请问如何得到在一个字符串中某一个字符串的数量c++
时间: 2023-06-02 18:06:07 浏览: 104
你可以使用字符串的 count() 方法来得到某个字符串在另一个字符串中出现的次数,例如:
```
s = 'hello world, hello universe'
count = s.count('hello')
print(count) # 输出 2
```
以上代码中,第一行定义了一个字符串 s,第二行使用 count() 方法得到字符串 'hello' 在 s 中出现的次数,第三行将结果输出。
阅读全文
相关推荐
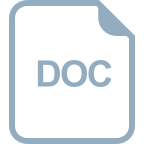
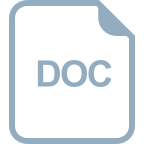
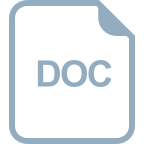
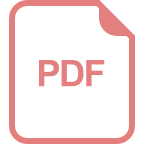
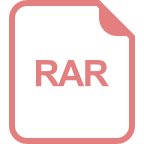
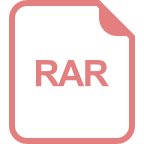
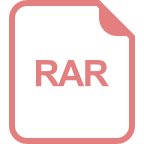
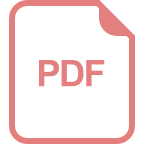
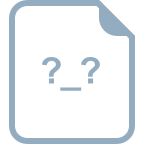
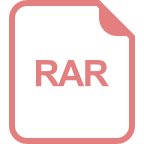
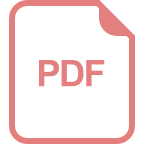
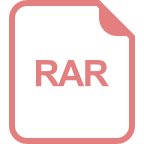