_id BIGINT NOT NULL
);
```
希望这些代码能够帮助您实现上传 PDF 文件到数据库的功能。
### 回答2:
首先是实体类代码:
```java
// 文件实体类
public class FileEntity {
private String fileName;
private String uploadTime;
private String filePath;
private int uploaderId;
// 省略getter和setter方法
}
```
接下来是控制器代码(使用Spring Boot):
```java
@RestController
public class FileController {
@Autowired
private FileService fileService;
@PostMapping("/uploadFile")
public String uploadFile(@RequestParam("file") MultipartFile file, @RequestParam("uploaderId") int uploaderId) {
// 生成文件保存路径
// ...
// 保存文件到指定路径
// ...
// 构建文件实体类
FileEntity fileEntity = new FileEntity();
fileEntity.setFileName(file.getOriginalFilename());
fileEntity.setUploadTime(new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(new Date()));
fileEntity.setFilePath(filePath);
fileEntity.setUploaderId(uploaderId);
// 调用Service层保存文件实体类到数据库
fileService.saveFile(fileEntity);
return "文件上传成功";
}
}
```
再来是前端代码(使用Vue和ElementUI):
```html
<template>
<div>
<input type="file" ref="file" @change="handleFileChange">
<el-button type="primary" @click="uploadFile">上传文件</el-button>
</div>
</template>
<script>
export default {
data() {
return {
file: null
};
},
methods: {
handleFileChange(e) {
this.file = e.target.files[0];
},
uploadFile() {
let formData = new FormData();
formData.append("file", this.file);
formData.append("uploaderId", 123); // 上传者的编号
this.$http.post("/uploadFile", formData).then(response => {
console.log(response.data);
// 文件上传成功的操作
});
}
}
};
</script>
```
接下来是MyBatis的代码(使用注解方式):
```java
@Mapper
public interface FileMapper {
@Insert("INSERT INTO file (file_name, upload_time, file_path, uploader_id) VALUES (#{fileName}, #{uploadTime}, #{filePath}, #{uploaderId})")
void saveFile(FileEntity fileEntity);
}
```
最后是数据库的建表语句:
```sql
CREATE TABLE file (
id INT AUTO_INCREMENT PRIMARY KEY,
file_name VARCHAR(255) NOT NULL,
upload_time DATETIME NOT NULL,
file_path VARCHAR(255) NOT NULL,
uploader_id INT NOT NULL
);
```
### 回答3:
实体类代码:
```java
// 文件实体类
public class FileEntity {
private Integer id;
private String fileName;
private Date uploadTime;
private String filePath;
private Integer uploaderId;
// 省略getter和setter方法
}
```
控制器代码:
```java
@RestController
public class FileController {
@Autowired
private FileService fileService;
@PostMapping("/uploadFile")
public String uploadFile(MultipartFile file, Integer uploaderId) {
try {
String fileName = file.getOriginalFilename();
String filePath = "指定上传文件的保存路径";
FileEntity fileEntity = new FileEntity();
fileEntity.setFileName(fileName);
fileEntity.setUploadTime(new Date());
fileEntity.setFilePath(filePath);
fileEntity.setUploaderId(uploaderId);
fileService.uploadFile(fileEntity);
return "上传成功";
} catch (Exception e) {
e.printStackTrace();
return "上传失败";
}
}
}
```
前端代码:
```vue
<template>
<div>
<input type="file" @change="handleFileChange" />
<button @click="uploadFile">上传</button>
</div>
</template>
<script>
export default {
data() {
return {
file: null
};
},
methods: {
handleFileChange(e) {
this.file = e.target.files[0];
},
uploadFile() {
const formData = new FormData();
formData.append("file", this.file);
formData.append("uploaderId", 1); // 上传者的编号
// 使用axios或其他方式发送POST请求,将formData作为参数传递给后端接口
}
}
}
</script>
```
MyBatis代码:
```xml
<!-- Mapper接口 -->
public interface FileMapper {
void insertFile(FileEntity fileEntity);
}
<!-- Mapper.xml文件 -->
<insert id="insertFile" parameterType="com.example.entity.FileEntity">
INSERT INTO file (file_name, upload_time, file_path, uploader_id)
VALUES (#{fileName}, #{uploadTime}, #{filePath}, #{uploaderId})
</insert>
```
数据库建表语句:
```sql
CREATE TABLE `file` (
`id` INT(11) PRIMARY KEY AUTO_INCREMENT,
`file_name` VARCHAR(255) NOT NULL,
`upload_time` DATETIME NOT NULL,
`file_path` VARCHAR(255) NOT NULL,
`uploader_id` INT(11) NOT NULL
);
```
阅读全文
相关推荐
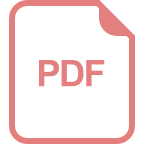
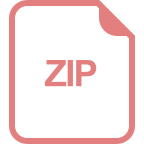
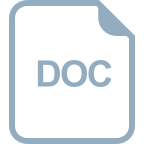
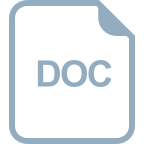
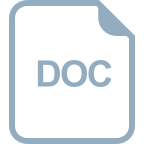
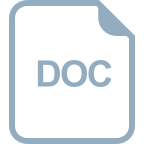
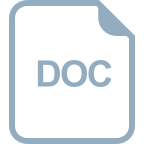
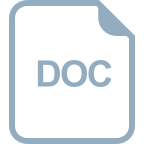
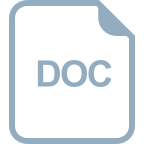
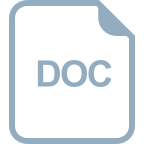
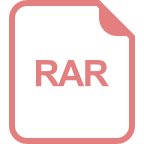
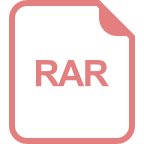
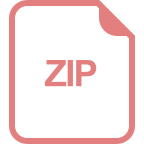
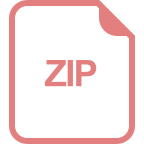