java压缩zip文件夹错误_Java将文件或者文件夹压缩成zip(修复文件夹中存在多个文件报Stream Closed错误问题)...
时间: 2024-03-11 11:50:49 浏览: 28
你可以使用Java中的ZipOutputStream类来压缩文件或文件夹,示例代码如下:
```java
import java.io.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class ZipUtil {
private static final int BUFFER_SIZE = 2 * 1024;
public static void zip(String srcDir, String zipFilePath) throws Exception {
FileOutputStream fos = null;
ZipOutputStream zos = null;
try {
File srcFile = new File(srcDir);
fos = new FileOutputStream(zipFilePath);
zos = new ZipOutputStream(fos);
compress(srcFile, zos, srcFile.getName());
} catch (Exception e) {
throw e;
} finally {
if (zos != null) {
zos.close();
}
if (fos != null) {
fos.close();
}
}
}
private static void compress(File sourceFile, ZipOutputStream zos, String name) throws Exception {
byte[] buf = new byte[BUFFER_SIZE];
if (sourceFile.isFile()) {
zos.putNextEntry(new ZipEntry(name));
int len;
FileInputStream in = new FileInputStream(sourceFile);
while ((len = in.read(buf)) != -1) {
zos.write(buf, 0, len);
}
zos.closeEntry();
in.close();
} else {
File[] listFiles = sourceFile.listFiles();
if (listFiles == null || listFiles.length == 0) {
zos.putNextEntry(new ZipEntry(name + File.separator));
zos.closeEntry();
} else {
for (File file : listFiles) {
compress(file, zos, name + File.separator + file.getName());
}
}
}
}
}
```
注意事项:
- BUFFER_SIZE 变量指定了压缩时使用的缓存大小,可以根据需要进行修改。
- 压缩时需要使用到 FileOutputStream 和 ZipOutputStream,要注意及时关闭这两个流。
- 压缩时需要递归处理文件夹中的文件,ZipEntry 对象的名称需要带上文件夹的路径。
相关推荐
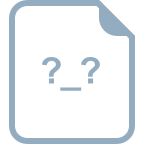














