ArcGIS enginee C#编写代码在axMapControl中打开一个要素类文件,生成一些不在该要素类范围内的均匀分布的随机点,并绘制在axMapControl上
时间: 2024-03-23 10:42:53 浏览: 243
以下是在 `axMapControl` 控件中打开要素类文件,生成随机点并绘制的 C# 代码示例:
```csharp
// Instantiate a new MapControl object
AxMapControl mapControl = new AxMapControl();
// Set the workspace path where the feature class is located
string workspacePath = @"C:\Data\MyGeodatabase.gdb";
// Set the name of the feature class to open
string featureClassName = "MyFeatureClass";
// Open the feature class
IFeatureClass featureClass = OpenFeatureClass(workspacePath, featureClassName);
// Generate random points
IPoint[] randomPoints = GenerateRandomPoints(featureClass, 100);
// Add the random points to a new feature class
IFeatureClass randomPointsFeatureClass = AddRandomPointsToFeatureClass(workspacePath, "RandomPoints", randomPoints);
// Add the random points feature class to the map
AddFeatureClassToMap(mapControl, randomPointsFeatureClass);
// Refresh the map control
mapControl.Refresh();
```
在上面的代码中,`OpenFeatureClass` 方法会打开指定的要素类,`GenerateRandomPoints` 方法会生成指定数量的随机点,`AddRandomPointsToFeatureClass` 方法会将随机点添加到一个新的要素类中,`AddFeatureClassToMap` 方法会将新的要素类添加到 `axMapControl` 控件中。在执行完所有操作之后,最后通过调用 `Refresh` 方法来刷新 `axMapControl` 控件。
下面是 `OpenFeatureClass` 方法的代码示例:
```csharp
public static IFeatureClass OpenFeatureClass(string workspacePath, string featureClassName)
{
// Instantiate a new workspace factory
IWorkspaceFactory workspaceFactory = new FileGDBWorkspaceFactory();
// Open the workspace
IWorkspace workspace = workspaceFactory.OpenFromFile(workspacePath, 0);
// Open the feature class
IFeatureWorkspace featureWorkspace = workspace as IFeatureWorkspace;
IFeatureClass featureClass = featureWorkspace.OpenFeatureClass(featureClassName);
return featureClass;
}
```
下面是 `GenerateRandomPoints` 方法的代码示例:
```csharp
public static IPoint[] GenerateRandomPoints(IFeatureClass featureClass, int numPoints)
{
// Get the extent of the feature class
IEnvelope envelope = featureClass.Extent;
// Generate random points within the extent
IRandom random = new RandomNumberGeneratorClass();
IPoint[] randomPoints = new IPoint[numPoints];
for (int i = 0; i < numPoints; i++)
{
double x = envelope.XMin + (envelope.XMax - envelope.XMin) * random.NextDouble();
double y = envelope.YMin + (envelope.YMax - envelope.YMin) * random.NextDouble();
IPoint point = new PointClass();
point.PutCoords(x, y);
randomPoints[i] = point;
}
return randomPoints;
}
```
下面是 `AddRandomPointsToFeatureClass` 方法的代码示例:
```csharp
public static IFeatureClass AddRandomPointsToFeatureClass(string workspacePath, string featureClassName, IPoint[] points)
{
// Instantiate a new workspace factory
IWorkspaceFactory workspaceFactory = new FileGDBWorkspaceFactory();
// Open the workspace
IWorkspace workspace = workspaceFactory.OpenFromFile(workspacePath, 0);
// Create a new feature class
IFeatureWorkspace featureWorkspace = workspace as IFeatureWorkspace;
IFields fields = new FieldsClass();
IFieldsEdit fieldsEdit = fields as IFieldsEdit;
IField objectIDField = new FieldClass();
IFieldEdit objectIDFieldEdit = objectIDField as IFieldEdit;
objectIDFieldEdit.Name_2 = "OBJECTID";
objectIDFieldEdit.Type_2 = esriFieldType.esriFieldTypeOID;
fieldsEdit.AddField(objectIDField);
IField shapeField = new FieldClass();
IFieldEdit shapeFieldEdit = shapeField as IFieldEdit;
shapeFieldEdit.Name_2 = "SHAPE";
shapeFieldEdit.Type_2 = esriFieldType.esriFieldTypeGeometry;
IGeometryDef geometryDef = new GeometryDefClass();
IGeometryDefEdit geometryDefEdit = geometryDef as IGeometryDefEdit;
geometryDefEdit.GeometryType_2 = esriGeometryType.esriGeometryPoint;
geometryDefEdit.SpatialReference_2 = ((IGeoDataset)featureClass).SpatialReference;
shapeFieldEdit.GeometryDef_2 = geometryDef;
fieldsEdit.AddField(shapeField);
IFeatureClass featureClass = featureWorkspace.CreateFeatureClass(featureClassName, fields, null, null, esriFeatureType.esriFTSimple, "SHAPE", "");
// Add the random points to the feature class
IFeatureBuffer featureBuffer = featureClass.CreateFeatureBuffer();
IFeatureCursor featureCursor = featureClass.Insert(true);
for (int i = 0; i < points.Length; i++)
{
featureBuffer.Shape = points[i];
featureCursor.InsertFeature(featureBuffer);
}
featureCursor.Flush();
return featureClass;
}
```
下面是 `AddFeatureClassToMap` 方法的代码示例:
```csharp
public static void AddFeatureClassToMap(AxMapControl mapControl, IFeatureClass featureClass)
{
// Create a new feature layer
IFeatureLayer featureLayer = new FeatureLayerClass();
featureLayer.FeatureClass = featureClass;
featureLayer.Name = featureClass.AliasName;
// Add the feature layer to the map
mapControl.Map.AddLayer(featureLayer);
}
```
请注意,以上代码仅供参考,你可能需要根据自己的需求进行修改和调整。
阅读全文
相关推荐





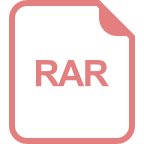

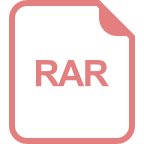




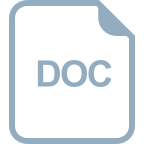
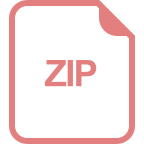
