java使用摄像头录制
时间: 2023-07-11 09:29:21 浏览: 65
要在 Java 中使用摄像头录制视频,你可以使用 Java 的 OpenCV 库或者 Java Media Framework(JMF)。
使用 OpenCV 库:
1. 安装 OpenCV 库并配置环境变量。
2. 在 Java 代码中导入 OpenCV 库。
3. 初始化摄像头并打开视频流。
4. 读取视频流中的每一帧,并将其显示在窗口中或者保存为视频文件。
以下是示例代码:
```java
import org.opencv.core.*;
import org.opencv.videoio.VideoCapture;
public class VideoRecorder {
public static void main(String[] args) {
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
VideoCapture camera = new VideoCapture(0);
if (!camera.isOpened()) {
System.out.println("Failed to open camera!");
return;
}
Mat frame = new Mat();
Size frameSize = new Size((int) camera.get(Videoio.CAP_PROP_FRAME_WIDTH), (int) camera.get(Videoio.CAP_PROP_FRAME_HEIGHT));
VideoWriter videoWriter = new VideoWriter("output.avi", VideoWriter.fourcc('M','J','P','G'), 30, frameSize, true);
while (camera.read(frame)) {
// Display the frame
HighGui.imshow("Camera", frame);
// Save the frame to video file
videoWriter.write(frame);
if (HighGui.waitKey(1) == 27) {
break;
}
}
camera.release();
videoWriter.release();
HighGui.destroyAllWindows();
}
}
```
使用 JMF:
1. 下载并安装 JMF。
2. 在 Java 代码中导入 JMF 库。
3. 创建一个 Player 对象并指定视频源。
4. 创建一个 DataSource 对象并将其连接到 Player 对象。
5. 创建一个 Processor 对象并连接到 DataSource 对象。
6. 将 Processor 对象的输出连接到一个 FileWriter 对象,用于保存视频文件。
7. 开始录制并停止录制时关闭所有对象。
以下是示例代码:
```java
import java.io.File;
import java.io.IOException;
import javax.media.*;
import javax.media.format.VideoFormat;
import javax.media.protocol.DataSource;
import javax.media.protocol.FileTypeDescriptor;
public class VideoRecorder {
public static void main(String[] args) {
String outputFile = "output.avi";
int width = 640;
int height = 480;
int frameRate = 30;
File file = new File(outputFile);
try {
file.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
// Create a player for the video source
Player player = null;
try {
MediaLocator locator = new MediaLocator("vfw://0");
player = Manager.createRealizedPlayer(locator);
} catch (Exception e) {
e.printStackTrace();
}
// Create a data source for the player
DataSource dataSource = player.getDataSource();
// Create a processor for the data source
Processor processor = null;
try {
processor = Manager.createProcessor(dataSource);
} catch (Exception e) {
e.printStackTrace();
}
// Configure the processor
processor.configure();
while (processor.getState() < Processor.Configured) {
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Set the output format
TrackControl trackControl = (TrackControl) processor.getTrackControls()[0];
VideoFormat videoFormat = new VideoFormat(VideoFormat.MJPG, new Dimension(width, height), Format.NOT_SPECIFIED, Format.byteArray, (float) frameRate);
trackControl.setFormat(videoFormat);
// Create a file writer for the processor
FileTypeDescriptor outputFileType = new FileTypeDescriptor(FileTypeDescriptor.QUICKTIME);
outputFileType.appendSuffix("avi");
DataSource outputDataSource = null;
try {
outputDataSource = Manager.createDataSource(new MediaLocator("file:" + outputFile));
} catch (Exception e) {
e.printStackTrace();
}
DataSink dataSink = null;
try {
dataSink = Manager.createDataSink(processor.getDataOutput(), outputDataSource);
dataSink.open();
} catch (Exception e) {
e.printStackTrace();
}
// Connect the processor to the file writer
processor.setContentDescriptor(new ContentDescriptor(outputFileType));
processor.realize();
while (processor.getState() < Processor.Realized) {
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
processor.start();
dataSink.start();
System.out.println("Recording...");
// Wait for the user to stop recording
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
// Stop recording and close all objects
System.out.println("Stopping recording...");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
dataSink.stop();
dataSink.close();
processor.stop();
processor.close();
player.stop();
player.close();
System.out.println("Done.");
}
}
```
相关推荐
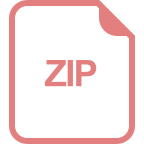














