qt 如何实现多线程的消息队列
时间: 2023-03-21 08:02:58 浏览: 773
在 Qt 中,可以使用 QThread 类来实现多线程应用程序。要在多个线程之间传递消息,可以使用 Qt 的信号和槽机制。可以使用信号将消息发送到主线程的消息队列中,并使用槽来处理这些消息。
以下是实现多线程消息队列的一般步骤:
1. 创建一个继承自 QThread 的子类。
```cpp
class WorkerThread : public QThread {
Q_OBJECT
public:
explicit WorkerThread(QObject *parent = nullptr);
void run() override;
signals:
void message(const QString &text);
};
```
2. 实现子类的 run() 函数,该函数将在新线程中运行。在 run() 函数中,可以使用信号来发送消息到主线程的消息队列中。
```cpp
void WorkerThread::run() {
// 在这里执行一些耗时操作
// 发送消息到主线程的消息队列中
emit message("耗时操作完成!");
}
```
3. 在主线程中创建 WorkerThread 实例,并连接其 message() 信号到槽函数。
```cpp
class MainWindow : public QMainWindow {
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr);
public slots:
void showMessage(const QString &text);
private:
Ui::MainWindow *ui;
WorkerThread *workerThread;
};
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow) {
ui->setupUi(this);
workerThread = new WorkerThread(this);
connect(workerThread, &WorkerThread::message,
this, &MainWindow::showMessage);
}
void MainWindow::showMessage(const QString &text) {
ui->statusBar->showMessage(text);
}
```
4. 启动 WorkerThread 线程。
```cpp
void MainWindow::on_startButton_clicked() {
workerThread->start();
}
```
当 WorkerThread 完成耗时操作时,将在主线程的消息队列中调用 showMessage() 槽函数,从而在应用程序的状态栏中显示消息。
相关推荐
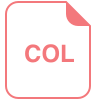
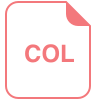
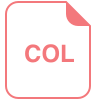
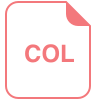
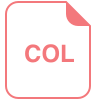









