C++ 时间戳转string 时间格式yyyymmddhhMMss
时间: 2023-08-04 11:35:48 浏览: 115
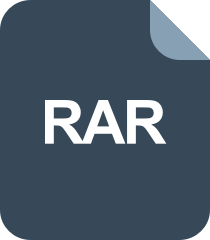
javascript时间戳与时间格式自由转换,可从时间到时间戳,也可从时间戳到任意指定时间格式
以下是C++中将时间戳转换为格式为`yyyymmddhhMMss`的字符串的示例代码:
```c++
#include <iostream>
#include <iomanip>
#include <sstream>
#include <ctime>
std::string timestampToString(long timestamp) {
std::time_t t = timestamp;
std::tm tm = *std::localtime(&t);
std::ostringstream oss;
oss << std::put_time(&tm, "%Y%m%d%H%M%S");
return oss.str();
}
int main() {
long timestamp = 1609459200; // 时间戳
std::string stringTime = timestampToString(timestamp);
std::cout << stringTime << std::endl; // 输出:20210101000000
return 0;
}
```
该示例中使用了`std::localtime`将时间戳转换为`tm`结构体,然后使用`std::put_time`将`tm`结构体格式化为`yyyymmddhhMMss`格式的字符串。最后使用`std::ostringstream`将格式化后的字符串转换为`std::string`类型。
阅读全文
相关推荐
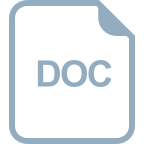
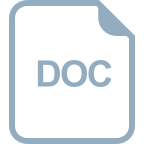


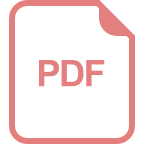
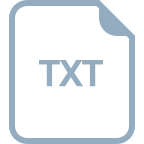
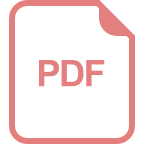
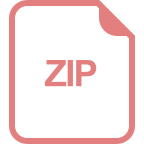
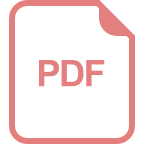
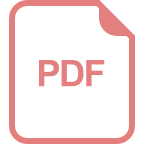
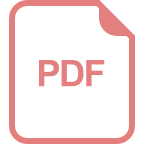




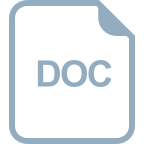