请用java使用winrm技术完成在远程服务器上传文件,下载文件和执行命令功能
时间: 2024-05-07 12:19:12 浏览: 178
在Java中使用WinRM技术需要使用WinRM4j库,下面是一个简单的示例代码:
```java
import com.github.kubesys.winrm.WinRMFactory;
import com.github.kubesys.winrm.WinRmTool;
import com.github.kubesys.winrm.exception.WinRMException;
import com.github.kubesys.winrm.ssl.TrustAllCerts;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.URI;
import java.nio.charset.StandardCharsets;
public class WinRMExample {
public static void main(String[] args) throws IOException, WinRMException {
String hostname = "remote-server.example.com";
String username = "remote-user";
String password = "remote-password";
String endpoint = String.format("https://%s:5986/wsman", hostname);
WinRmTool.Builder builder = WinRMFactory.builder(endpoint, username, password);
builder.setAuthenticationScheme(WinRmTool.AuthenticationScheme.NTLM);
builder.setHttpsNoVerification();
builder.setListener(new WinRmTool.Listener() {
@Override
public void onStdout(String message) {
System.out.println("STDOUT: " + message);
}
@Override
public void onStderr(String message) {
System.err.println("STDERR: " + message);
}
});
WinRmTool tool = builder.build();
tool.executeCommand("ipconfig");
// 上传文件
String remoteFile = "C:\\test\\file.txt";
String localFile = "/path/to/local/file.txt";
byte[] content = "Hello, World!".getBytes(StandardCharsets.UTF_8);
try (FileOutputStream out = new FileOutputStream(new File(localFile))) {
out.write(content);
}
tool.copyToRemote(localFile, remoteFile);
// 下载文件
byte[] remoteContent = tool.copyFromRemote(remoteFile);
String downloadedFile = "/path/to/downloaded/file.txt";
try (FileOutputStream out = new FileOutputStream(new File(downloadedFile))) {
out.write(remoteContent);
}
}
}
```
在上面的示例代码中,我们使用WinRMFactory创建WinRmTool对象,并设置了远程服务器的连接参数、身份验证方式、HTTPS证书验证方式和监听器。然后我们调用executeCommand方法执行命令,并使用copyToRemote和copyFromRemote方法上传和下载文件。需要注意的是,如果远程服务器没有安装WinRM服务,需要先安装WinRM服务并启用WinRM服务。
阅读全文
相关推荐
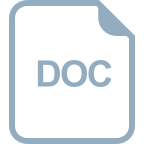
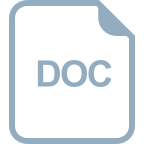
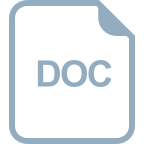
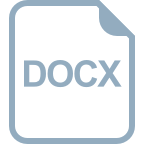
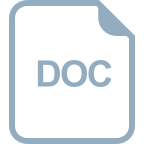
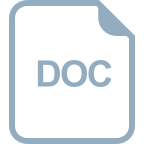
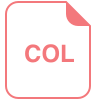
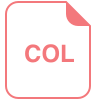
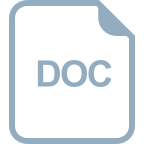
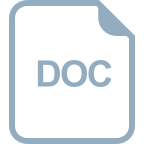